HTTP requests and JSON parsing in Python
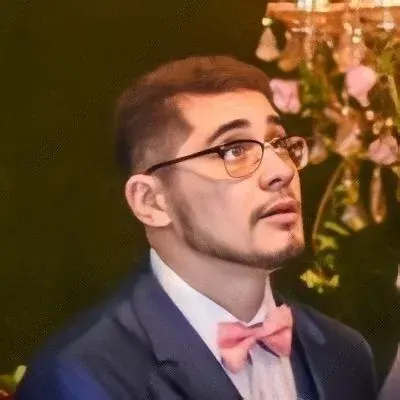
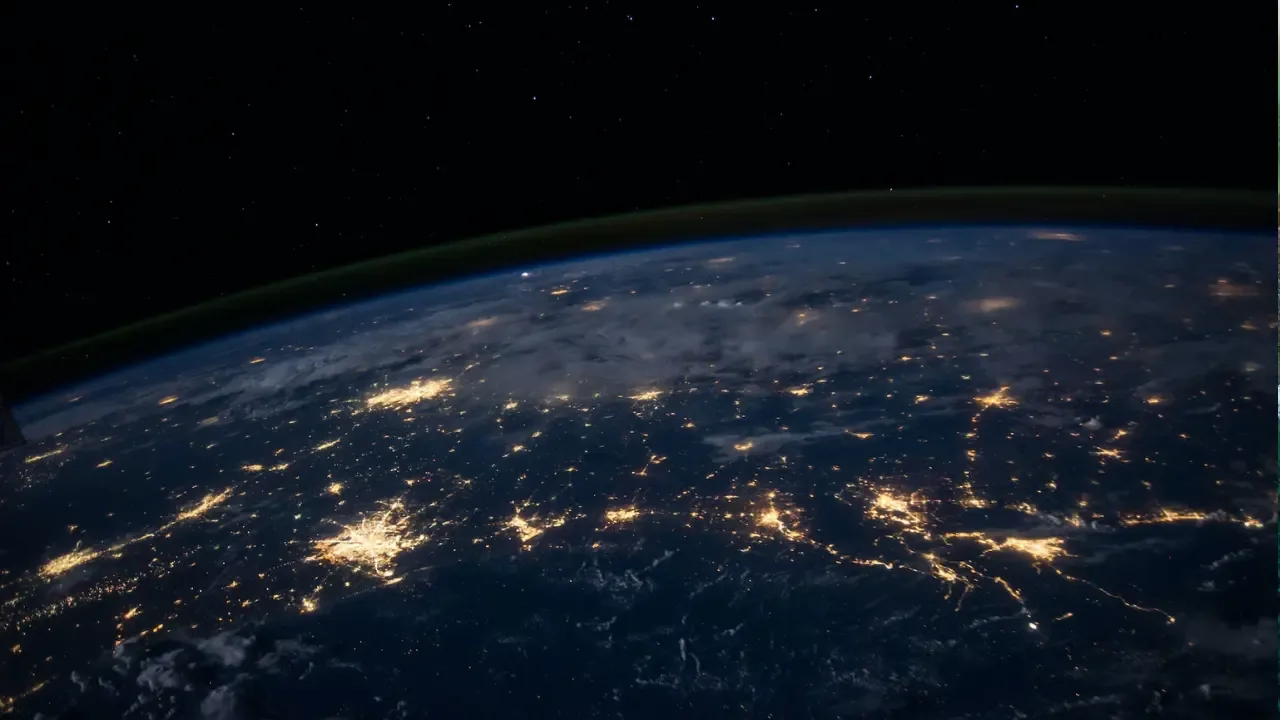
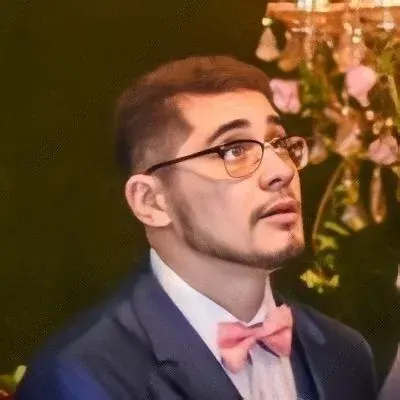
π Title: Mastering HTTP Requests and JSON Parsing in Python
Introduction: Hey, Pythonistas! π Are you ready to unlock the power of dynamically querying the Google Maps API and parsing the JSON response like a pro? ππΊοΈ In this guide, we'll dive into the world of HTTP requests and JSON parsing in Python to help you conquer any roadblocks you might encounter. Let's hit the road, shall we? ππ¨
Understanding the Challenge: So, you want to send an HTTP request to the Google Directions API and receive the result in JSON format. No worries, we got your back! Here's a sample request to calculate the route from Chicago, IL to Los Angeles, CA with waypoints in Joplin, MO and Oklahoma City, OK:
http://maps.googleapis.com/maps/api/directions/json?origin=Chicago,IL&destination=Los+Angeles,CA&waypoints=Joplin,MO|Oklahoma+City,OK&sensor=false
The response you'll get will be in JSON format, which you can then extract and utilize in your Python code. π
Solution:
Installing the Required Libraries:
To start our adventure, make sure you have the requests
library installed. You can do this by running the command pip install requests
.
Sending the HTTP Request:
Now, let's get behind the wheel and send our HTTP request using Python's requests
library. Here's a code snippet to get you going:
import requests
url = "http://maps.googleapis.com/maps/api/directions/json"
params = {
"origin": "Chicago,IL",
"destination": "Los+Angeles,CA",
"waypoints": "Joplin,MO|Oklahoma+City,OK",
"sensor": "false"
}
response = requests.get(url, params=params)
Handling the Response:
Once we receive the response, we can access the JSON data and interact with it. Here's how you can extract the JSON content:
json_data = response.json()
Parsing the JSON:
Let's say you want to retrieve the distance and duration of the calculated route. We can accomplish this by navigating through the JSON structure. Here's an example:
routes = json_data["routes"]
leg = routes[0]["legs"][0]
distance = leg["distance"]["text"]
duration = leg["duration"]["text"]
print("Distance:", distance)
print("Duration:", duration)
Hit the Gas: Now that you've mastered the art of sending HTTP requests and parsing JSON in Python, you can take your projects to the next level! π Why not experiment with different endpoints or combine this knowledge with other APIs to build something amazing? The possibilities are endless! π
We hope this guide helped you navigate through the winding roads of HTTP requests and JSON parsing in Python. Remember, the journey might be challenging at times, but with your determination, you'll always find a way forward. π
So, buckle up, keep coding, and let us know about your amazing creations in the comments below! ππ»β¨