How to use a variable inside a regular expression
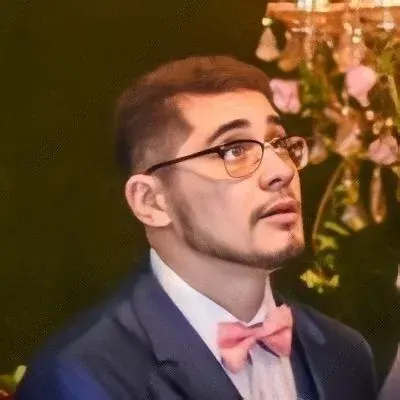
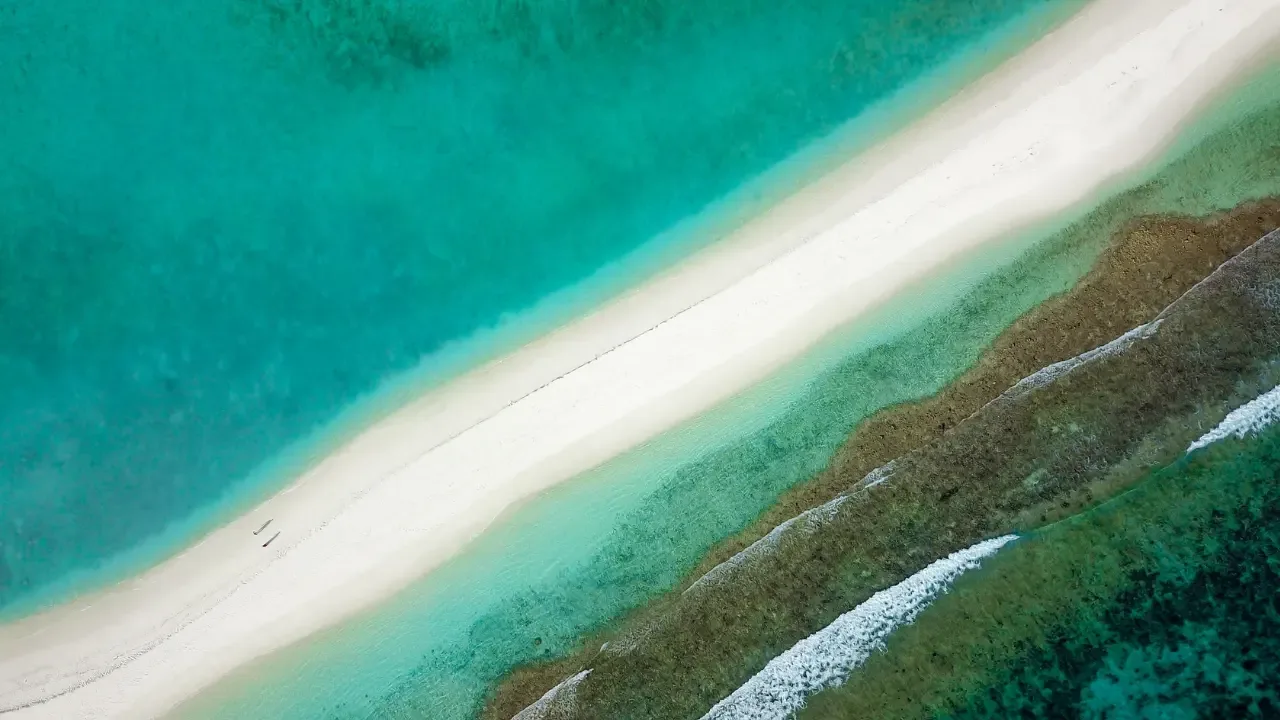
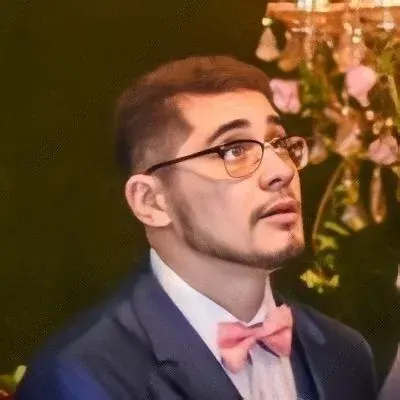
How to Use a Variable Inside a Regular Expression in Python 🐍
Regular expressions are powerful tools used for pattern matching and searching in strings. They allow you to define complex patterns and find matches within text. But what if you want to use a variable inside a regular expression? In this blog post, we'll explore how you can achieve that in Python.
The Problem 😕
Let's say you have a variable called TEXTO
and you want to use it as part of a regular expression pattern. In the example provided, the regular expression \b(?=\w)TEXTO\b(?!\w)
attempts to match the word TEXTO
surrounded by word boundaries. However, the code above treats TEXTO
as a literal string instead of a variable.
The Solution 💡
To use a variable inside a regular expression, you need to dynamically construct the regular expression pattern. One way to achieve this is by using f-strings, a feature introduced in Python 3.6 that allows for variable substitution within strings.
Here's an updated version of the code:
import re
import sys
TEXTO = sys.argv[1]
pattern = fr'\b(?=\w){TEXTO}\b(?!\w)'
if re.search(pattern, subject, re.IGNORECASE):
# Successful match
else:
# Match attempt failed
By prefixing the string with f
, we can include the TEXTO
variable directly in the regular expression pattern. The resulting pattern will be dynamically constructed at runtime, allowing for variable substitution.
Explanation 📝
The
fr
prefix before the string indicates an f-string, allowing for variable substitution.The
{}
curly braces are used to enclose the variableTEXTO
within the regular expression pattern.Word boundaries
\b
are included at the start and end of the pattern to ensure the word is matched as a whole.The
(?=\w)
positive lookahead and(?!\w)
negative lookahead are used to ensure the word is not part of a larger word.
Conclusion and Call-to-Action 👏
Using variables inside regular expressions in Python is a simple but powerful technique that can help you create more flexible and dynamic patterns. By leveraging f-strings and dynamically constructing the regular expression pattern, you can easily incorporate variables and solve the problem at hand.
Next time you find yourself needing to use a variable inside a regular expression in Python, remember this blog post and apply the techniques we've covered. Happy coding! 😄
Feel free to share your thoughts in the comments section below. Have you encountered any other issues with regular expressions? Let's discuss and learn from each other! ✨