In Python, how do I determine if an object is iterable?
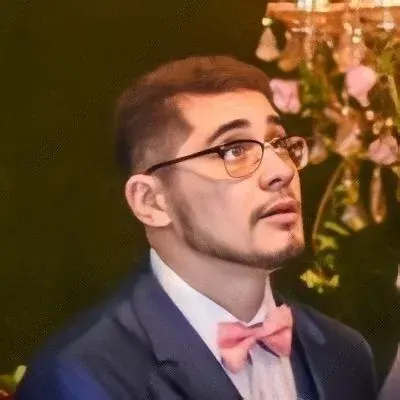
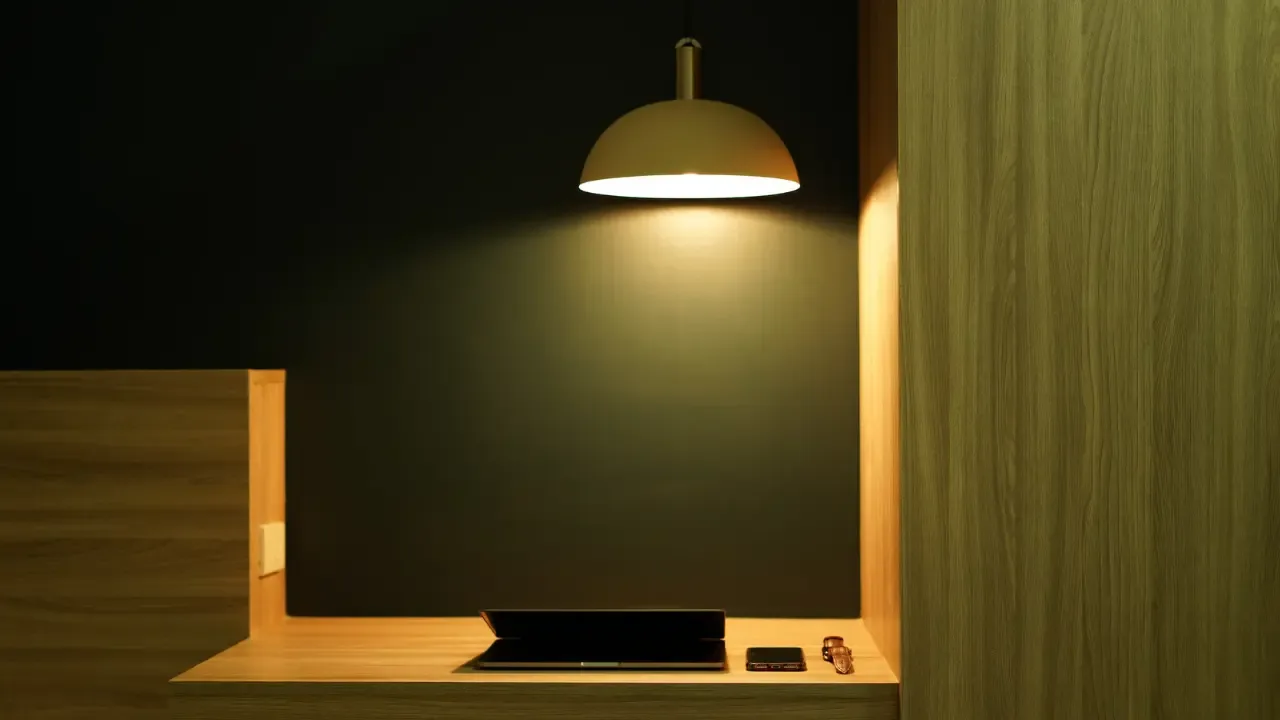
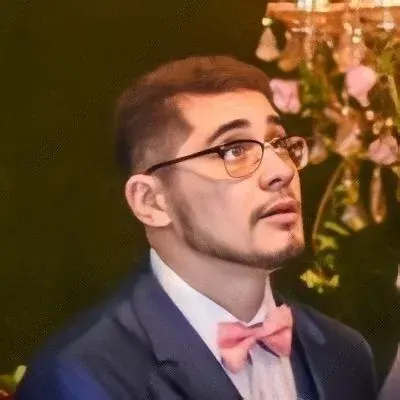
🔎 How to Determine if an Object is Iterable in Python 🐍
So you've stumbled upon a little Python predicament - you want to know if an object is iterable or not. 🤔 Fear not, for we have just the solution for you! In this blog post, we will address this common issue and provide you with easy-to-implement solutions. ✨
🧠 Understanding the Problem: The first step in tackling this challenge is to grasp the concept of iterable objects. An iterable object is one that can be looped over, such as a list, tuple, string, or even a dictionary. Whether it's iterating through a series of elements or accessing one element at a time, determining iterability is crucial when working with different data types.
💡 Quick Solution:
The method you stumbled across, using the hasattr()
function, is indeed a viable option. By checking for the existence of the __iter__
attribute, you can determine if an object is iterable or not. Here's an example using your snippet:
if hasattr(myObj, '__iter__'):
print("The object is iterable 🚀")
else:
print("The object is not iterable ❌")
🤝 But Wait, There's More:
Although the hasattr()
method works fine for most cases, it's not a foolproof solution. Some objects might have a __iter__
attribute but still raise an exception when attempting to iterate over them. 😬 So let's equip ourselves with an even more reliable solution!
🐛 Introducing the iter()
Function:
Python provides the iter()
function, which allows us to check if an object is iterable without relying solely on attributes. This function takes two arguments: the object to be tested and a sentinel value (usually None
). Here's an example:
try:
iterator = iter(myObj)
print("The object is iterable 🚀")
except TypeError:
print("The object is not iterable ❌")
Using a try-except
block, we attempt to create an iterator using iter()
and catch any TypeError
that may arise. If the object is iterable, it will create the iterator successfully, confirming its iterability. If a TypeError
occurs, it means the object is not iterable.
📢 Your Turn to Engage! 📢 Have you ever encountered situations where you struggled to determine if an object is iterable? What approach did you take? Share your thoughts and experiences in the comments below! Let's learn from each other and conquer Python challenges together. 🙌
So the next time you face the question, "Is this object iterable?", remember these tips and choose the method that suits your needs best. Happy coding! 💻🎉