Is generator.next() visible in Python 3?
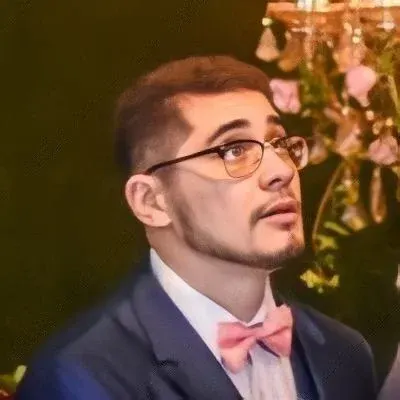
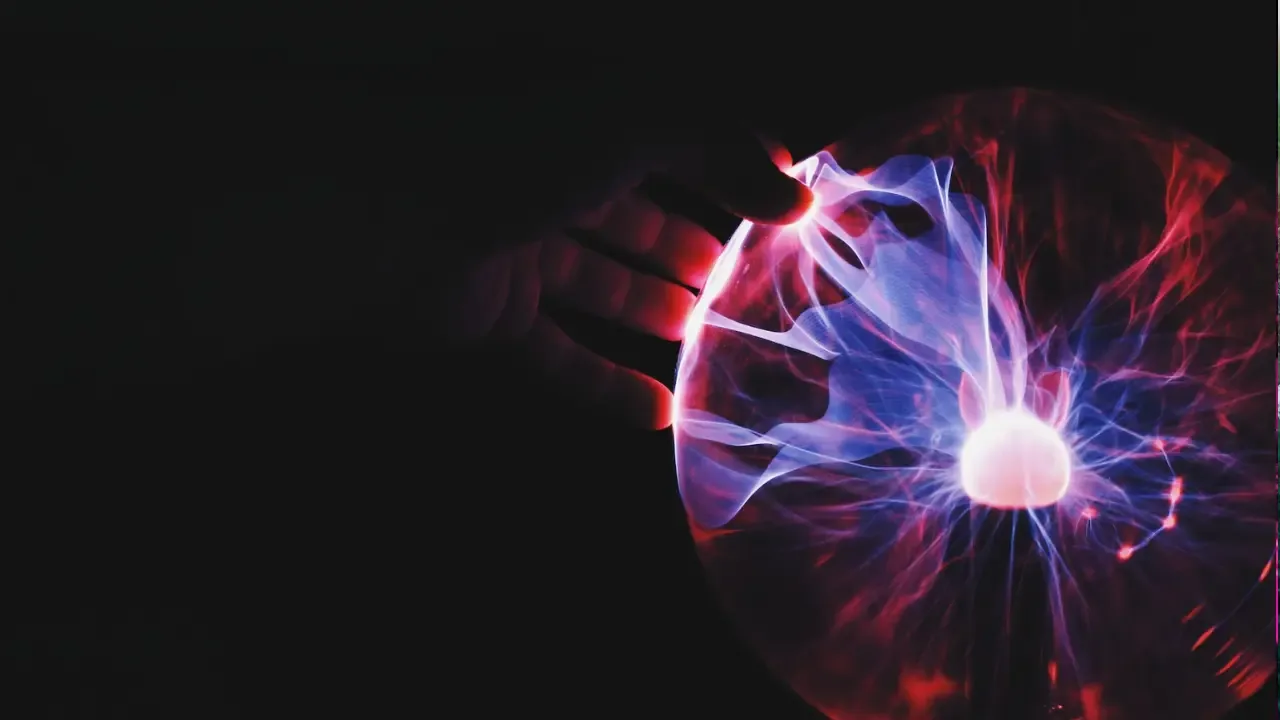
Is generator.next() visible in Python 3? 🤔
Have you ever wondered why the same code that works in Python 2 doesn't work in Python 3? 🐍 Well, you're not alone! Many developers face this confusion and get stuck when trying to use the generator.next()
method in Python 3. But fear not, my friend! In this blog post, we will unveil the reason behind this discrepancy and provide you with easy solutions to overcome it. Let's dive in! 💪
Understanding the Problem 👀
To illustrate the issue, let's consider a simple example: a generator function that generates a series of triangle numbers. In Python 2, you could easily access the next value using the next()
method:
def triangle_nums():
tn = 0
counter = 1
while True:
tn += counter
yield tn
counter += 1
g = triangle_nums() # get the generator
print(g.next()) # get the next value
However, when you run the same code in Python 3, you'll be met with an error message:
AttributeError: 'generator' object has no attribute 'next'
Bummer, right? 😞
The Solution 💡
Fear not, intrepid Pythonista! The reason for this error lies in a small but impactful change made in Python 3. Rather than using the next()
method, Python 3 has adopted a more intuitive approach that aligns with the language's overall philosophy of readability.
