How would you make a comma-separated string from a list of strings?
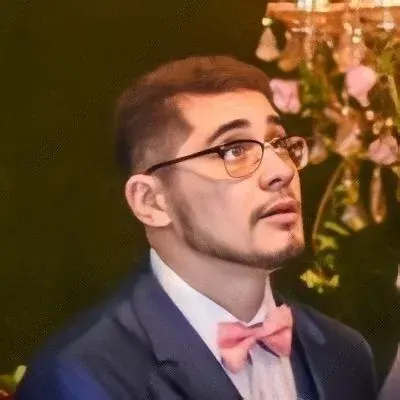
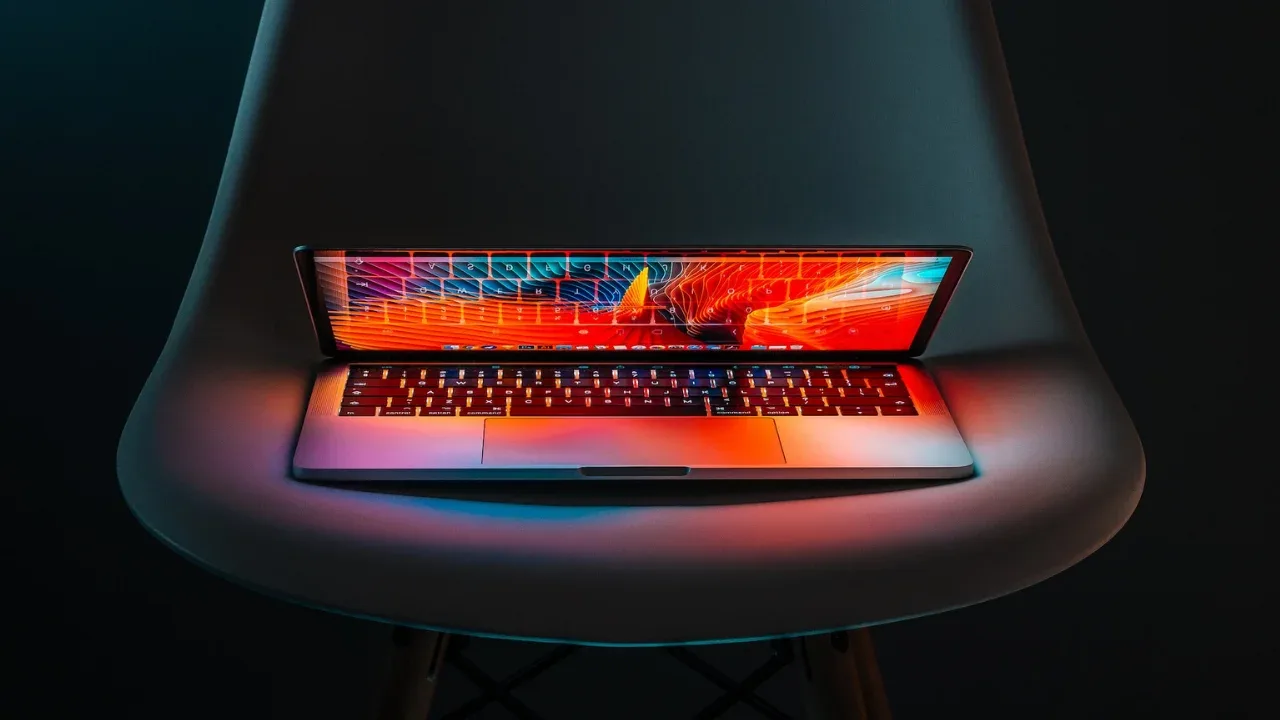
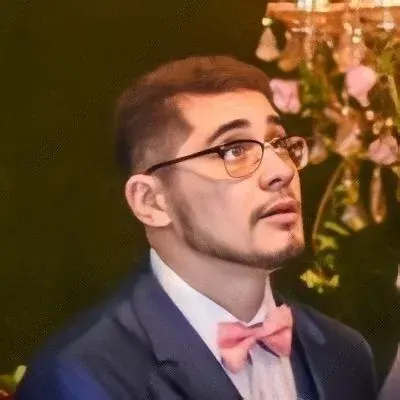
π Making a Comma-Separated String from a List of Strings! π
Have you ever found yourself scratching your head, wondering how to transform a list of strings into a comma-separated string? π€ Well, fear not! In this handy blog post, we'll explore common issues and provide you with easy solutions to tackle this problem. So, let's dive right in!
The Question at Hand
The challenge is to take a list of strings, like ['a', 'b', 'c']
, and transform it into a comma-separated string, like 'a,b,c'
. Simple enough, right? But what about edge cases like ['s']
and []
? These should respectively turn into 's'
and ''
βmake sure to keep those in mind!
The Usual Suspect
Now, you might be familiar with a solution like this: ''.join(map(lambda x: x+',',l))[:-1]
. While it gets the job done, there's something unsatisfying about it. Plus, with all those parentheses and lambda expressions, it can feel a bit daunting. π«
Enter the Wonderous 'join' Method
Fear not, for there's a simpler and more elegant solution! π Let me introduce you to the amazing 'join' method. This little gem allows you to concatenate strings from a list, using a specified separatorβin our case, a comma!
my_list = ['a', 'b', 'c']
result = ','.join(my_list)
print(result) # Output: 'a,b,c'
VoilΓ ! With just one line of code, you can transform your list into a beautiful comma-separated string. π₯³ But remember, the 'join' method works its magic only when all elements in the list are strings. So, be careful not to mix and match different data types!
Handling Edge Cases
Now, let's tackle those tricky edge cases. As we mentioned earlier, a list with a single string should return that string, whilst an empty list should simply return an empty string.
single_item_list = ['s']
result = ','.join(single_item_list)
print(result) # Output: 's'
empty_list = []
result = ','.join(empty_list)
print(result) # Output: ''
No special tricks or extra code needed! The 'join' method gracefully handles these cases for you. π«
Your Turn to Shine!
Now that you've learned the secret to creating comma-separated strings from lists, go ahead and give it a try! Experiment with different lists, add your own twist to the code, and have fun. π
If you have any questions, suggestions, or an even better approach to solving this problem, let us know in the comments below. We can't wait to hear from you! π
Remember, sharing is caring! If you found this blog post helpful, share it with your friends and colleagues who might also benefit from this knowledge. Spread the coding joy! π
That's all for now, folks! Until next time, happy coding! π
P.S. Don't forget to bookmark this post for future reference. Who knows, you might need it again someday! π