How to use StringIO in Python3?
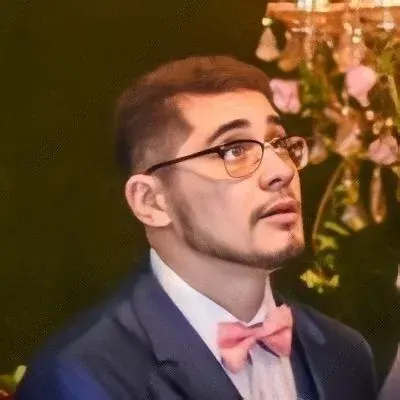
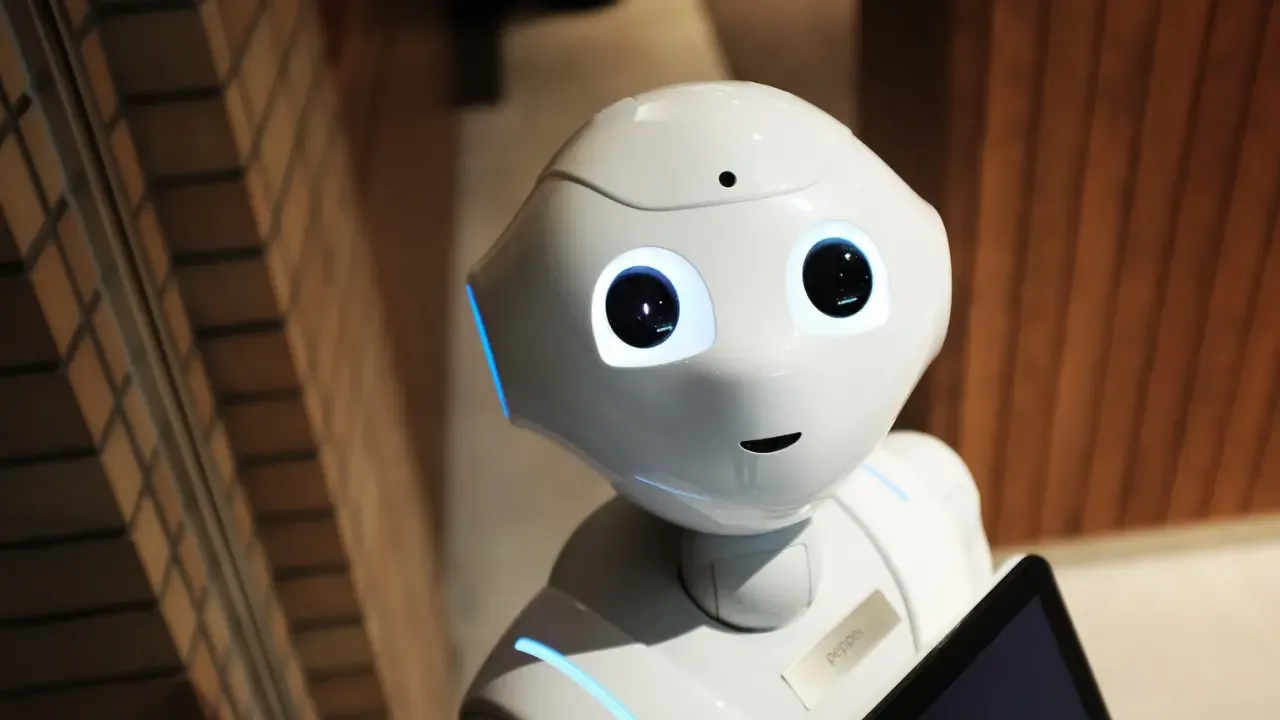
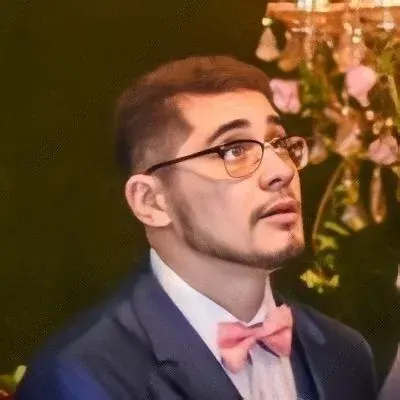
How to Use StringIO in Python 3: A Complete Guide 🐍💻
Are you struggling to import the StringIO
module in Python 3? 😫 Don't worry, we've got you covered! In this guide, we will address the common issues and provide easy solutions to help you use StringIO
effortlessly.
Problem
Here's a common problem faced by many Python developers:
import StringIO
ImportError: No module named 'StringIO'
When trying to import StringIO
, you might encounter this error message.
Solution
Python 3 introduced a new io
module as a replacement for Python 2's StringIO
module. To resolve the import error, simply use io.StringIO
instead:
import io
s = io.StringIO()
The Catch
Now that you can successfully import io.StringIO
, you might face another issue when using it with certain libraries, such as numpy
's genfromtxt
.
import io
import numpy
x = "1 3\n 4.5 8"
numpy.genfromtxt(io.StringIO(x))
This code snippet triggers the following error:
TypeError: Can't convert 'bytes' object to str implicitly
The Explanation
The genfromtxt
function expects a byte-like object, while io.StringIO
returns a string-like object by default. Hence the error message indicating the inability to implicitly convert the bytes
object.
The Solution (Part 2)
To successfully use genfromtxt
with io.StringIO
in Python 3, you need to explicitly encode the string-like object to bytes using the appropriate encoding, such as utf-8
. Here's the updated code:
import io
import numpy
x = "1 3\n 4.5 8"
numpy.genfromtxt(io.StringIO(x.encode('utf-8')))
This modification ensures that io.StringIO
returns a bytes
object, which is compatible with genfromtxt
.
Final Thoughts
Using StringIO
in Python 3 might present a few challenges due to the changes introduced by the io
module. However, by following the solutions provided in this guide, you can overcome these obstacles and leverage the power of string-like objects in your Python code.
If you found this guide helpful, don't forget to share it with your fellow Python enthusiasts! And feel free to leave a comment if you have any questions or faced any other issues related to Python development.
Happy coding! 🚀🐍💻