In Django, how do I check if a user is in a certain group?
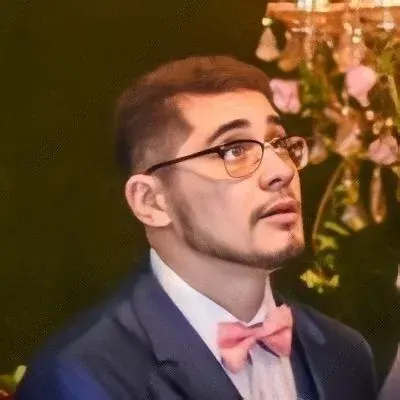
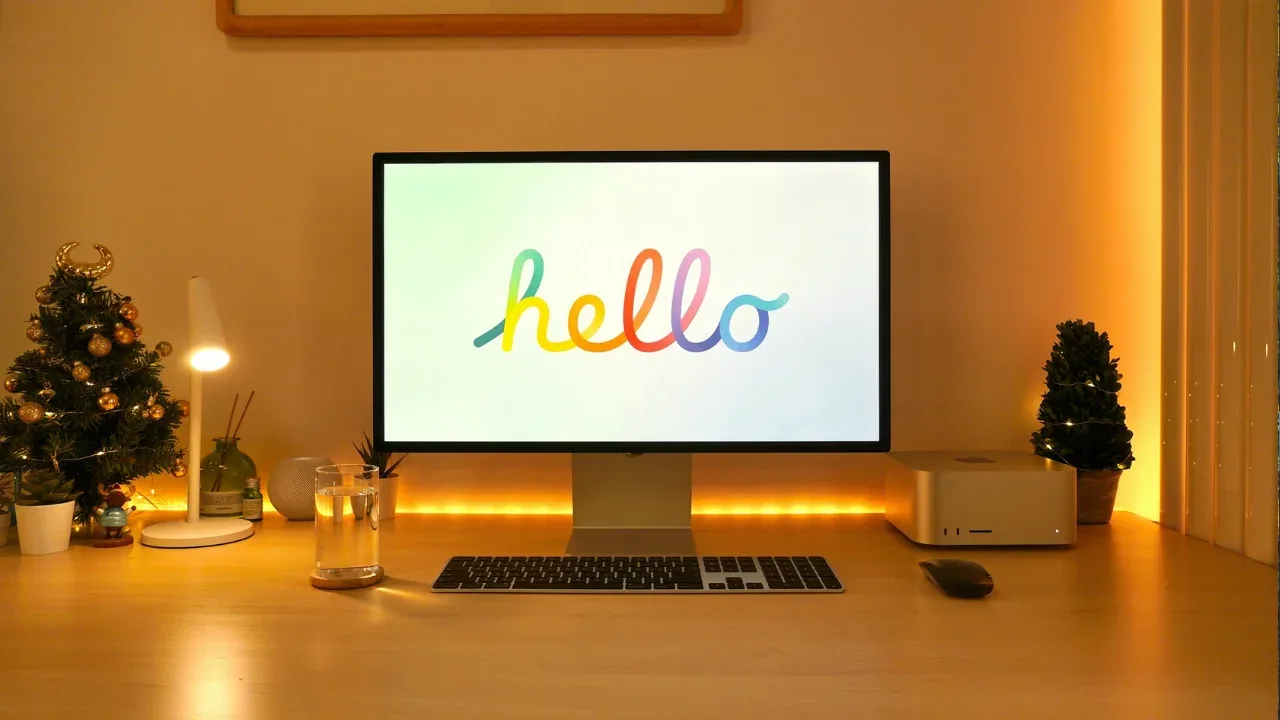
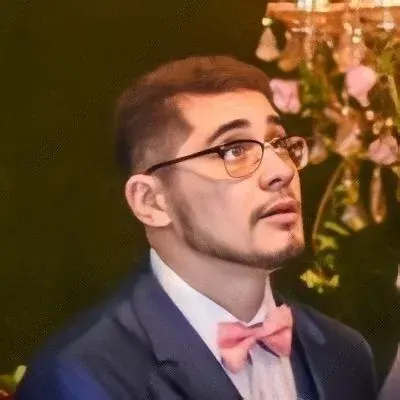
👋 Hey there Django developer! 👩💻
So, you've created a custom group in Django's admin site 🙌, and now you want to check if a user is in this group. That's a great question! Let's dive into it and solve this problem together. 💪
The Challenge 💥
You have a custom group and you want to verify if a user belongs to that group. This can be useful for implementing certain features or restricting access based on user roles. But how do we achieve this in Django? 🤔
The Solution 👍
Fortunately, Django provides a straightforward way to check if a user is in a certain group. You can use the user.groups.filter
method to accomplish this task. Here's an example:
from django.contrib.auth.models import Group
# Assuming "user" is the user instance you want to check
if user.groups.filter(name='your_custom_group_name').exists():
# The user is in the group. Perform your desired action here.
pass
In the above code snippet, we import the Group
model from django.contrib.auth.models
and filter user groups based on the name
attribute. If the group exists in the user's groups, we can then execute our desired action. In this case, we simply have a pass
statement, but you can customize this to fit your needs.
Handling Potential Issues ⚠️
It's important to remember that the name
attribute in the code sample should match exactly with the group name you created in the Django admin site. Keep in mind that group names are case-sensitive.
If you encounter issues with the code not working as expected, here are a few troubleshooting tips:
Double-check spelling and capitalization: Ensure that the group name is spelled correctly and matches the case used when creating the group.
Verify user-group association: Make sure the user is properly associated with the group. You can do this in the Django admin site or programmatically using the
user.groups.add(group)
method.Ensure the user is authenticated: Since only authenticated users have groups, ensure that the user you're checking is logged in.
Take It a Step Further! 🚀
Now that you know how to check if a user is in a certain group in Django, you can start implementing custom functionalities based on user roles! 🔐 Let your imagination run wild and create amazing experiences for your users.
Share Your Thoughts! 💬
Have you ever encountered any difficulties when working with Django groups? How did you solve them? 🤔 Share your experiences and tips in the comments below. Let's learn from each other and make Django development even more enjoyable!
Remember, coding is more fun when we share knowledge, so hit that share button and let your fellow devs join the conversation! 🌟
Happy Pythonic coding! 🐍✨