Is there a built-in function to print all the current properties and values of an object?
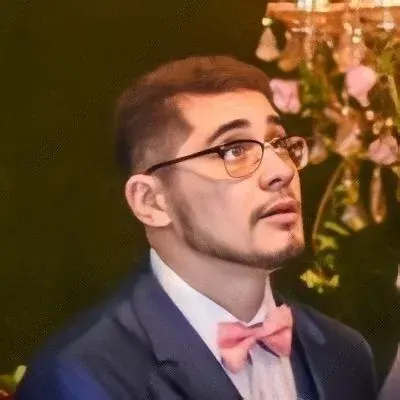
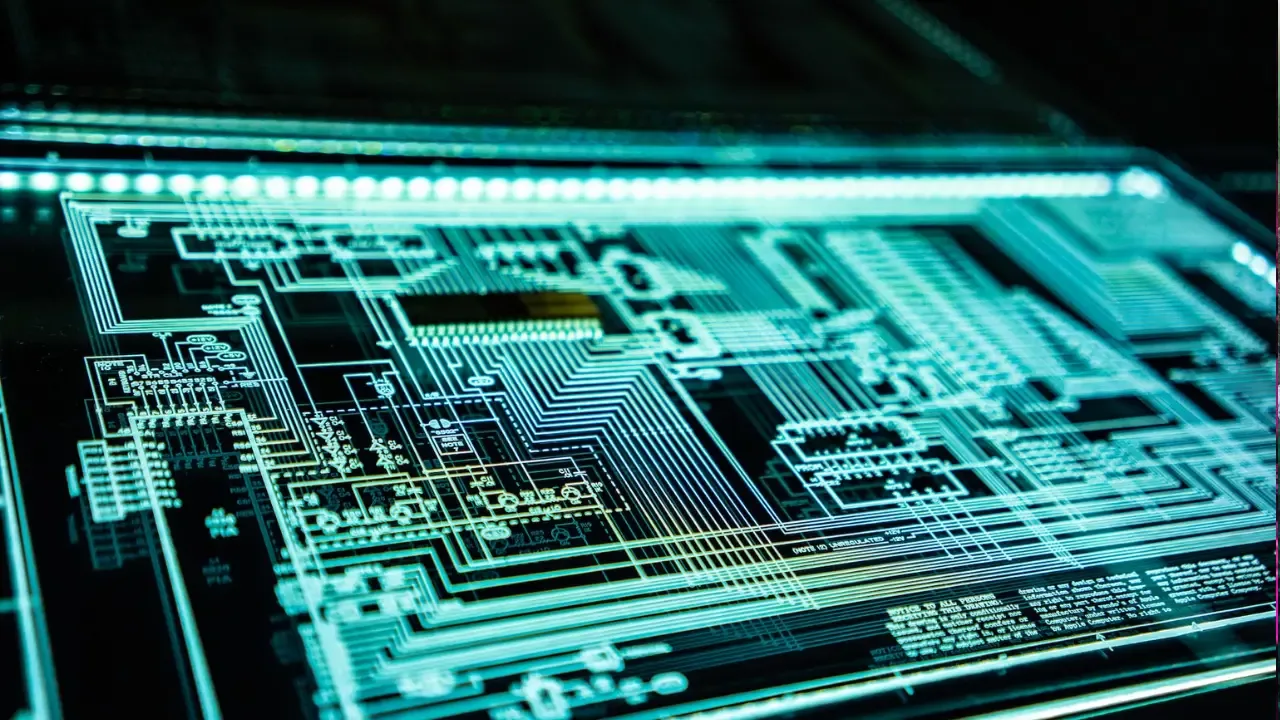
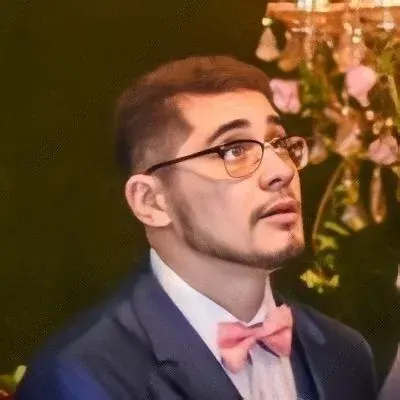
🔍 Looking for a Way to Print Object Properties and Values? 🔍
If you're searching for a quick and convenient way to print all the current properties and values of an object in your code, you're in luck! We've got you covered with a solution that will make your debugging process a whole lot easier. 🎉
🤔 Why Do We Need to Print Object Properties and Values?
Debugging is an essential part of the development process. It allows you to identify and resolve issues in your code effectively. One common technique used by developers is to print the current state of an object, which helps in understanding its properties and values during runtime.
💡 The Power of PHP's print_r Function
You mentioned PHP's print_r
function, which provides a straightforward way to display an object's structure. Unfortunately, in other programming languages like JavaScript, Python, or Java, there is no built-in function that directly mirrors print_r
. But fret not! We have some great alternatives for you. 🙌
⚡ Alternative 1: JSON.stringify in JavaScript
In JavaScript, you can use the JSON.stringify
method to accomplish a similar result. This function converts a JavaScript object into a JSON string representation. Let's take a look at an example:
const myObject = {
name: "John Doe",
age: 25,
profession: "Developer"
};
console.log(JSON.stringify(myObject));
This will output the following JSON string:
{"name":"John Doe","age":25,"profession":"Developer"}
🎉 Alternative 2: pprint in Python
Python developers can take advantage of the pprint
module, which provides a neat way to display complex data structures. Here's an example:
import pprint
myObject = {
"name": "John Doe",
"age": 25,
"profession": "Developer"
}
pprint.pprint(myObject)
The output will be:
{'age': 25, 'name': 'John Doe', 'profession': 'Developer'}
⭐ Alternative 3: Reflection in Java
In Java, you can use reflection to achieve a similar result. Reflection is a powerful tool that allows you to inspect and manipulate classes, methods, and fields at runtime. Here's an example:
import java.lang.reflect.Field;
public class Main {
public static void main(String[] args) {
MyClass myObject = new MyClass("John Doe", 25, "Developer");
Field[] fields = myObject.getClass().getDeclaredFields();
for (Field field : fields) {
field.setAccessible(true);
try {
System.out.println(field.getName() + ": " + field.get(myObject));
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
}
}
class MyClass {
private String name;
private int age;
private String profession;
// Constructor, getters, and setters omitted for brevity
}
The output will be:
name: John Doe
age: 25
profession: Developer
💼 Take It a Step Further!
Now that you know how to print object properties and values, you can enhance your debugging process and streamline your development workflow. 💪
Why not give it a try? Use these techniques in your next project and see the difference they make. Share your experience with us in the comments section below! 👇
Happy coding! 💻✨