How to suppress or capture the output of subprocess.run()?
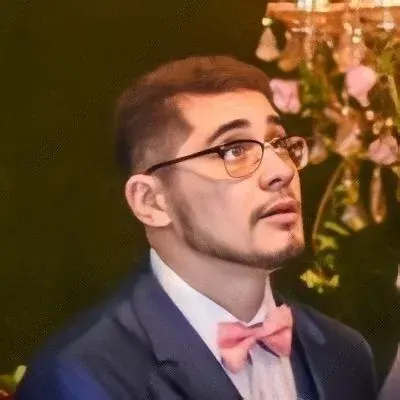
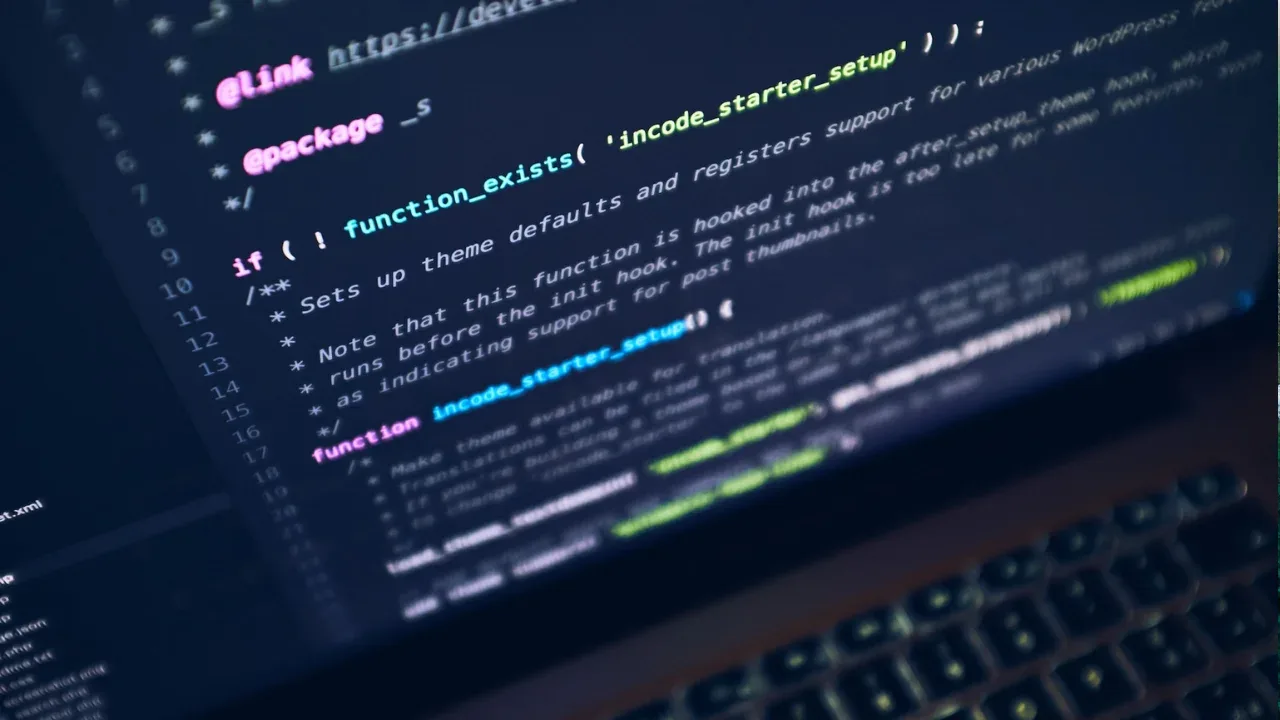
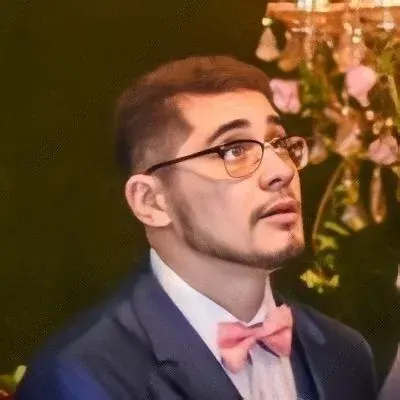
🔥📝🔥 How to suppress or capture the output of subprocess.run()?
Are you tired of seeing unwanted output cluttering your Python scripts when using subprocess.run()? 😩 Don't worry, I've got you covered! In this guide, we'll address the common issue of output suppression and show you easy solutions to capture or silence subprocess.run() output.
💡 Understanding the Default Behavior Many Python developers, just like you, have wondered why their code is producing output when the official documentation says otherwise. Let's clear the air and explain this mystery! 👻
As shown in the example provided, subprocess.run(["ls", "-l"]) is expected to suppress output. However, when tested in a Python shell, the listing is printed to the console. Why does this happen? 🤔
The explanation lies in how subprocess.run() handles output streams. By default, the output from the executed command is redirected to the parent process's standard output, hence the unexpected printing. But fear not, there are ways to tame this wild output, and we'll explore them now. 🐍
🔇 1. Silencing the Output If you want to suppress the output entirely, you can redirect it to the special file device called /dev/null. This device discards anything that is written to it, providing a silent execution. Let's update our example to demonstrate this: 🚀
import subprocess
subprocess.run(["ls", "-l"], stdout=subprocess.DEVNULL)
Now you can execute your command without any pesky output cluttering your workspace! 🙌🏼
📝 2. Capturing the Output Sometimes, we actually want to capture and utilize the output from subprocess.run(). The trick is to redirect the output to a variable rather than printing it directly. Here's how you can capture the output: 💥
import subprocess
result = subprocess.run(["ls", "-l"], capture_output=True, text=True)
print(result.stdout)
By setting the capture_output parameter to True and employing text=True for capturing text-based output, we can effectively capture the command's output. You can then manipulate and work with the captured output as needed. 🎉
🔗 Take Action and Level Up Your Python Skills! Now that you know how to tame the unruly output of subprocess.run(), it's time to put your new knowledge into practice! 🚀 Use the silencing or capturing techniques to optimize your Python scripts and command-line interactions. Don't let unwanted output interrupt your development flow! 💪
If you found this guide helpful or have any other questions or suggestions, feel free to leave a comment below. Let's engage in discussions and learn from each other's experiences! 🤝
Happy coding! 💻💡