Import multiple CSV files into pandas and concatenate into one DataFrame
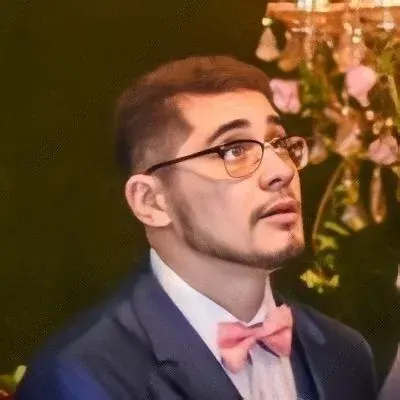
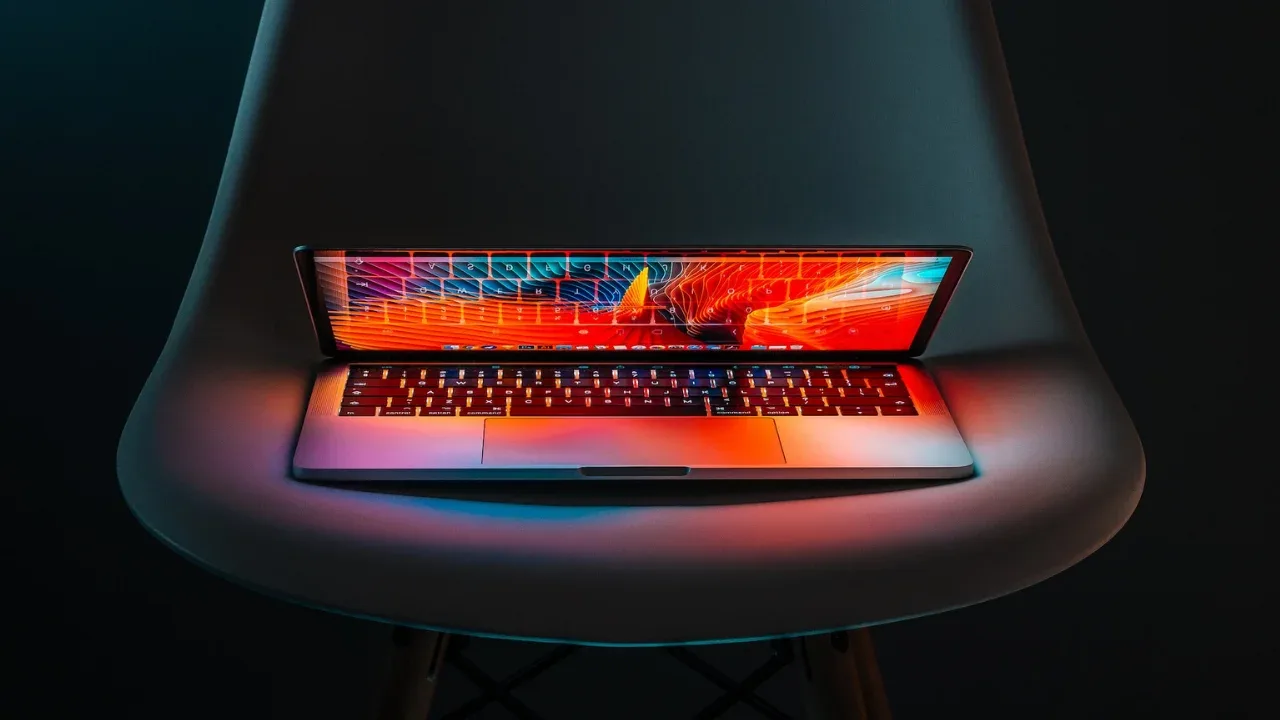
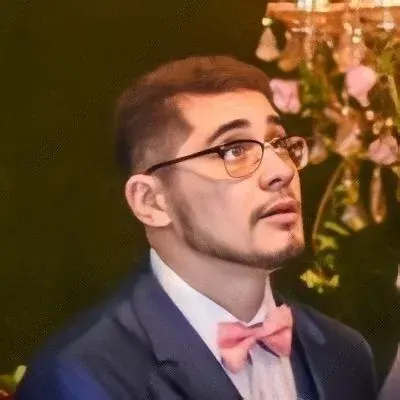
š Title: How to Import and Concatenate Multiple CSV Files into One DataFrame in Pandas
š”š» Introduction
Are you looking to combine multiple CSV files into one DataFrame using pandas, but can't quite figure out how to do it? Don't worry, we've got you covered! In this guide, we'll walk you through the process step by step, addressing common issues and providing easy solutions. By the end, you'll be a pro at importing and concatenating CSV files in pandas!
