Is there a "foreach" function in Python 3?
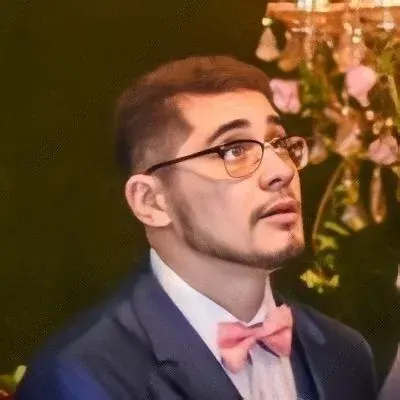
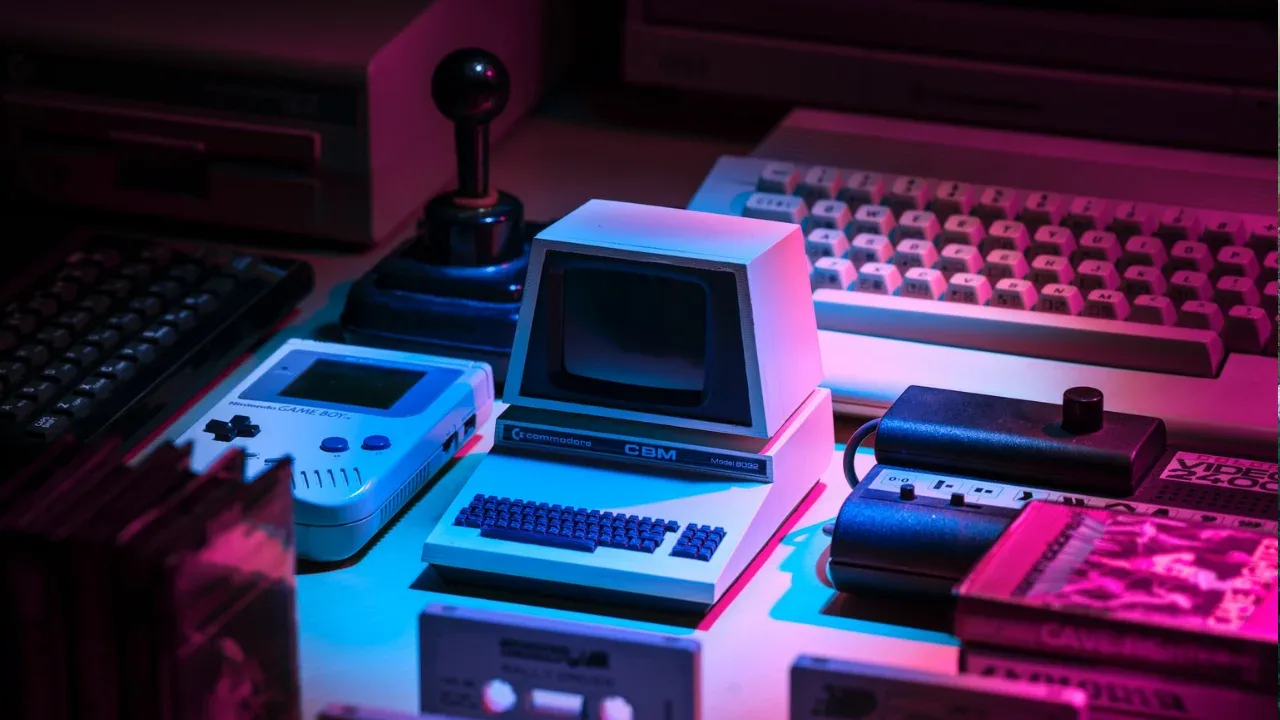
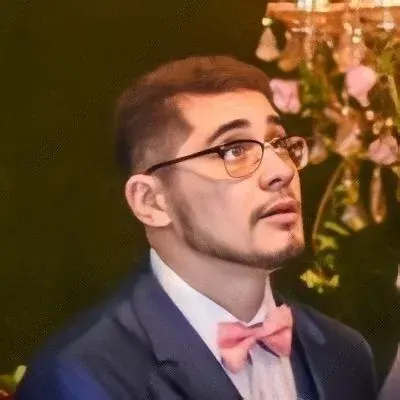
Is there a 'foreach' function in Python 3? π€π»
If you've ever worked with JavaScript, you might be familiar with the convenience of the 'foreach' function. It allows you to perform a specific action on each element of an iterable without worrying about the details of iteration. But what about Python 3? π
Python does not have a built-in 'foreach' function like JavaScript. However, fear not! Python offers a couple of alternatives that can achieve the same functionality. In this blog post, we will explore common issues and provide easy solutions to help you iterate over elements in Python 3. Let's get started! π
The 'foreach' function explained π
In JavaScript, the 'foreach' function takes two parameters: a function and an iterable. It executes the function on each element of the iterable. Here's a simple example in JavaScript:
let nums = [1, 2, 3, 4, 5];
nums.forEach(function(num) {
console.log(num);
});
This code will print each number in the 'nums' array.
Alternative solutions in Python 3 π
While Python doesn't provide a 'foreach' function out of the box, you can achieve similar functionality using different techniques. Let's explore two popular alternatives:
1. Using a for loop π
The most straightforward approach is to use a for loop to iterate over the elements in an iterable. Here's how you can achieve the same action as the 'foreach' function using a for loop in Python:
nums = [1, 2, 3, 4, 5]
for num in nums:
print(num)
The output will be the same as the JavaScript example.
2. Using the 'map' function πΊοΈ
Python offers a built-in 'map' function that can be used to apply a function to each element in an iterable. Here's how you can use the 'map' function to achieve the same result:
nums = [1, 2, 3, 4, 5]
list(map(lambda num: print(num), nums))
The output will again be the same.
Why doesn't Python have a 'foreach' function? π€·ββοΈ
It's a common question among Python developers. While JavaScript provides a convenient 'foreach' function, Python emphasizes readability and simplicity. The for loop and 'map' function are clear and explicit, making the code more understandable. Moreover, Python encourages the use of list comprehensions and generator expressions for more complex iteration scenarios.
Conclusion and call-to-action ππ’
In Python 3, there is no built-in 'foreach' function like in JavaScript. However, you can achieve similar functionality using a for loop or the 'map' function. Remember to choose the solution that best fits your needs and aligns with Python's coding style. Experiment with different approaches and see which one works best for your specific use case.
If you enjoyed this blog post or found it helpful, consider sharing it with your fellow Python enthusiasts. And don't forget to leave a comment below! Let's keep the conversation going. π¬π