How to take column-slices of dataframe in pandas
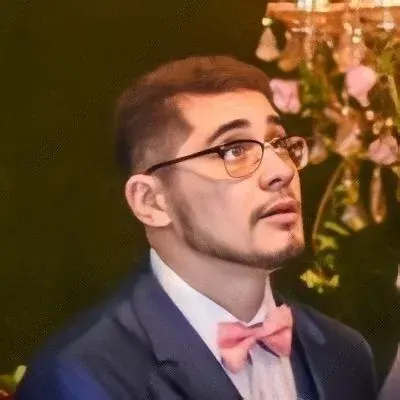
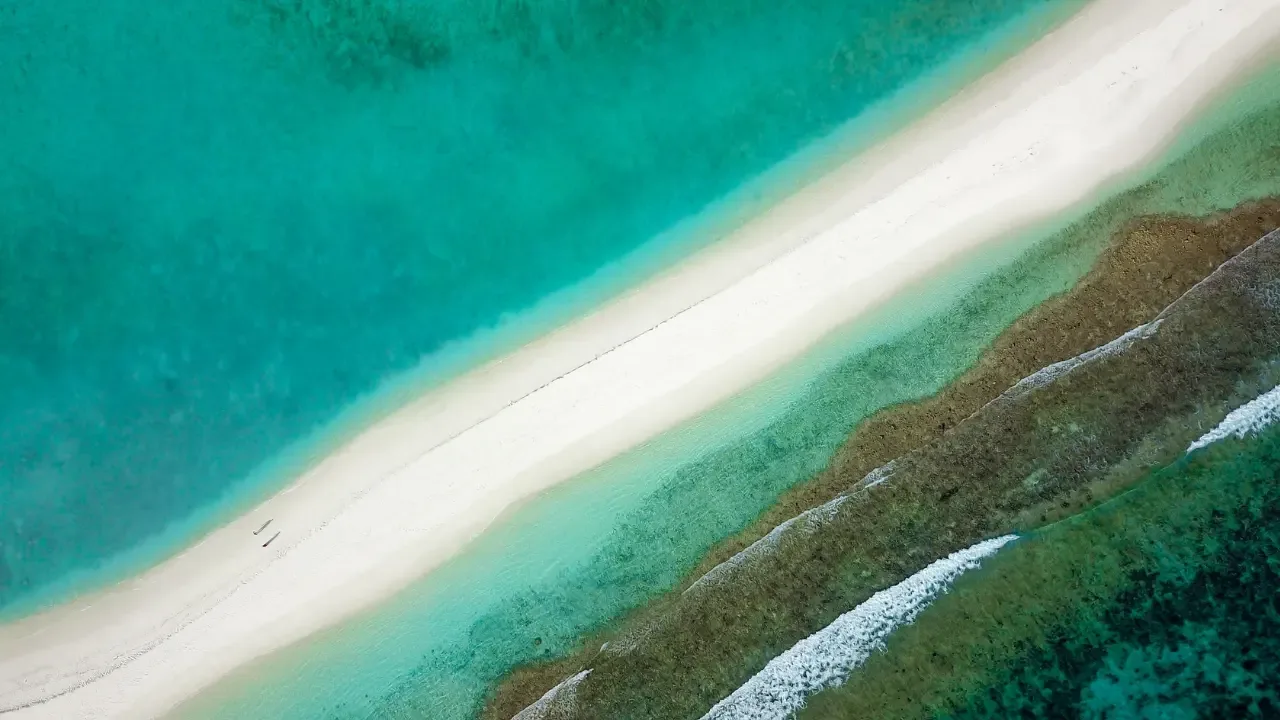
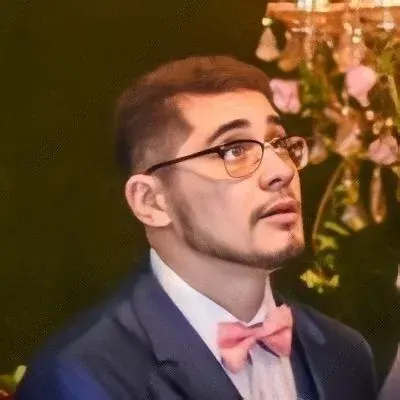
How to Take Column-Slices of DataFrame in Pandas
Are you struggling to slice your DataFrame in Pandas and extract specific columns? 🤔 Don't worry, you're not alone! Many pandas users find DataFrame indexing to be inconsistent and confusing.
