Iterating over dictionaries using "for" loops
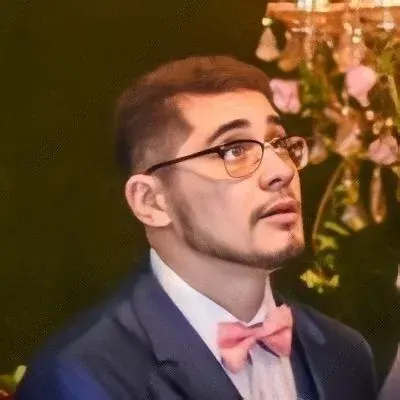
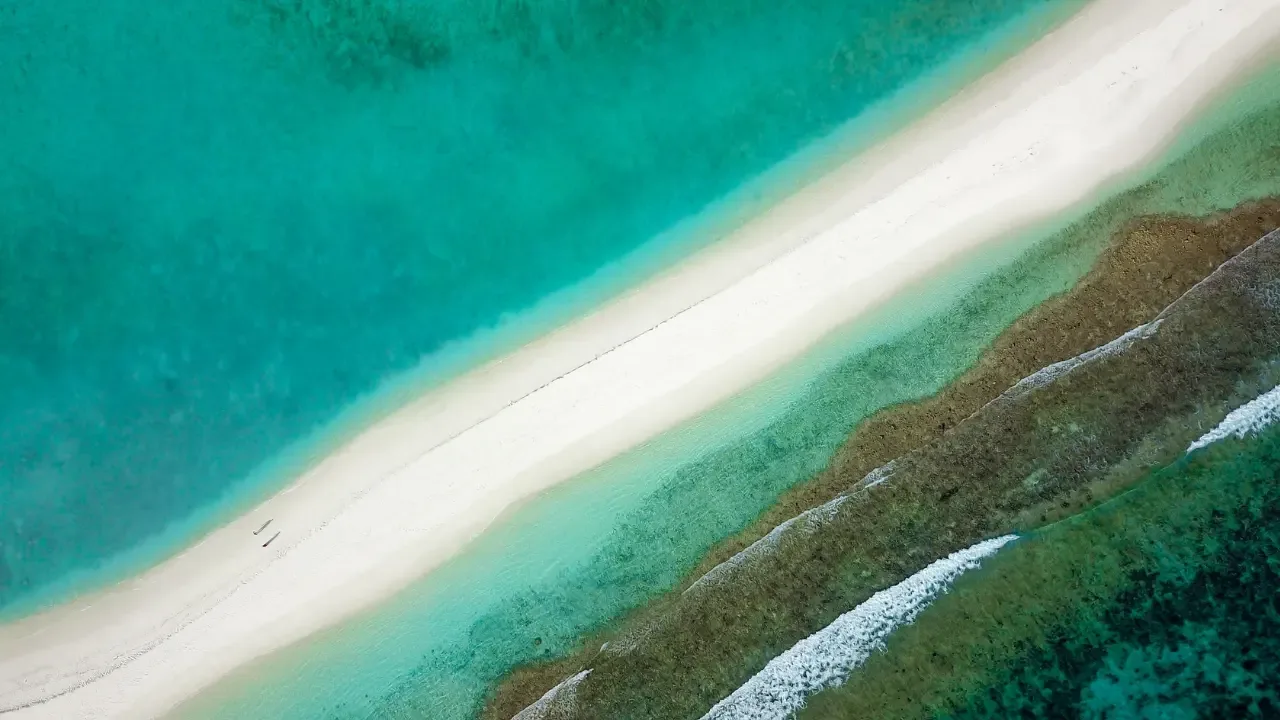
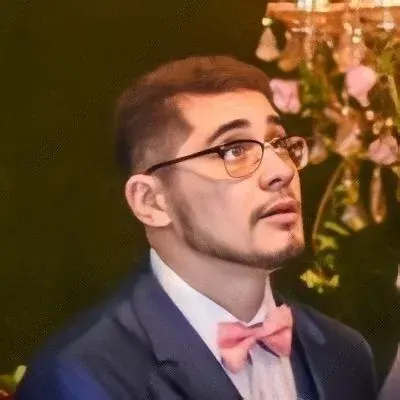
📚 The Ultimate Guide to Iterating Over Dictionaries in Python Using 'for' Loops
Are you a Python enthusiast who wants to master the art of iterating over dictionaries using 'for' loops? 🐍 No worries! We've got you covered. In this comprehensive guide, we'll address common issues, provide easy solutions, and empower you to become a dictionary-dwelling ninja! 💪
But before we dive into the world of dictionaries and loops, let's take a step back and understand the context that sparked this question:
d = {'x': 1, 'y': 2, 'z': 3}
for key in d:
print(key, 'corresponds to', d[key])
🤔 Understanding the Magic: How Does Python Know What to Do?
Python is an intelligent language, and it recognizes that when you iterate over a dictionary using a 'for' loop, it should traverse the keys of the dictionary. 🎩
So, what about the key
itself? Is it some sort of magical keyword, or just a regular variable? 🧐
Well, the good news is that key
is just a plain old variable. You could name it whatever you want! The convention is to use key
to make your code more readable, but you can go wild and call it anything you like. How about pineapple
or banana
? 🍍 🍌
💡 A Solution Straight Out of the 📚
Now that the mystery is solved, let's get down to business. Here's the way to iterate over dictionaries in Python:
for key in dictionary:
# Do something with `key` or `dictionary[key]`
Pretty simple, right? 🎉
🚀 Overcoming Common Challenges: Accessing Values
When iterating over dictionaries, it's common to want both the key and the corresponding value. 🗝️ To access the value, you can use the dictionary[key]
syntax we saw in the original example.
But what if you only want the values and don't care about the keys? Well, Python's got a trick up its sleeve! You can use the .values()
method to directly access the values in the dictionary:
for value in dictionary.values():
# Do something with the `value`
No need to worry about the keys here, Python will handle that for you! 🎩✨
🤝 Joining Forces: Getting Both Keys and Values
Now, suppose you're feeling greedy and want both the keys and values as you iterate over the dictionary. Python's got you covered here as well! Just use the .items()
method to gain access to both:
for key, value in dictionary.items():
# Do something with both `key` and `value`
With this technique, you'll be a dictionary master in no time! 🥷
💬 We Need Your Pythonic Superpowers!
That's it, fellow Pythonistas! You've learned the art of iterating over dictionaries using 'for' loops, and you're now ready to conquer the programming world! 🌍💻
But before you go, we'd love to hear from you! Share your favorite use case for iterating over dictionaries in the comments below, and let's inspire each other with our Pythonic superpowers! 💪✨
Happy coding, and may the Python gods be ever in your favor! 🐍❤️