How to use `subprocess` command with pipes
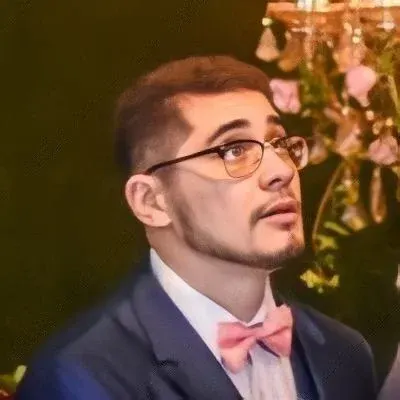
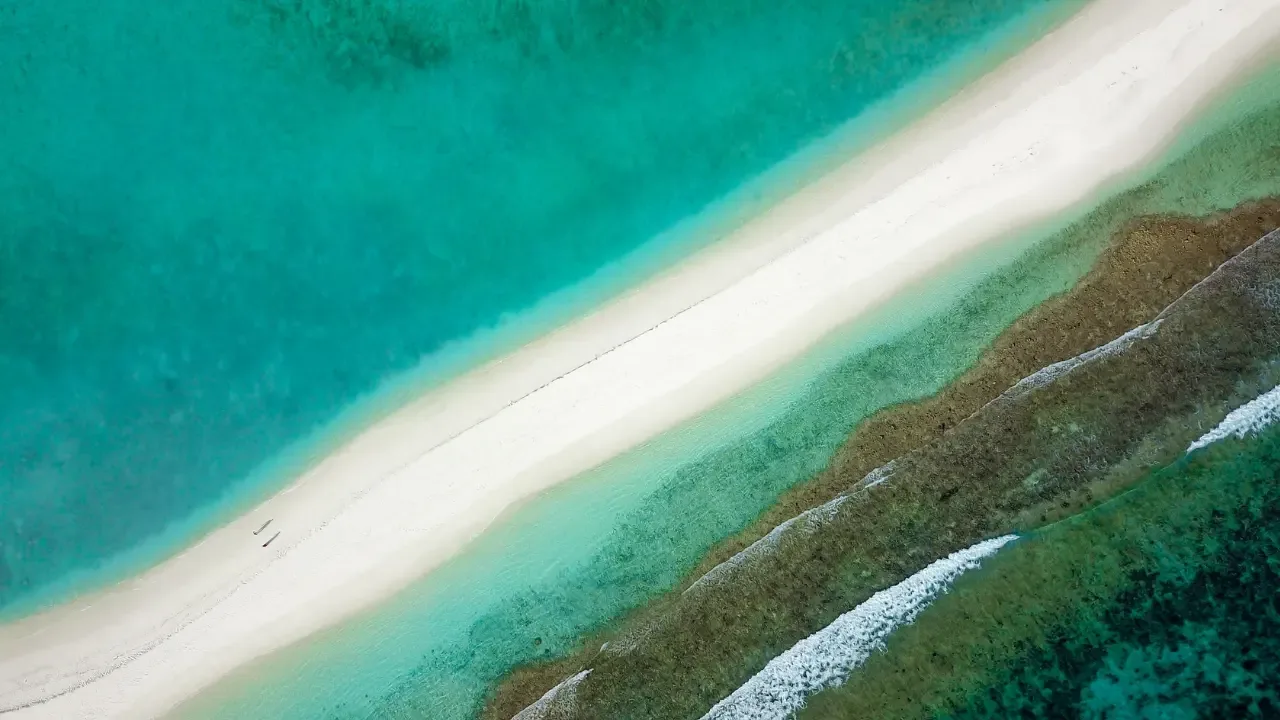
🚀 Supercharge your Python scripts with subprocess
command and pipes!
Are you tired of running your Python scripts in isolation? Would you like to tap into the power of the command line and utilize powerful shell commands within your scripts? Look no further! In this blog post, we'll explore how to use the subprocess
module in Python, specifically focusing on how to use it with pipes to achieve complex command-line functionality. 💪🐍
🧐 Understanding the problem
Let's start by understanding the specific problem at hand. Our reader wants to use subprocess.check_output()
in combination with the shell command: ps -A | grep 'process_name'
. This command, when executed in a shell, lists all running processes and filters them based on the provided name.
The issue is that simply passing the entire command as a single string to subprocess.check_output()
doesn't work. So how can we solve this?
