How to use filter, map, and reduce in Python 3
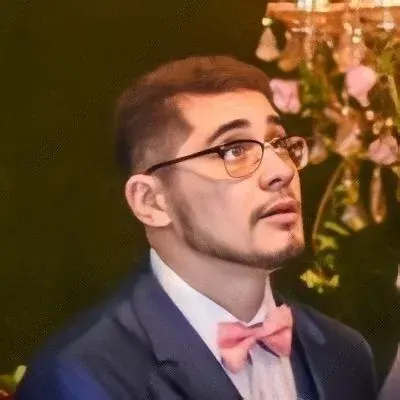
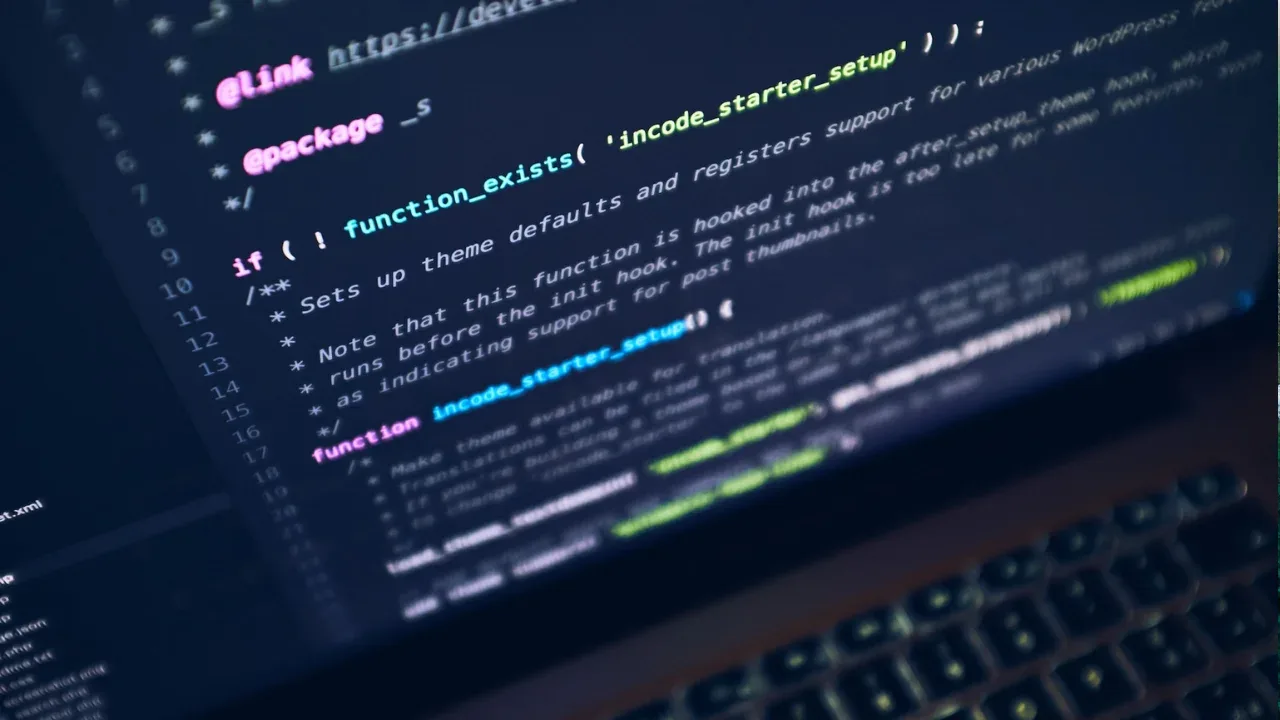
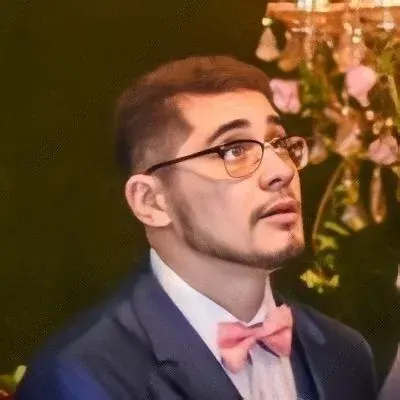
How to Use filter, map, and reduce in Python 3: A Complete Guide
Introduction 🐍
Python 3 has made some changes to the filter
, map
, and reduce
functions compared to Python 2. If you're accustomed to working with these functions in Python 2 and now finding them breaking in Python 3, you're not alone! In this guide, we will discuss the differences and provide easy solutions to make your Python 3 code work like the Python 2 code did.
Understanding the Differences 🔄
filter
Function:
In Python 2, the filter
function returned a list containing the filtered elements. However, in Python 3, filter
returns an iterable object called a filter object. To obtain a list, you can simply convert the filter object into a list using list()
.
# Python 2
filter(f, range(2, 25)) # Output: [5, 7, 11, 13, 17, 19, 23]
# Python 3
list(filter(f, range(2, 25))) # Output: [5, 7, 11, 13, 17, 19, 23]
map
Function:
Similar to filter
, the behavior of the map
function has changed in Python 3. In Python 2, map
returned a list, but in Python 3, it returns a map object, which is an iterable. You can also convert the map object into a list using list()
.
# Python 2
map(cube, range(1, 11)) # Output: [1, 8, 27, 64, 125, 216, 343, 512, 729, 1000]
# Python 3
list(map(cube, range(1, 11))) # Output: [1, 8, 27, 64, 125, 216, 343, 512, 729, 1000]
reduce
Function:
Python 3 has moved the reduce
function from the built-in namespace to the functools
module. Therefore, you need to import the reduce
function from functools
before using it.
# Python 2
reduce(add, range(1, 11)) # Output: 55
# Python 3
from functools import reduce
reduce(add, range(1, 11)) # Output: 55
Easy Solutions to Make Your Python 3 Code Work 🚀
To make your Python 3 code work like Python 2, you can follow these simple steps:
For the
filter
function, convert the filter object into a list usinglist()
.For the
map
function, convert the map object into a list usinglist()
.For the
reduce
function, import it from thefunctools
module before using it.
Conclusion 🏁
Python 3 introduced changes to the filter
, map
, and reduce
functions, which might lead to confusion if you're transitioning from Python 2. By understanding the differences and following the easy solutions provided, you can successfully use these functions with Python 3. So go ahead and start filtering, mapping, and reducing data with confidence in Python 3!
If you found this guide helpful, don't forget to share it with your Python enthusiast friends 🙌. If you have any questions or comments, feel free to reach out in the comments section below. Happy coding! 💻✨