How to terminate a python subprocess launched with shell=True
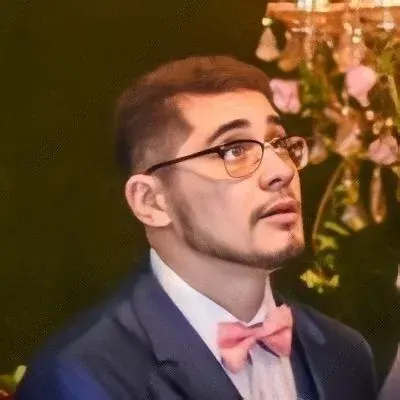
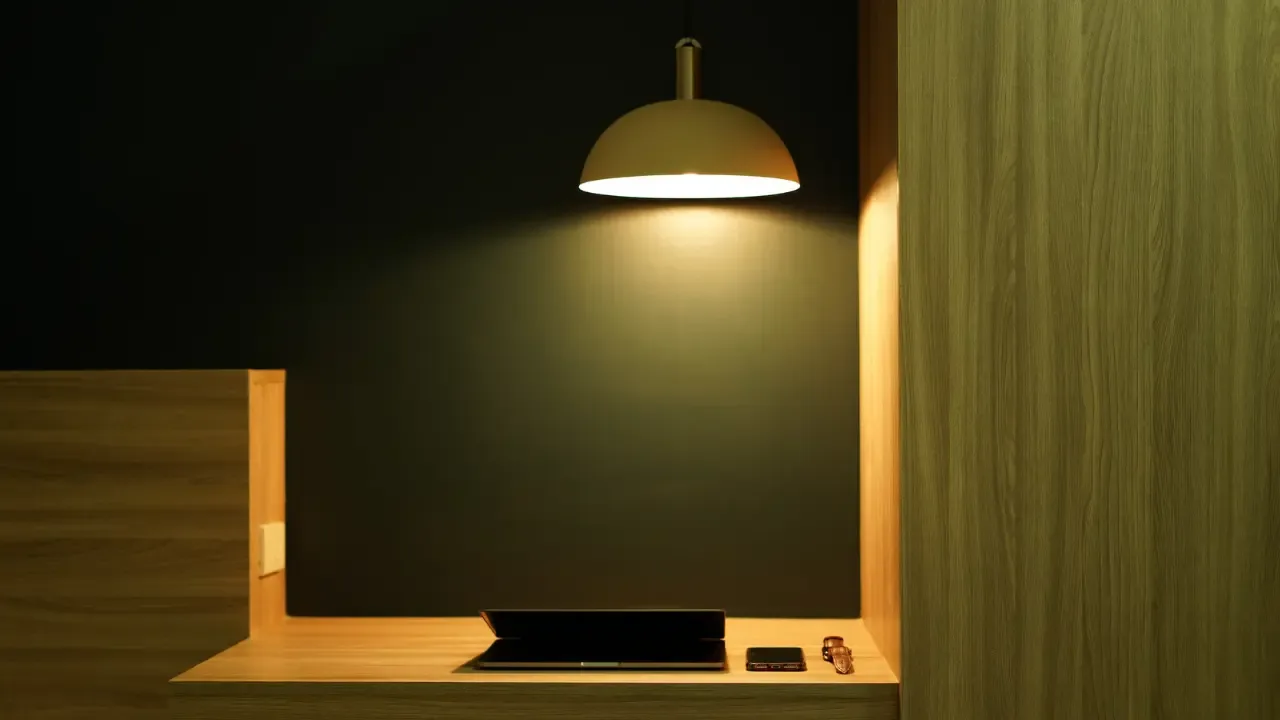
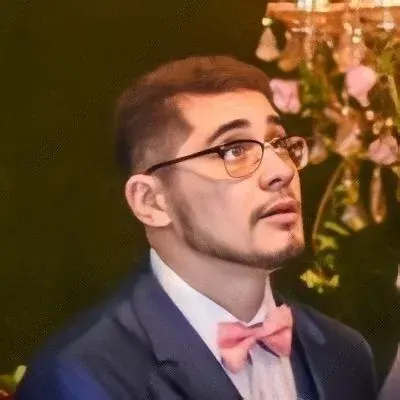
How to Terminate a Python Subprocess Launched with shell=True
So, you're trying to terminate a Python subprocess that was launched with shell=True
, but it keeps running in the background even when you try to use p.terminate()
or p.kill()
. Don't worry, you're not alone in facing this issue! Let's break it down and find an easy solution to terminate the process successfully.
The Problem Explained
When you use subprocess.Popen
with shell=True
, it launches a new shell process to execute your command. This can be useful when you need to execute complex shell commands or when you want to take advantage of shell features like shell variables or pipes.
However, terminating a subprocess launched with shell=True
can be a bit tricky. The p.terminate()
and p.kill()
methods may not work as expected in this case. This happens because terminate()
and kill()
only send the termination signal to the shell process, not to the actual command that was executed.
The Solution: Separating the Command and the Shell
To successfully terminate a subprocess launched with shell=True
, we need to separate the command from the shell. We can do this by splitting the command into a list of arguments and launching the subprocess without using the shell.
Here's an example of how you can modify your code to achieve this:
p = subprocess.Popen(cmd.split(), stdout=subprocess.PIPE)
By splitting the cmd
string into a list of arguments and launching the subprocess without the shell, you will be able to terminate the process using p.terminate()
or p.kill()
successfully.
Why Does it Work?
When you use shell=True
, the entire command is executed within a shell process. This means that sending termination signals like p.terminate()
or p.kill()
only affect the shell process itself, not the command running inside it.
However, when you separate the command from the shell by using cmd.split()
, the subprocess is launched directly without involving the shell. This allows termination signals to be directly sent to the command itself, resulting in successful termination.
Call-to-Action: Share Your Experience
Have you ever faced this issue when trying to terminate a Python subprocess launched with shell=True
? How did you solve it? Share your experiences and solutions in the comments below! Your insights might help others facing the same problem.
📢 Share your experience in the comments below and help others! 📢