💡 The solution: Using pipes with subprocess
To tackle this problem, we need to break down the shell command into individual commands, and then use pipes to connect them together. Here's an easy solution using the subprocess
module:
import subprocess
# Splitting the shell commands into a list
ps_command = ["ps", "-A"]
grep_command = ["grep", "process_name"]
# Executing the commands and connecting them with a pipe
ps_output = subprocess.check_output(ps_command)
filtered_output = subprocess.check_output(grep_command, input=ps_output)
Let's break down the code to understand what's happening:
First, we split the command
ps -A
into two separate commands:["ps", "-A"]
.Next, we split the
grep
command into["grep", "process_name"]
.We then execute the
ps
command and capture its output usingsubprocess.check_output()
. This output is stored in theps_output
variable.Finally, we pass the
ps_output
as theinput
argument tosubprocess.check_output()
while executing thegrep
command. This connects the two commands together, emulating the behavior of pipes.
Voila! You now have the filtered output from the grep
command in the filtered_output
variable. 🎉
✨ Handling common issues
Working with subprocess
and pipes can sometimes lead to unexpected issues. Let's address a few common problems and provide easy solutions:
1. 🛑 subprocess.CalledProcessError: Command '...' returned non-zero exit status ...
If you encounter this error, it means that one of the commands in the pipe chain has failed. To handle it gracefully, you can wrap your subprocess.check_output()
calls within a try-except block, like this:
try:
ps_output = subprocess.check_output(ps_command)
filtered_output = subprocess.check_output(grep_command, input=ps_output)
except subprocess.CalledProcessError as e:
print(f"Error: {e}")
2. 📝 Working with binary data
By default, subprocess.check_output()
returns the output as a bytes object, which is suitable for text data. However, if you're dealing with binary data, you can pass an additional argument universal_newlines=True
to decode the output as text:
ps_output = subprocess.check_output(ps_command, universal_newlines=True)
filtered_output = subprocess.check_output(grep_command, input=ps_output, universal_newlines=True)
📣 Your turn to shine!
Now that you've learned how to use subprocess
with pipes in Python, it's time to put your skills to the test! Try using this approach to accomplish tasks that require complex shell commands within your Python scripts.
Got any questions? Stuck somewhere? Feel free to reach out in the comments section below. Let's collaborate and troubleshoot together! 💬🤝
Happy coding! 😄🐍
Note: This blog post assumes basic knowledge of the subprocess
module in Python. If you're new to it, it's worth checking out the official documentation for more information.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
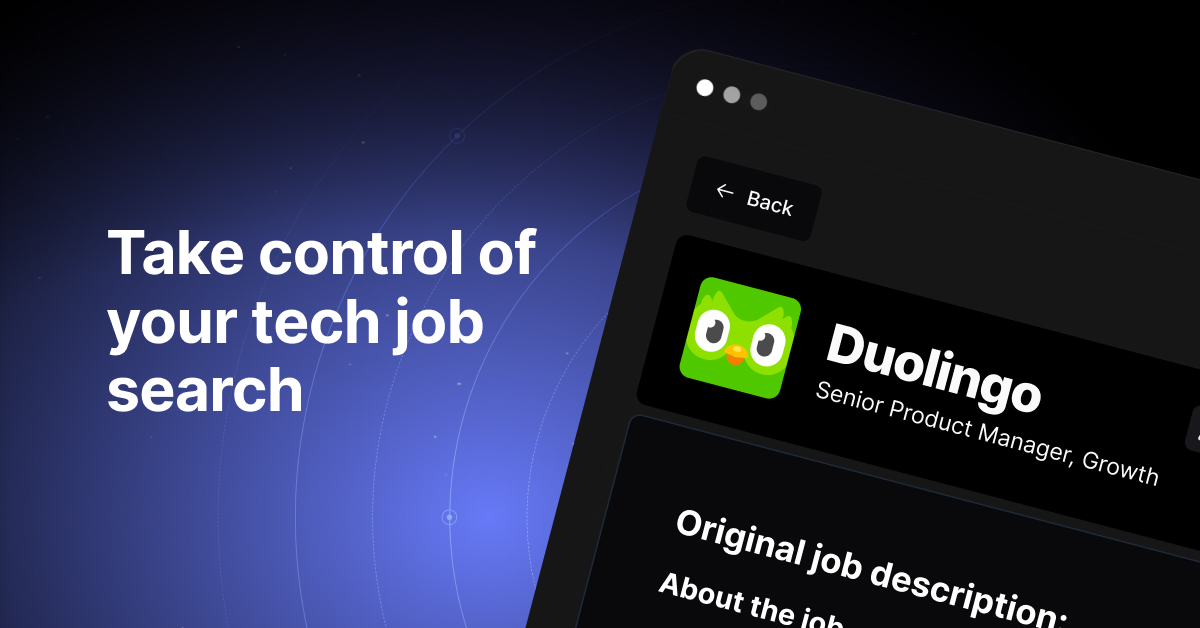