How to write to an existing excel file without overwriting data (using pandas)?
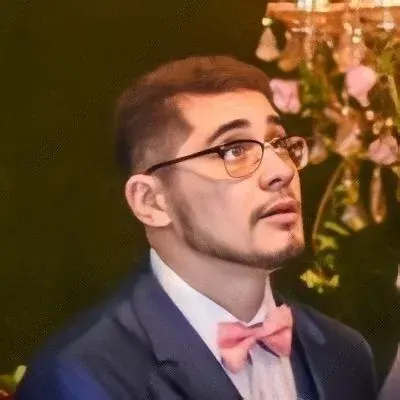
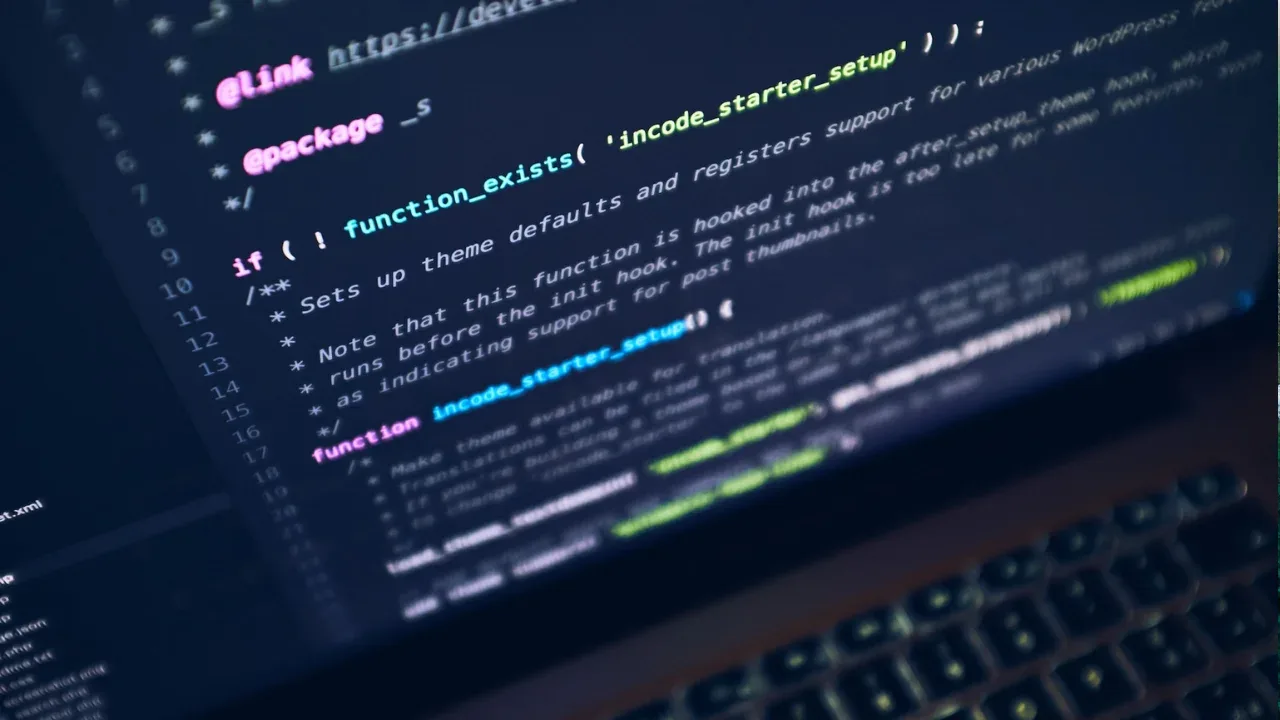
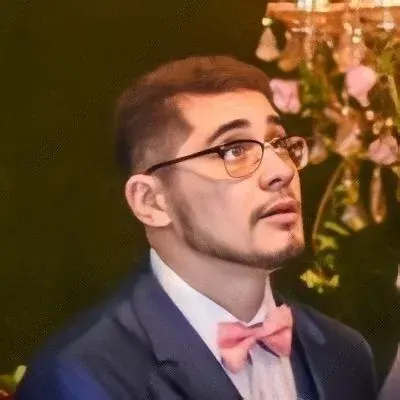
π How to Write to an Existing Excel File without Overwriting Data (Using pandas) πΌπ
Are you a data enthusiast who loves using pandas to analyze and manipulate data in Python? If so, you've probably come across the challenge of writing to an existing Excel file without accidentally overwriting any data. Don't worry, you're not alone! Many pandas users have faced this common issue when trying to add new sheets to an existing Excel file without losing the existing ones.
In this article, we'll guide you through the process of writing to an existing Excel file while preserving the existing data using pandas. We'll explore common issues and provide you with easy solutions so you can save time and avoid frustration. Let's dive in! πͺπ»
π© The Problem: Overwriting Existing Tabs in Excel
The code snippet you shared demonstrates a typical approach to writing to an Excel file using pandas. However, it has an unintended consequence β when you write to a specific sheet, all other existing sheets in the Excel file are deleted. This behavior can be frustrating, especially if you have spent a significant amount of time organizing and formatting your data in multiple tabs. π«
π― The Solution: Use the pandas append
Method
Fortunately, pandas provides a simple yet powerful method called append
that allows us to add new sheets to an existing Excel file without overwriting any data.
Here's an updated version of your code that leverages the append
method:
import pandas as pd
# Load the existing Excel file
existing_file = pd.ExcelFile('Masterfile.xlsx')
# Create a new ExcelWriter instance with the existing file
writer = pd.ExcelWriter('Masterfile.xlsx', engine='openpyxl')
# Copy the existing sheets to the new ExcelWriter
for sheet_name in existing_file.sheet_names:
df = existing_file.parse(sheet_name)
df.to_excel(writer, sheet_name, index=False)
# Create a new sheet and write the desired data to it
data_filtered.to_excel(writer, "Main", index=False, columns=['Diff1', 'Diff2'])
# Save and close the writer
writer.save()
writer.close()
Here's what's happening in the updated code:
We use
pd.ExcelFile
to load the existing Excel file into anexisting_file
object.We create a new
ExcelWriter
instance and specify the existing file as the target.We iterate over the sheet names in the
existing_file
object.For each sheet, we parse the data into a DataFrame (
df
) and write it to the newExcelWriter
using the same sheet name.Finally, we create a new sheet called "Main" and write the
data_filtered
DataFrame to it.
By using the append
approach, we preserve all existing sheets in the Excel file, including their data and formatting. ππ
π Time to Take Action!
Now that you've learned how to write to an existing Excel file without overwriting any data, it's time to put your knowledge into practice. Grab your favorite dataset, locate your Excel file, and give it a try using the append
method. Feel the excitement as you add new sheets to your Excel file while keeping your hard-earned data intact! π
We hope this guide has been helpful in addressing the common issue of overwriting tabs in Excel when using pandas. By using the append
method, you can confidently write to existing Excel files without the fear of losing any valuable information. If you found this article useful, be sure to share it with your friends and colleagues who are also pandas enthusiasts. π€π€
If you have any questions or want to share your experience with writing to Excel files using pandas, don't hesitate to leave a comment below. Let's continue the conversation! ππ¬
Happy pandas coding, and may your Excel files always be organized and intact! πΌππΌ