Label encoding across multiple columns in scikit-learn
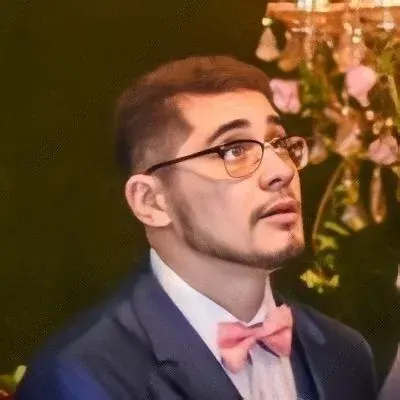
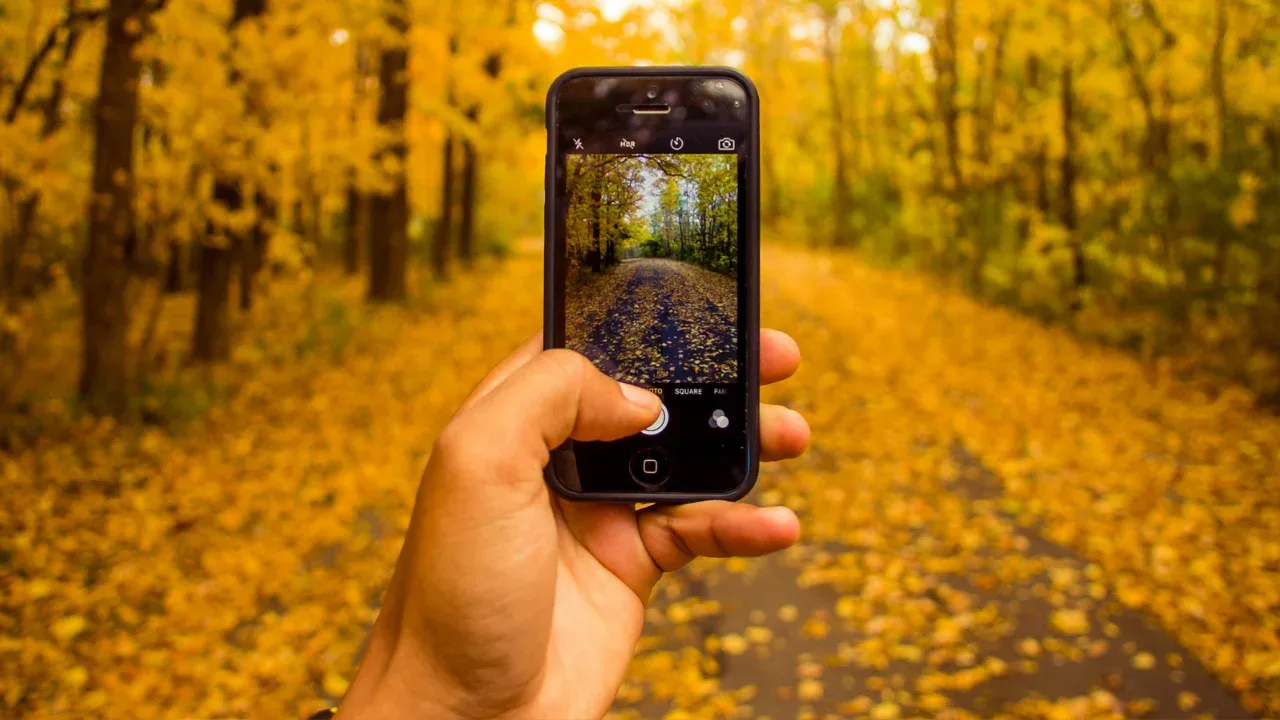
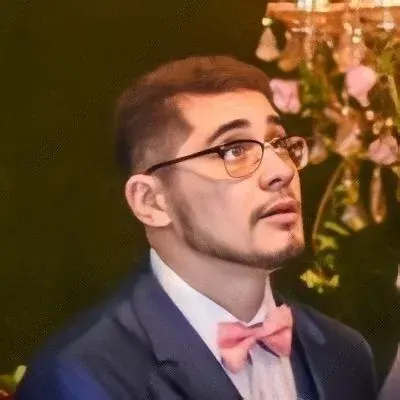
📝 Label Encoding Across Multiple Columns in scikit-learn
Are you working with a pandas DataFrame that has multiple columns of string labels and want to encode them using scikit-learn's LabelEncoder? You've come to the right place! In this blog post, we'll discuss a common issue when using LabelEncoder across multiple columns and provide an easy solution to help you avoid creating a LabelEncoder object for each column.
