How to use XPath in Python?
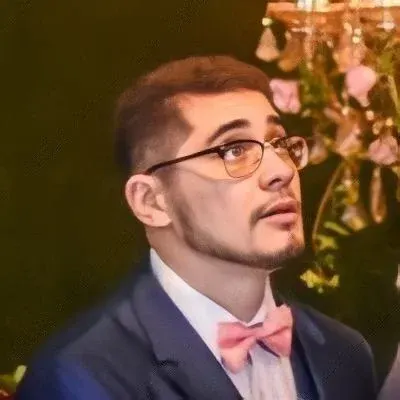
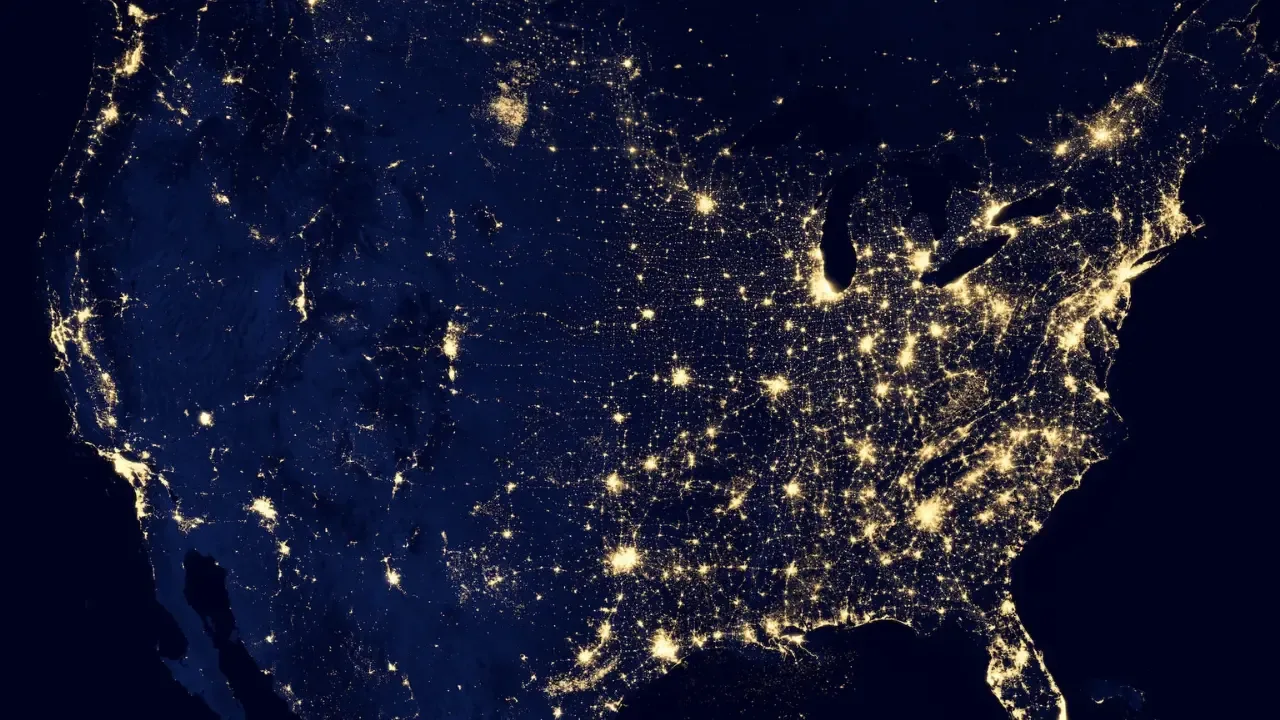
🤔 Introduction:
Hey there tech enthusiasts! If you've ever found yourself scratching your head 🤔 and wondering how to use XPath in Python, fear not! 🙌 In this blog post, we'll explore the magical world of XPath and learn how to wield its power with Python 🐍. Whether you're a beginner or an experienced developer, we've got you covered! Let's dive right in! 💪
💡 Understanding XPath:
XPath (XML Path Language) is a powerful query language used to navigate and extract data from XML documents. It provides a way to traverse through the elements and attributes of an XML structure using a unique syntax. So if you're dealing with XML data and want to extract specific information, XPath is here to save the day!