In Python 3, you can use the loop iterator syntax to access the next value from a generator:
for n in triangle_nums():
# Do something with n...
Voila! 🎉 The loop takes care of retrieving the next value for you, eliminating the need for the next()
method altogether.
Understanding the Behavior 🤔
Now, you might be wondering why Python made this change. Well, think about it like this: Python is all about simplicity and readability. By using the loop iterator syntax, the code becomes more straightforward and easier to understand. Plus, it aligns with the concept of generators being iterable objects.
Conclusion and Call to Action 📝
And there you have it, folks! The reason behind the absence of generator.next()
in Python 3. While it may seem like a minor difference, understanding this behavior will prevent you from scratching your head when trying to run code that previously worked in Python 2.
So next time you encounter the error "AttributeError: 'generator' object has no attribute 'next'," remember that Python 3 encourages you to iterate over your generators using the loop iterator syntax!
Now it's your turn to share your Python 3 generator experiences! Have you encountered any other differences between Python 2 and Python 3? Let us know in the comments below! And don't forget to hit that share button to spread the word about this helpful insight to your fellow Pythonistas! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
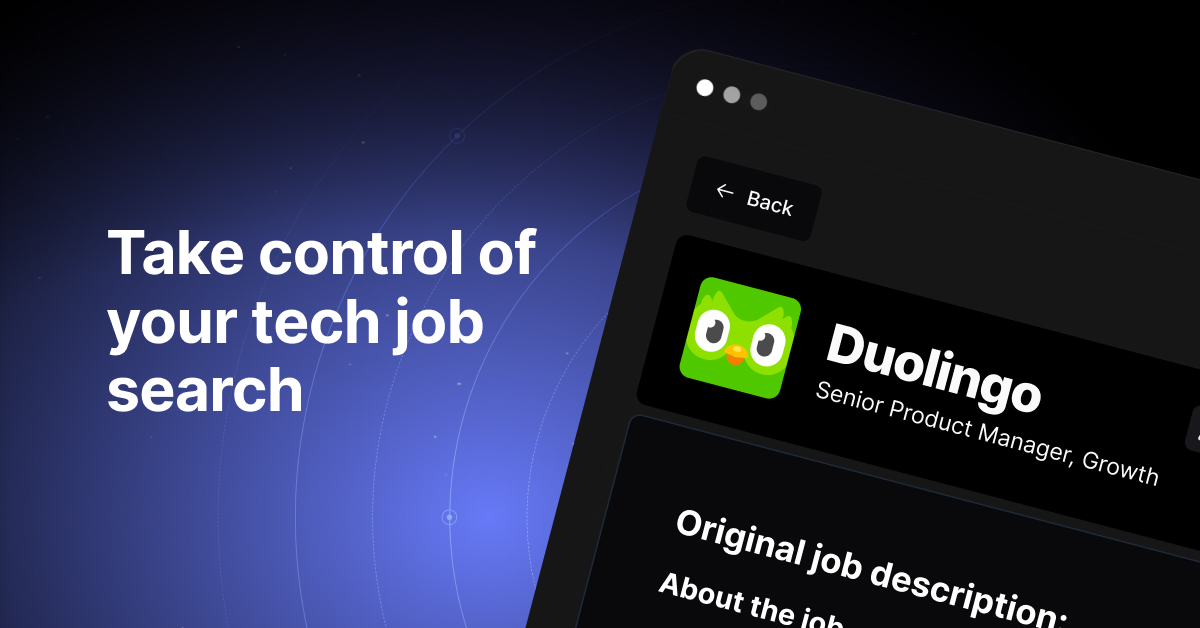