In this blog post, we will address the common issue of slicing a DataFrame to extract column-slices. We will provide you with easy solutions and clear explanations to help you overcome this challenge. By the end of this post, you will be able to confidently extract the columns you need from your DataFrame. Let's dive in! 💪
The Problem
Let's start by setting the context. You have loaded machine learning data from a CSV file into a DataFrame. The first two columns represent observations, while the remaining columns represent features.
import pandas as pd
data = pd.read_csv('mydata.csv')
Your DataFrame, data
, looks something like this:
a b c d e
0 0.677564 0.564232 0.856879 0.438726 0.965432
1 0.123456 0.789012 0.345678 0.901234 0.567890
2 0.234567 0.890123 0.456789 0.123456 0.098765
3 0.987654 0.876543 0.654321 0.234567 0.543210
4 0.345678 0.456789 0.987654 0.345678 0.987654
5 0.654321 0.987654 0.234567 0.654321 0.420987
6 0.432109 0.345678 0.543210 0.654321 0.123456
7 0.876543 0.098765 0.012345 0.123456 0.876543
8 0.789012 0.234567 0.901234 0.012345 0.765432
9 0.567890 0.543210 0.678901 0.789012 0.234567
You want to slice this DataFrame into two separate DataFrames. The first DataFrame should contain columns a
and b
, and the second DataFrame should contain columns c
, d
, and e
.
The Solution
It might be tempting to use simple indexing to slice the DataFrame, but that won't work in this case. The key to successfully slicing columns in Pandas is to use the .loc
indexer.
To extract the columns a
and b
into a new DataFrame, you can use the following code:
observations = data.loc[:, 'a':'b']
Here, :
represents all rows, and 'a':'b'
represents the range of columns you want to extract. The resulting observations
DataFrame would look like this:
a b
0 0.677564 0.564232
1 0.123456 0.789012
2 0.234567 0.890123
3 0.987654 0.876543
4 0.345678 0.456789
5 0.654321 0.987654
6 0.432109 0.345678
7 0.876543 0.098765
8 0.789012 0.234567
9 0.567890 0.543210
Similarly, to extract columns c
, d
, and e
into another DataFrame, you can use the following code:
features = data.loc[:, 'c':'e']
The resulting features
DataFrame would look like this:
c d e
0 0.856879 0.438726 0.965432
1 0.345678 0.901234 0.567890
2 0.456789 0.123456 0.098765
3 0.654321 0.234567 0.543210
4 0.987654 0.345678 0.987654
5 0.234567 0.654321 0.420987
6 0.543210 0.654321 0.123456
7 0.012345 0.123456 0.876543
8 0.901234 0.012345 0.765432
9 0.678901 0.789012 0.234567
Understanding DataFrame Indexing
You might be wondering why Pandas' DataFrame indexing is a bit inconsistent. Columns can be indexed using labels, like data['a']
, but not by position, like data[0]
. On the other hand, slicing with data['a':]
is not allowed, but slicing with data[0:]
is permitted.
The reason behind this is to avoid ambiguity when indexing columns and rows. By allowing column indexing with labels and row indexing with positions, Pandas ensures that you can clearly refer to the data you need without confusion. For instance, data['a']
unambiguously refers to the column labeled 'a', whereas data[0]
could be interpreted as the first row or the first column.
Remember, when using .loc
to slice a DataFrame, both rows and columns are labeled. This consistent behavior avoids confusion and enhances the usability of Pandas.
Conclusion
Slicing columns in Pandas can be confusing, but with the right approach, it becomes straightforward. By using the .loc
indexer and specifying the range of columns, you can easily extract the column-slices you need from your DataFrame.
Next time you face the task of slicing a DataFrame, embrace this simple solution, and power up your data manipulation skills! 🔥
If you found this blog post helpful, feel free to share it with your fellow pandas enthusiasts and spread the knowledge. Also, let us know in the comments if you have any further questions or topics you'd like us to cover. Happy coding! 💻🐼