📚 XPath Libraries for Python:
Python has some fantastic libraries that make working with XPath a breeze. Let's take a look at a few popular ones:
1️⃣ lxml: Lxml is a high-performance library that combines XPath with ElementTree API, offering a full XPath implementation. This powerful library provides support for parsing, modifying, and manipulating XML and HTML documents. To get started, ensure you have lxml installed by running pip install lxml
in your terminal.
2️⃣ xml.etree.ElementTree: This library is included in Python's standard library and provides a lightweight and efficient way to parse and navigate XML documents. While it doesn't offer the full range of XPath features, it's still a handy tool for simpler XML processing tasks. No additional installation is required as it comes bundled with Python.
🔬 Using XPath in Python:
Now that we have our libraries sorted, let's dive into how to use XPath in Python. We'll use the lxml library as an example, but the concepts will translate to other libraries as well. Here's a step-by-step guide to get you started:
1️⃣ Import the necessary modules: Begin by importing the required modules from the lxml library.
from lxml import etree
2️⃣ Parse the XML document: Next, you'll need an XML document to work with. You can either load it from a file or create an XML string directly. For this example, we'll load it from a file.
tree = etree.parse("path/to/your/xml/file.xml")
3️⃣ Create an XPath object: Once the XML document is parsed, create an XPath object to perform XPath queries.
xpath_selector = etree.XPath("your_xpath_expression")
4️⃣ Execute the XPath query: With the XPath object in hand, you can execute XPath queries on the XML document.
result = xpath_selector(tree)
5️⃣ Process the results: Finally, you can process the results returned by the XPath query.
for element in result:
# Do something useful with each element
And voilà! 🎉 You've successfully used XPath in Python to navigate and retrieve data from XML!
💥 Call to Action:
XPath is an indispensable tool when it comes to extracting information from XML documents, and with Python at your side, the possibilities are endless! 🚀 So why not give it a try and let us know your amazing XPath adventures in the comments below? We'd love to hear your success stories and answer any questions you might have! Happy XPathing! 😃💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
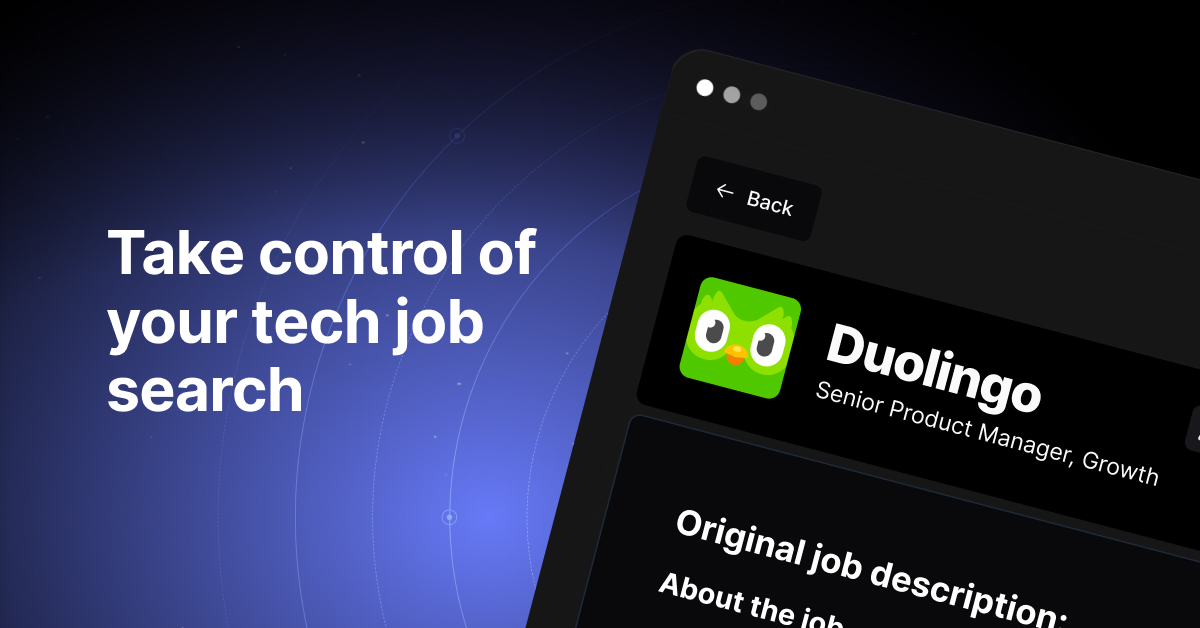