Is there a simple way to remove multiple spaces in a string?
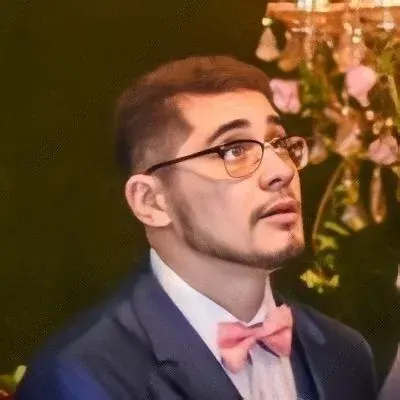
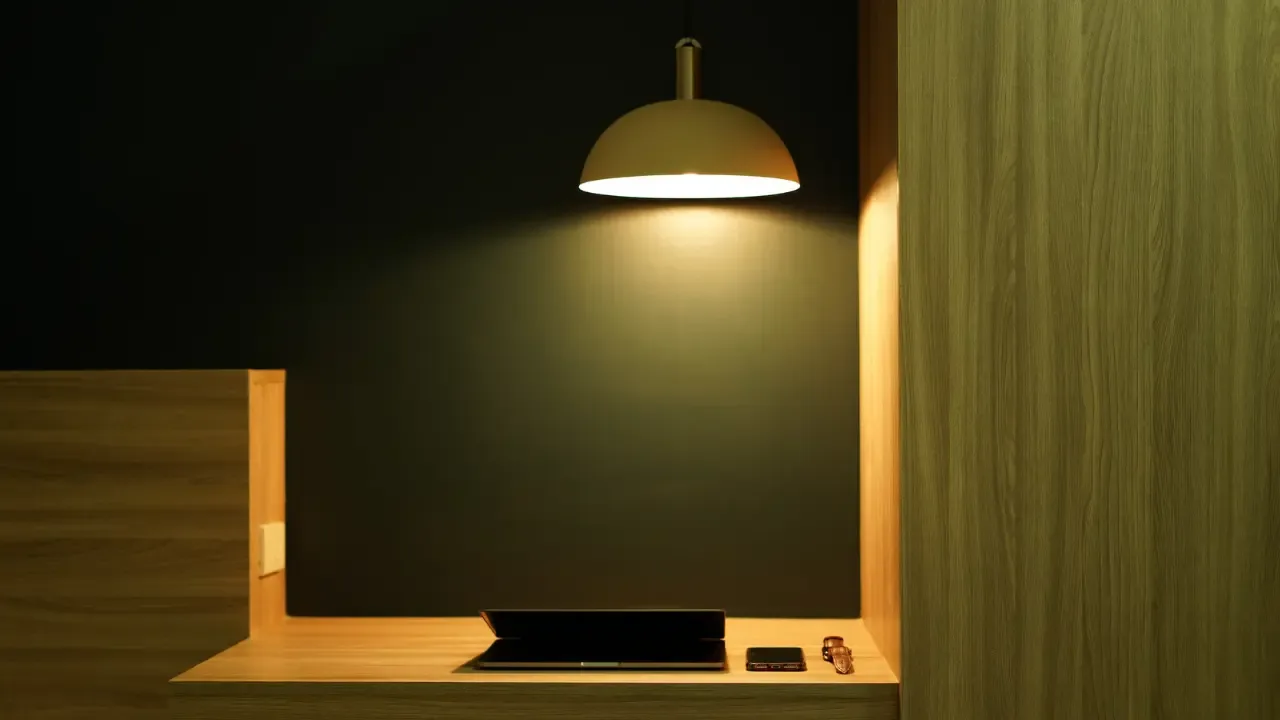
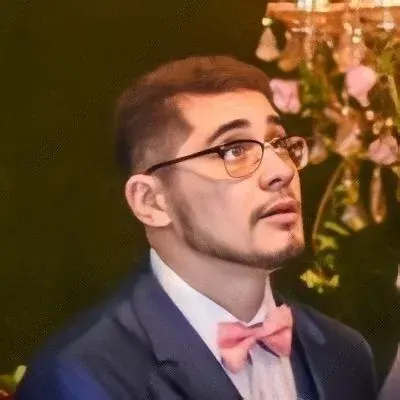
Removing Multiple Spaces in a String: The Simple Solution π₯
Have you ever encountered a string that is littered with unnecessary multiple spaces? It can be quite frustrating to deal with! Whether it's a user input or data from an external source, having extra spaces can negatively impact the readability and functionality of your code. π«
But fear not! We're here to save the day with a simple solution that doesn't involve complicated string splitting or messy list manipulations. π¦ΈββοΈβ¨
The Problem: Too Many Spaces in a String
Let's take a look at an example string to illustrate the issue:
"The fox jumped over the log."
Notice those pesky extra spaces between words? We want to transform this string into a clean, single-spaced version:
"The fox jumped over the log."
The Simple Solution: One Line, No Fuss! π
To achieve our goal of removing multiple spaces in a string, Python provides a convenient method called join()
that can swiftly solve this problem. Here's how you can use it in just one line of code:
import re
clean_string = re.sub(' +', ' ', your_string)
Let's break down what's happening here:
First, we import the regular expression (regex) module
re
. In order to usere.sub()
, we need to have this module available.Next, we call the
re.sub()
method with three arguments:The first argument,
' +'
, is a regex pattern that matches one or more spaces in the string.The second argument,
' '
, is the replacement for each match β in this case, a single space.Finally, the third argument,
your_string
, is the string that we want to process and clean up.
The
re.sub()
method replaces all occurrences of one or more consecutive spaces with a single space, resulting in a clean and readable string.
That's it! π In just one line of code, all those extra spaces are neatly removed, leaving behind a beautifully formatted string.
Why Use This Solution?
Simplicity: The
re.sub()
method makes removing multiple spaces from a string a breeze. No need for elaborate loops or complex logic. One line is all it takes!Efficiency: The solution efficiently replaces all multiple spaces in one go, ensuring optimal performance even with large strings.
Maintainability: By using a common method like
re.sub()
, your code remains clean and easy to understand for other developers who may read or maintain it.
Your Turn! π
Now that you have the superpower to clean up strings with ease, go ahead and try it out in your own Python projects! Let us know in the comments below how this solution has helped you to tidy up your strings. Share any other creative use cases you come across, too!
If you've encountered any other frustrating coding dilemmas, remember to leave a comment with your questions or share them with our helpful community. We'd love to hear from you and help you find solutions!
Happy string cleaning! β¨π§Ή