Is it worth using Python"s re.compile?
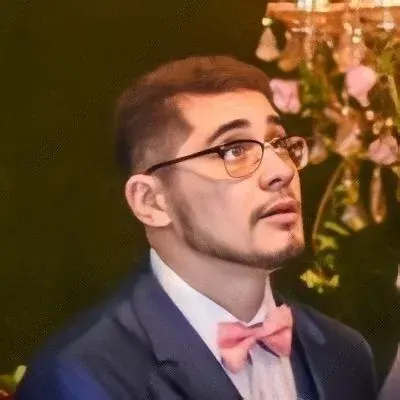
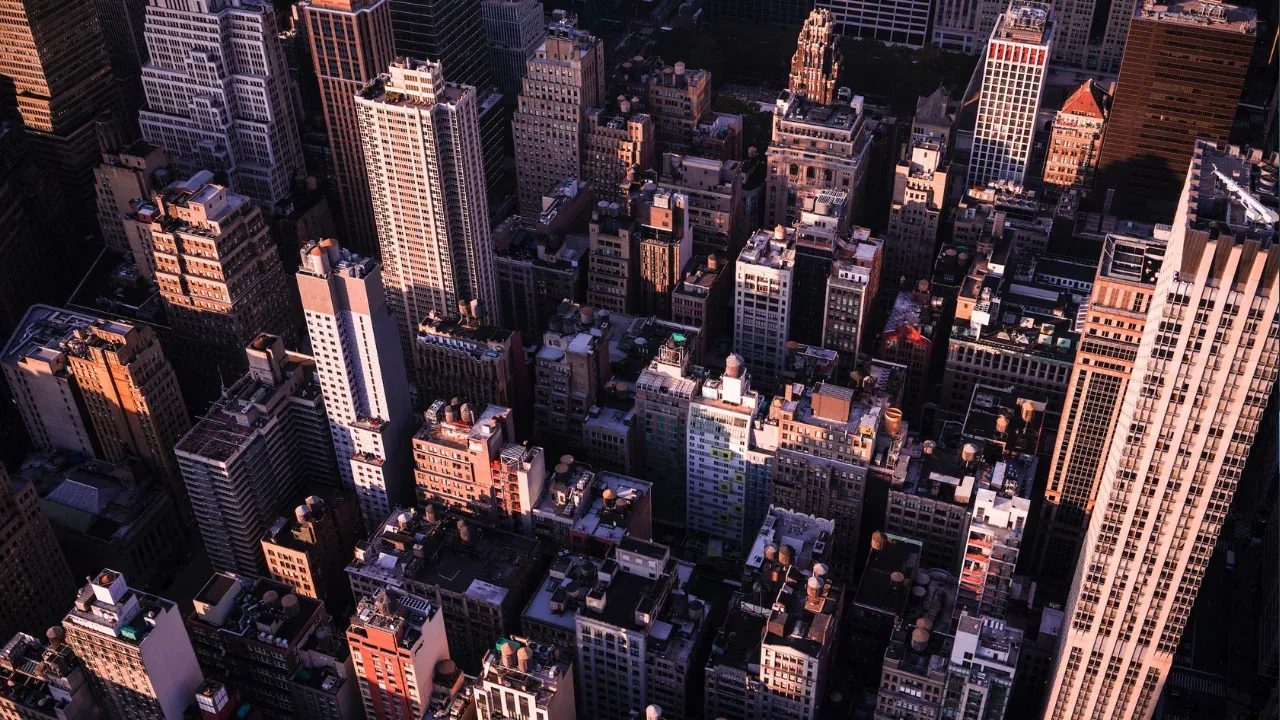
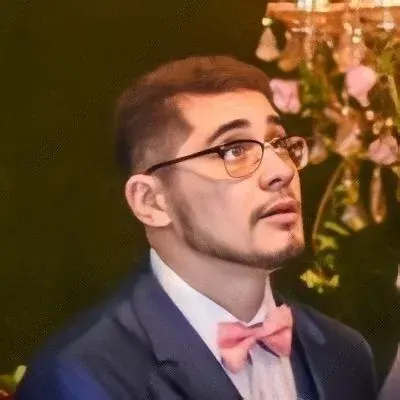
Is it worth using Python's re.compile? 🐍💻
Regular expressions (regex) are powerful tools used to match and manipulate strings. In Python, the re
module provides functionality to work with regex patterns. One common question that arises is whether it's worth using the re.compile
method. Let's dive in and find out! 🏊♀️
The Basics of re.compile
📚
To understand the benefit of using re.compile
, let's first explore how regex matching works without it. In the example provided:
h = re.compile('hello')
h.match('hello world')
vs
re.match('hello', 'hello world')
Both snippets achieve the same result - testing if the string 'hello world'
matches the pattern 'hello'
. However, there's a key difference in how the regular expression is processed.
The Case for re.compile
👏
When using re.match('hello', 'hello world')
, Python internally compiles the regex pattern every time the re.match
function is called. This means that if you use the same regex pattern multiple times, you'll pay the compilation cost each time.
On the other hand, when you pre-compile the pattern using re.compile
, like h = re.compile('hello')
, Python stores the compiled pattern as a regular expression object. You can then reuse this object multiple times without the need for recompilation.
Benefits of Using re.compile
✨
1. Performance Boost ⚡️
By using re.compile
, you eliminate the overhead of repeatedly compiling the same regex pattern. This can yield significant performance improvements, especially when working with large datasets or in speed-sensitive scenarios.
2. Readability Improvement 📖
When you store a compiled regex pattern in a variable, it enhances code readability. A pattern defined once and referenced multiple times throughout your codebase makes it easier to understand the intent of the regular expression.
3. Error Detection 🚦
Using re.compile
allows the Python interpreter to catch any syntax errors in your regex pattern during the compilation stage. This helps identify issues early on and avoids runtime errors that may be harder to troubleshoot.
When Not to Use re.compile
❌
While re.compile
brings several advantages, it's essential to note that it may not always be necessary.
If you only use a regex pattern once, it's often more concise to skip compiling and directly use re.match
or other matching functions. This approach is suitable when you don't anticipate reusing the pattern throughout your code.
Conclusion and Call-to-Action 🎉
In most cases, using re.compile
offers numerous advantages, including improved performance, code readability, and error detection. By taking advantage of pre-compilation, you maximize the efficiency of your regex operations. However, when dealing with one-time or simple patterns, it may not be worth the added complexity.
Next time you encounter a regex pattern that you'll be using over and over again, give re.compile
a try! Your code will be faster, easier to understand, and potentially free of runtime regex errors.
Do you regularly use re.compile
in your projects? What other regex tips do you have? Let's discuss in the comments section below! 👇