How to specify multiple return types using type-hints
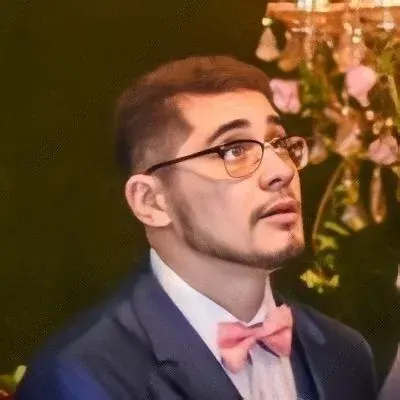
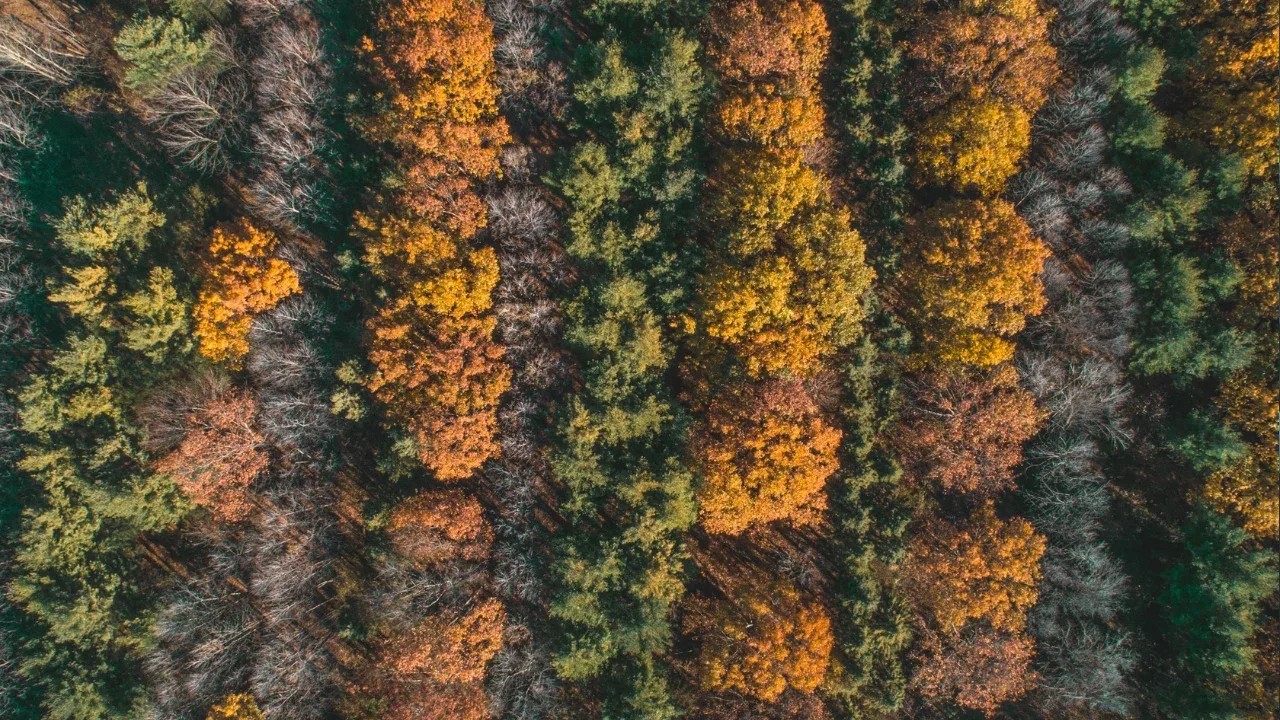
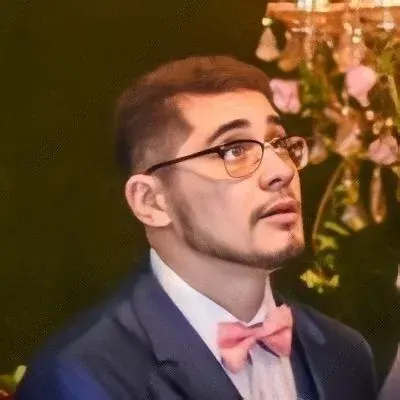
🎉 How to Specify Multiple Return Types Using Type Hints! 🎉
Have you ever found yourself in a situation where a function in your Python code might return different types of values? 🤔 Don't worry; you're not alone! It's a common challenge that many developers face. But fear not, because in this blog post, I'll show you how to use type hints to specify multiple return types and solve this problem once and for all! 💪
The Problem 💔
Let's imagine you have a function called foo
that can either return a bool
or a list
. How do you indicate this using type hints? 🤷♀️
def foo(id) -> list or bool:
...
Is the code snippet above the right way to do it? Sadly, it's not. 😓 Using the or
operator like that won't give you the desired result. But fret not! I'm here to show you the correct way to tackle this issue. Let's dive in! 🏊♀️
The Solutions 💡
1. Using Union Types 🤝
The most elegant way to specify multiple return types is to use the Union
type from the typing
module. It allows you to combine multiple types into one. Simply import it, and you're good to go! ✨
from typing import Union
def foo(id) -> Union[list, bool]:
...
By using Union[list, bool]
, you're telling Python that the function foo
can return either a list
or a bool
. 🙌 Problem solved! 🎉
2. Using Tuple Types 🎭
If you prefer to be more specific and know the exact order of the returned values, you can use tuple types. This approach is handy when you have fixed return types and need to maintain the order. 👌
For example, if your function always returns a list
followed by a bool
, you can use a tuple type like this:
def foo(id) -> Tuple[list, bool]:
...
See how easy it is? You can now specify the multiple return types with clarity! 👍
The Call-to-Action 📢
Now that you know how to specify multiple return types using type hints, don't let this newfound knowledge go to waste! 🚀 Go ahead and update your Python code to make it more readable and maintainable. Share this blog post with your fellow developers, and let's spread the love for clean code! ❤️
Wrapping Up 🎁
Type hints provide an excellent way to make your code more expressive and self-documenting. By specifying multiple return types, you can enhance readability and avoid confusion. Use the Union
type for a broader range of possible return types or tuple types for a more specific order.
Remember, clean code is not just about being aesthetically pleasing; it's about making your codebase easier to understand and maintain. 😊
I hope this guide helped you solve the mystery of specifying multiple return types using type hints. If you have any questions or want to share your thoughts, leave a comment below. Happy coding! 🙌🚀