How to split a dataframe string column into two columns?
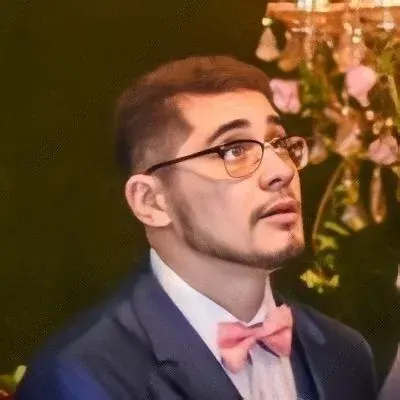
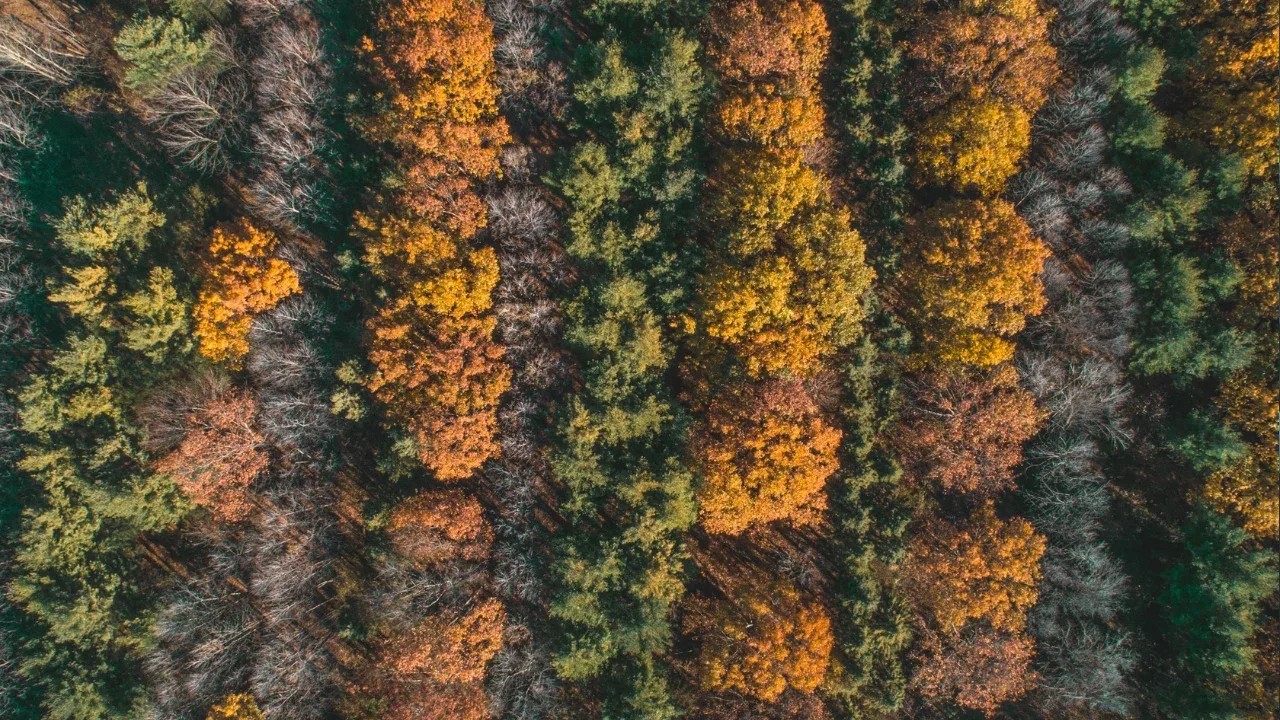
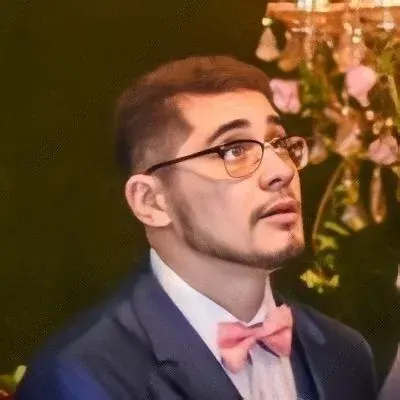
How to Split a Dataframe String Column into Two Columns?
So, you have a dataframe with a string column that you want to split into two separate columns. 🤔 Don't worry, we've got you covered! In this guide, we'll address this common issue and provide you with easy solutions to achieve your goal! 💪
The Problem: Splitting a String Column
Let's take a look at the context around this question. The dataframe, df
, has a single column called row
, which contains strings. The goal is to split the row
column into two columns: fips
and row
.
row
0 00000 UNITED STATES
1 01000 ALABAMA
2 01001 Autauga County, AL
3 01003 Baldwin County, AL
4 01005 Barbour County, AL
The user mentioned that they are aware of how to add a new column and populate it but are unsure about how to split the row
column.
The Solution: Using Pandas' String Manipulation Operations
To split the row
column into two, we can leverage the powerful string manipulation operations provided by the Pandas library. Specifically, we'll use the str
accessor and the str.split()
method.
Here's how you can achieve this:
df[['fips', 'row']] = df['row'].str.split(' ', 1, expand=True)
Let's break down this code:
df['row'].str.split(' ', 1, expand=True)
: This splits therow
column into two columns based on the space (' ') separator. The1
parameter specifies that the split should happen only once. Theexpand=True
argument ensures that the result is returned as a DataFrame.df[['fips', 'row']] = ...
: This assigns the result of the split operation to two new columns in the existing dataframe,fips
androw
.
After running this code, your dataframe will look like this:
fips row
0 00000 UNITED STATES
1 01000 ALABAMA
2 01001 Autauga County, AL
3 01003 Baldwin County, AL
4 01005 Barbour County, AL
🎉 Hooray! You have successfully split the row
column into two columns!
Wrap Up and Call-to-Action
In this blog post, we addressed the common problem of splitting a string column in a dataframe and provided you with an easy solution using Pandas' string manipulation operations. We hope you found this guide helpful in achieving your goal!
Now it's your turn to give it a try! Take a dataframe with a string column and see if you can split it into two columns using the techniques we discussed. If you encounter any issues or have other tech-related questions, feel free to leave a comment below. We'd love to hear from you and help you out!
Happy coding! 💻🔥