Is there a way to auto-adjust Excel column widths with pandas.ExcelWriter?
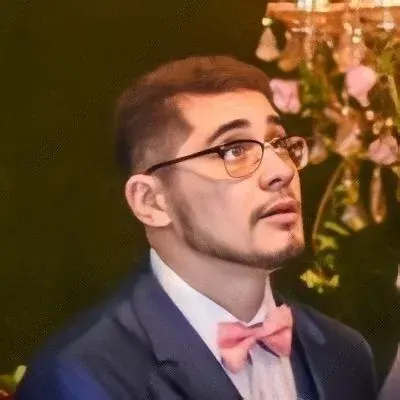
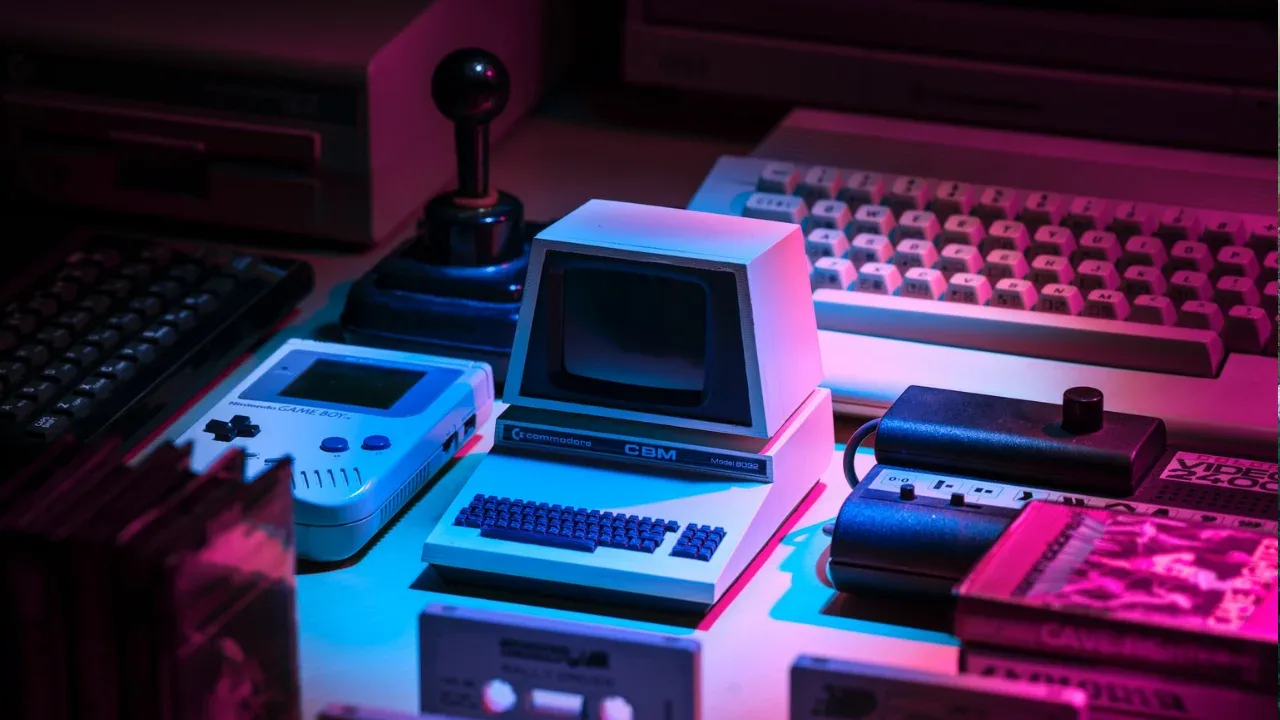
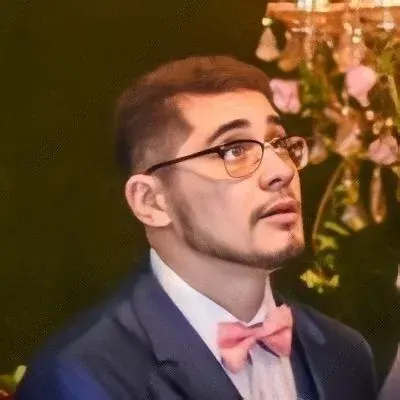
Is there a way to auto-adjust Excel column widths with pandas.ExcelWriter?
So, you're using pandas and the pandas.ExcelWriter
method to generate some awesome Excel reports. 📊📈 But there's just one little problem: the column widths are fixed, and that's not cool. 😓
Fear not, my friend! I'm here to help you find the solution and make those columns auto-adjust to the data. 🙌
First, let's take a look at the code you have so far. You're using the df.to_excel()
method to write your dataframe to an Excel file using the pandas.ExcelWriter
engine. Here's a snippet of your code:
writer = pd.ExcelWriter(excel_file_path, engine='openpyxl')
df.to_excel(writer, sheet_name="Summary")
Now, let's dive into the options available to adjust the column widths. You were right in noticing that the pandas documentation doesn't provide an explicit option for setting column widths. But worry not, we have a sneaky trick up our sleeves! 😉
You can use OpenPyXL, the library you're already using, to adjust the column widths after the data has been written to the Excel file. Here's how you can do it:
# After writing the dataframe to Excel
writer.save()
# Open the workbook with openpyxl
workbook = openpyxl.load_workbook(excel_file_path)
# Select the sheet you want to adjust the column widths for
sheet = workbook["Summary"]
# Loop over the columns and set the width based on the maximum content length in each column
for column in sheet.columns:
max_length = 0
column = [(cell.coordinate, len(str(cell.value))) for cell in column]
for cell in column:
if cell[1] > max_length:
max_length = cell[1]
adjusted_width = (max_length + 2)
sheet.column_dimensions[column[0][0].split(':')[0]].width = adjusted_width
# Save the workbook with the adjusted column widths
workbook.save(excel_file_path)
And there you have it! 🎉 After writing the data to the Excel file, you can use OpenPyXL to loop over the columns, find the maximum content length in each column, and adjust the column widths accordingly.
Remember to adjust the excel_file_path
variable to the path where you want to save your Excel file.
Now, go ahead and try it out! Your columns will adjust to the data, and your Excel reports will be even more awesome. 😎
If you have any questions or face any problems, feel free to leave a comment below. Let's rock those column widths together! 💪
Did you find this guide helpful? Share your experience or any other tricks you've discovered in the comments below! Let's geek out over Excel together. 😄