The Problem
Let's start by setting the context. You have a pandas DataFrame with many columns (let's say 50+) and you want to encode the string labels in each column using a single LabelEncoder object. This approach ensures consistency in encoding across all columns and simplifies your code. However, when you try to fit the entire DataFrame into LabelEncoder, you encounter the following error:
import pandas
from sklearn import preprocessing
df = pandas.DataFrame({
'pets': ['cat', 'dog', 'cat', 'monkey', 'dog', 'dog'],
'owner': ['Champ', 'Ron', 'Brick', 'Champ', 'Veronica', 'Ron'],
'location': ['San_Diego', 'New_York', 'New_York', 'San_Diego', 'San_Diego', 'New_York']
})
le = preprocessing.LabelEncoder()
le.fit(df)
Traceback (most recent call last):
File "", line 1, in
File "/Users/bbalin/anaconda/lib/python2.7/site-packages/sklearn/preprocessing/label.py", line 103, in fit
y = column_or_1d(y, warn=True)
File "/Users/bbalin/anaconda/lib/python2.7/site-packages/sklearn/utils/validation.py", line 306, in column_or_1d
raise ValueError("bad input shape {0}".format(shape))
ValueError: bad input shape (6, 3)
The Solution
The error message suggests that the input shape is not compatible with LabelEncoder. To overcome this problem, we need to iterate over each column and apply the LabelEncoder individually. Here's the solution:
import pandas as pd
from sklearn import preprocessing
df = pd.DataFrame({
'pets': ['cat', 'dog', 'cat', 'monkey', 'dog', 'dog'],
'owner': ['Champ', 'Ron', 'Brick', 'Champ', 'Veronica', 'Ron'],
'location': ['San_Diego', 'New_York', 'New_York', 'San_Diego', 'San_Diego', 'New_York']
})
le = preprocessing.LabelEncoder()
for column in df.columns:
df[column] = le.fit_transform(df[column])
By iterating over each column in the DataFrame and applying LabelEncoder to each column individually, we avoid the error and successfully encode all the string labels.
Going Beyond
If you want to take your label encoding code a step further, you can consider creating a utility function that encapsulates the iteration process. This utility function can handle any DataFrame with string label columns and automatically apply the label encoding. Here's an example of how the utility function can look like:
def label_encode_dataframe(df):
le = preprocessing.LabelEncoder()
for column in df.columns:
df[column] = le.fit_transform(df[column])
# Usage
label_encode_dataframe(df)
Conclusion
Label encoding across multiple columns in scikit-learn's LabelEncoder can be a bit tricky when encountering the "bad input shape" error. However, by iterating over each column individually, we can easily encode the string labels without any issues. Furthermore, considering the creation of a utility function simplifies the process even more.
Now, it's your turn! Give it a try and enhance your data preprocessing skills by using the tips and code snippets provided in this blog post. Don't hesitate to share your experience and any other solutions you come up with.
Happy label encoding! ✨🔠
📞 Call-to-Action
Did you find this blog post helpful? Do you have any other data preprocessing questions or challenges? Leave a comment below and let's start a conversation! Don't forget to share this post with your fellow data enthusiasts and help them overcome the labeling encoding hurdle.