š§ The Code
Let's start by taking a look at the code you've provided:
import glob
import pandas as pd
# Get data file names
path = r'C:\DRO\DCL_rawdata_files'
filenames = glob.glob(path + "/*.csv")
dfs = []
for filename in filenames:
dfs.append(pd.read_csv(filename))
# Concatenate all data into one DataFrame
big_frame = pd.concat(dfs, ignore_index=True)
Now, let's break it down and explain each step in detail.
š Step 1: Import the Required Libraries
To get started, we need to import the necessary libraries. In this case, we need the glob
module from the standard library to retrieve the filenames and the pandas
library for data manipulation and analysis.
import glob
import pandas as pd
š Step 2: Get the List of CSV File Names
The next step is to retrieve the list of CSV file names from a directory. In your code, you've already defined the path
variable, which represents the directory containing the CSV files.
path = r'C:\DRO\DCL_rawdata_files'
filenames = glob.glob(path + "/*.csv")
Here, the glob.glob
function is used to search for all files with a .csv
extension in the specified directory. It returns a list of file names that match the pattern.
š Step 3: Read and Store CSV Files in DataFrames
Now that we have the list of file names, we can loop through each file, read it using the pd.read_csv
function, and store the resulting DataFrame in a list called dfs
.
dfs = []
for filename in filenames:
dfs.append(pd.read_csv(filename))
In each iteration of the loop, the pd.read_csv
function is called to read a CSV file into a DataFrame, and then that DataFrame is appended to the dfs
list.
š Step 4: Concatenate the DataFrames into One
Finally, we can use the pd.concat
function to concatenate all the DataFrames in the dfs
list into one big DataFrame. The ignore_index=True
parameter ensures that the final DataFrame has a continuous index.
big_frame = pd.concat(dfs, ignore_index=True)
š Congratulations!
You've successfully imported and concatenated multiple CSV files into one DataFrame using pandas. Now you can perform various analyses and manipulations on the combined data!
šØ Common Issues and Troubleshooting
Sometimes, you may encounter issues when importing or concatenating CSV files. Here are a few common problems and their solutions:
File Not Found Error: Double-check that the
path
variable points to the correct directory and that the CSV files exist in that location.Inconsistent Column Names: If the CSV files have different column names or orders, you may end up with mismatched columns in the final DataFrame. Consider renaming or reordering columns before concatenating.
Encoding Errors: If you encounter encoding errors while reading CSV files, try specifying the
encoding
parameter in thepd.read_csv
function, e.g.,pd.read_csv(filename, encoding='utf-8')
.Memory Limitations: Concatenating a large number of massive CSV files may exceed your system's memory capabilities. In such cases, consider processing the files in chunks or using alternative memory-efficient techniques.
Remember to keep these solutions in mind when facing similar issues during the import and concatenation process.
šÆ Call-to-Action
Now that you've learned how to import and concatenate multiple CSV files into one DataFrame, go ahead and give it a try! You can apply this knowledge to combine any CSV files that you need for your data analysis tasks. Experiment with different files, explore the pandas library, and unlock new insights from your data!
š£ Did you find this guide helpful?
If you enjoyed this guide and found it useful, please consider sharing it with your fellow data enthusiasts or anyone who might benefit from this knowledge. Remember, sharing is caring! š
Let us know in the comments below if you have any questions, suggestions, or if there are any other topics you'd like us to cover in future blog posts. We value your feedback and are always here to help!
Happy analyzing! šš