Importing class from another file
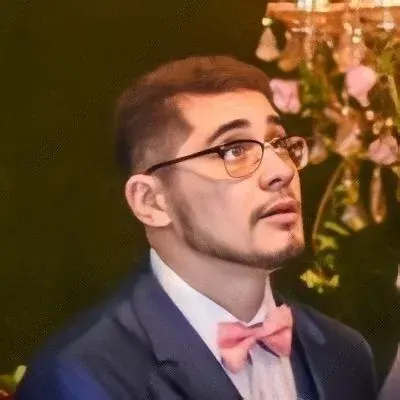
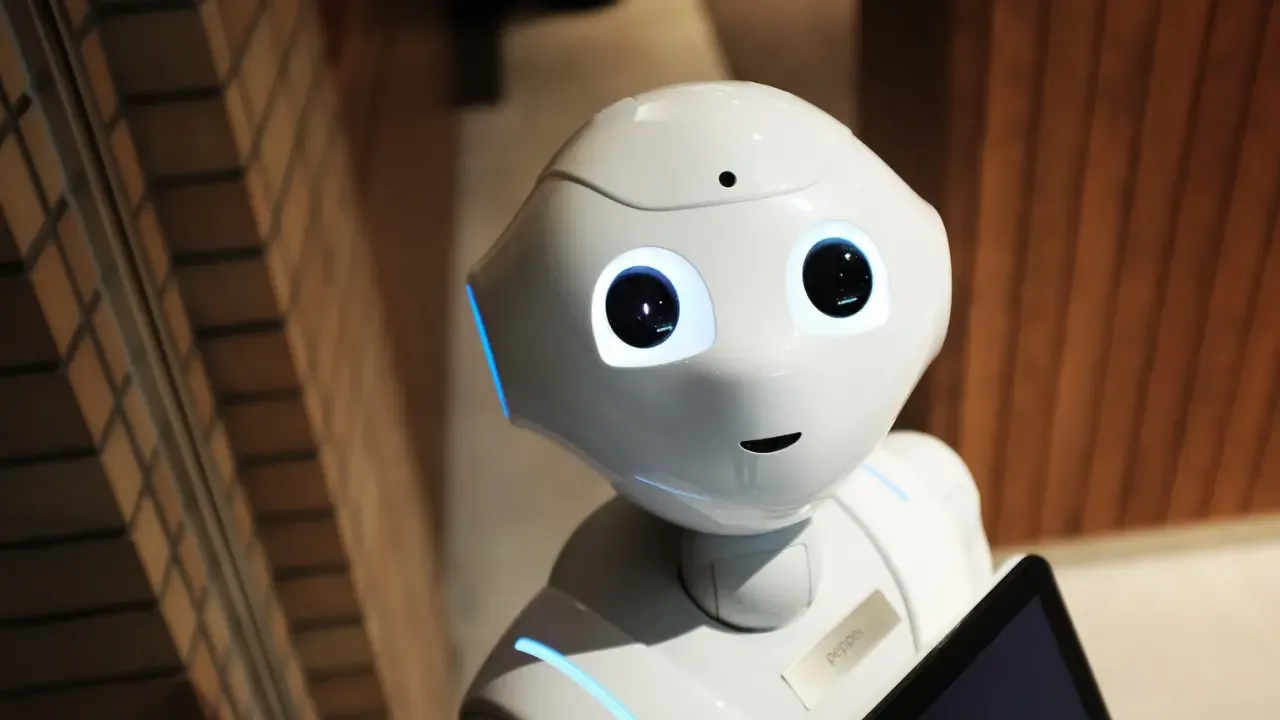
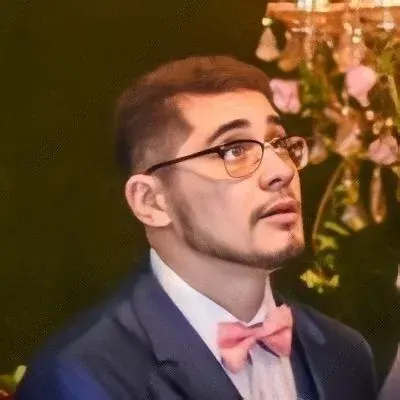
Importing Class from Another File: Common Issues and Easy Solutions 😎💡
Are you having trouble importing a class from another file in Python? Don't worry, you're not alone! This is a common issue that many developers face. In this blog post, we'll address the problem, provide easy solutions, and help you understand what you might be doing wrong. Let's dig in! 🚀
The Problem: Importing a Class from a Subdirectory 🤔
Let's consider a scenario where you have the following file structure:
> main.py
> ---> folder/
> ------> file.py
In file.py
, you have implemented a class called Klasa
. Now, in main.py
, you want to import this Klasa
class. However, when you try to import it using the following code:
from folder import file
from file import Klasa
You encounter an error:
from file import Klasa
ImportError: No module named 'file'
Another attempt to import using:
from folder import file
Also results in an error:
tmp = Klasa()
NameError: name 'Klasa' is not defined
You've tried putting an empty __init__.py
file in the subfolder, but it still doesn't work. You even added from file import Klasa
in __init__.py
, but the issue persists. 😫
The Easy Solutions: Fixing the Import Errors ✅
Correcting the Module Import:
To import a class from a file in a subdirectory, you need to specify the filename without the .py
extension. Update your code in main.py
as follows:
from folder import file
from folder.file import Klasa
By including the subdirectory name (folder
) before the filename (file
), you can access the Klasa
class without any import errors.
Ensuring a Proper Subdirectory Structure:
It's essential to maintain a valid subdirectory structure for Python to recognize it as a package. Ensure that both the folder
and file.py
are within your project's main folder, or make sure the subdirectory is properly defined in your project's root directory.
Checking the PYTHONPATH:
Sometimes, import errors can occur due to incorrect PYTHONPATH configurations. Ensure that your subdirectory (folder
) is included in the PYTHONPATH environment variable. This will allow Python to recognize it as a valid module and import the classes without any issues.
Avoiding Circular Import Dependencies:
If you inadvertently have circular import dependencies between your files, it can lead to import errors. Make sure you don't have any circular dependencies, where file.py
imports from main.py
and vice versa.
Try implementing these solutions, and you should be able to import the Klasa
class successfully! 😄🎉
Have More Questions or Issues? Let's Connect! 🤝
We hope this guide has helped you resolve your importing issues. If you have any more questions or encounter further problems, don't hesitate to reach out. Our team is always here to assist you. 🙌
Please leave a comment below, or connect with us on Twitter or Instagram. We would love to hear your thoughts and help you out!
Remember, importing classes from separate files shouldn't be a stumbling block on your coding journey. Keep learning and building amazing things! 💪🚀
Happy coding! ✨🐍