Iterate over model instance field names and values in template
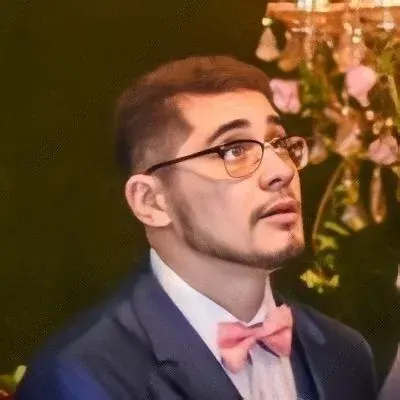
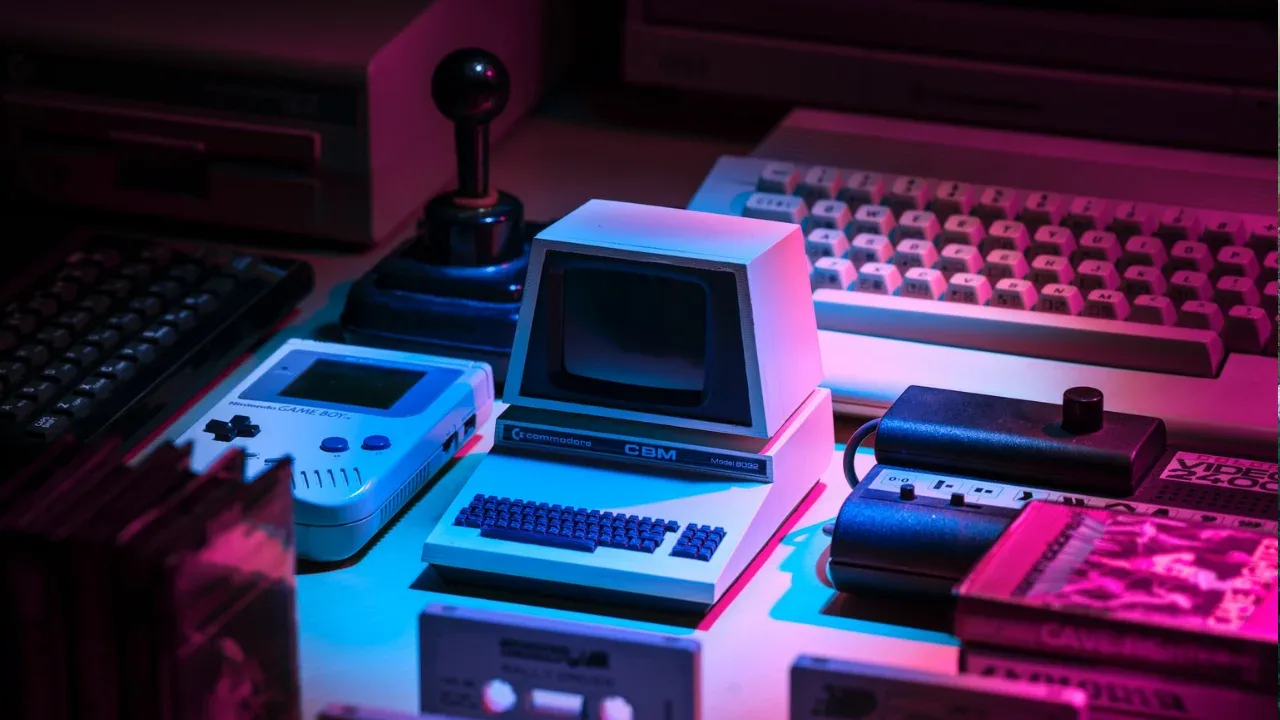
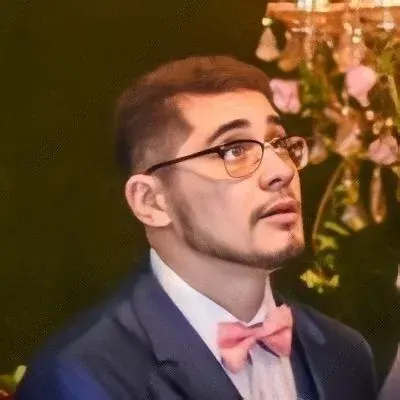
Iterating Over Model Instance Field Names and Values in a Django Template
Are you trying to display the field names and corresponding values of a model instance in a tabular format in your Django template? Look no further! In this blog post, we will explore a neat and "Django-approved" way to achieve this commonly required task.
The Problem
Let's say you have a model called Client
with fields name
and email
. You want to display the field names and their corresponding values of a model instance in a tabular format like this:
Field Name Field Value
---------- -----------
Name Wayne Koorts
E-mail waynes@email.com
The challenge is to dynamically iterate over the field names and values in your Django template without hardcoding them.
The Solution
Django provides a handy method called model._meta.fields
that returns a list of all the fields of a model instance in the order they were declared. We can leverage this method to iterate over the fields dynamically in our template.
Here's a step-by-step guide to implementing the solution:
Pass the instance of the model to your template from the view. Let's assume you pass the instance as a variable called
client_instance
.# views.py def client_detail(request, client_id): client_instance = Client.objects.get(id=client_id) return render(request, 'client_detail.html', {'client_instance': client_instance})
In your template, loop over the
client_instance._meta.fields
using the{% for %}
template tag.<!-- client_detail.html --> <table> {% for field in client_instance._meta.fields %} <tr> <td>{{ field.verbose_name }}</td> <td>{{ field.value_from_object(client_instance) }}</td> </tr> {% endfor %} </table>
In the above code,
field.verbose_name
retrieves the verbose name of the field, andfield.value_from_object(client_instance)
fetches the value of that particular field from theclient_instance
.
That's it! With these simple steps, you can dynamically iterate over the field names and values of a model instance in a Django template.
Why This Approach Works
Django's _meta
attribute contains metadata about the model, including information about its fields. By accessing model._meta.fields
, we can obtain a list of all the fields in the model instance, allowing us to iterate over them in the template.
By using field.verbose_name
, we ensure that the displayed field names match the verbose_name
specified in the model (if provided).
The field.value_from_object()
method provides a convenient way to retrieve the value of a specific field from a model instance, without having to know the field's name in advance.
Conclusion
Displaying the field names and values of a model instance in a tabular format is a common task in Django development. With the solution we explored in this blog post, you can easily achieve this without hardcoding the fields in your template.
So go ahead, implement this solution in your Django projects, and create stunning template displays of your model instances!
If you found this blog post helpful, don't forget to share it with your fellow Django developers and leave a comment below sharing your thoughts and experiences.
Happy coding! 😊🐍