How to sort mongodb with pymongo
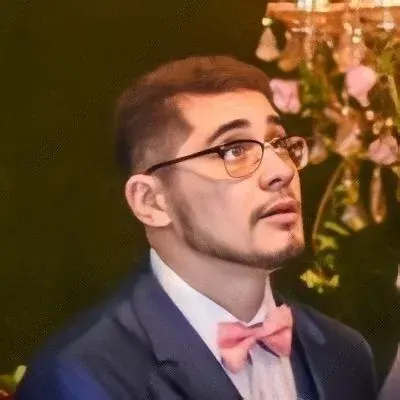
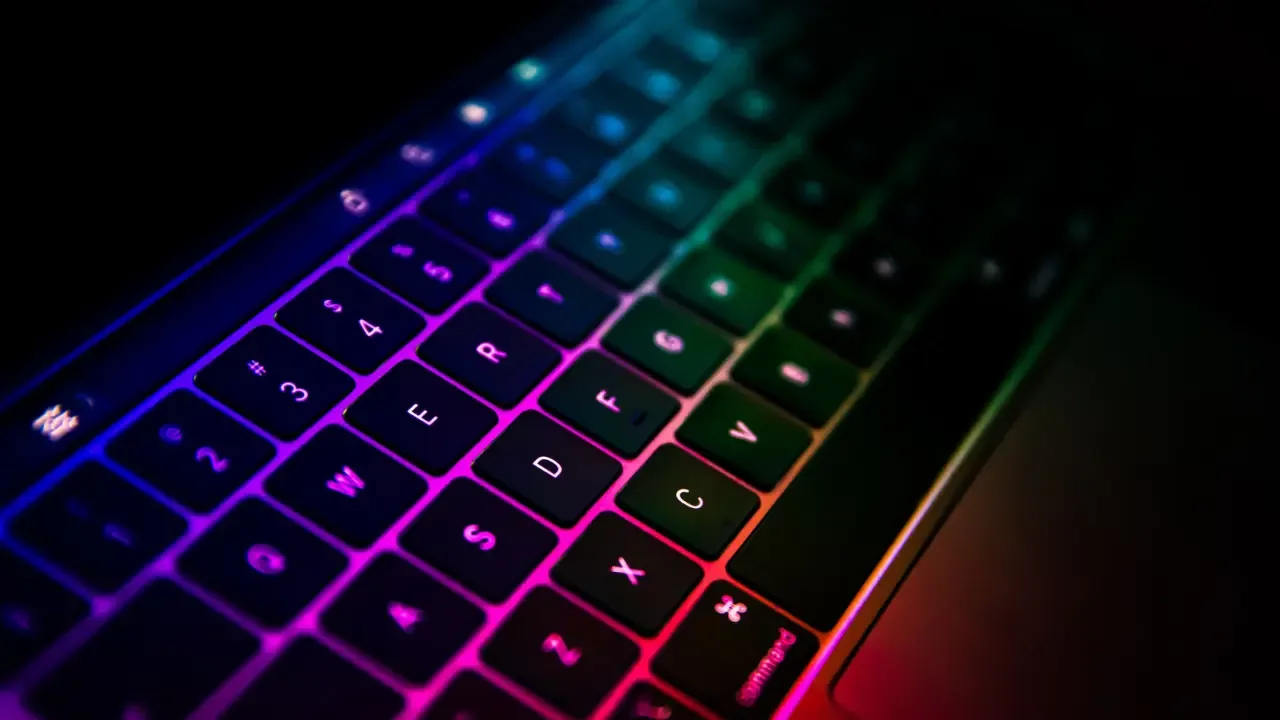
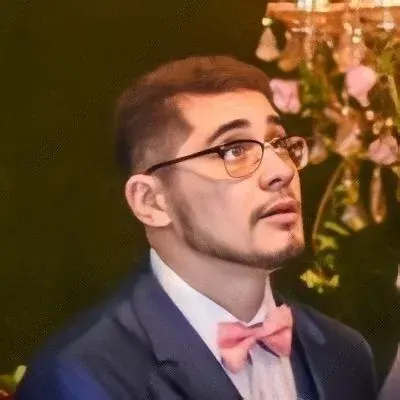
How to Sort MongoDB with PyMongo: A Simple Guide
Are you struggling to sort your MongoDB data using PyMongo? You're not alone! Sorting data in MongoDB can sometimes be a bit tricky, especially when using PyMongo. But fret not, because we're here to help you solve this problem and get you on your way to sorting your data like a pro!
The Problem
You're trying to use the sort()
feature in PyMongo to sort your MongoDB data, but you're facing some issues. 🤔 The same query works perfectly fine in the MongoDB console, but it's failing when you try it in your PyMongo code. Let's dive into the issue and find a solution.
The Code
Here's the code that's causing the problem:
import pymongo
from pymongo import Connection
connection = Connection()
db = connection.myDB
print db.posts.count()
for post in db.posts.find({}, {'entities.user_mentions.screen_name':1}).sort({u'entities.user_mentions.screen_name':1}):
print post
The Error
You're seeing the following error message:
Traceback (most recent call last):
File "find_ow.py", line 7, in <module>
for post in db.posts.find({}, {'entities.user_mentions.screen_name':1}).sort({'entities.user_mentions.screen_name':1},1):
File "/Library/Python/2.6/site-packages/pymongo-2.0.1-py2.6-macosx-10.6-universal.egg/pymongo/cursor.py", line 430, in sort
File "/Library/Python/2.6/site-packages/pymongo-2.0.1-py2.6-macosx-10.6-universal.egg/pymongo/helpers.py", line 67, in _index_document
TypeError: first item in each key pair must be a string
The Solution
The error message is indicating that the first item in each key pair must be a string. To fix this issue, you need to pass the sorting key as a dictionary with strings as keys. Here's the corrected code:
import pymongo
from pymongo import MongoClient
client = MongoClient()
db = client.myDB
# Count the number of posts
print db.posts.count()
# Sort and iterate over the posts
for post in db.posts.find({}, {'entities.user_mentions.screen_name': 1}).sort('entities.user_mentions.screen_name', 1):
print post
In the corrected code, we changed the way we pass the sorting key to the sort()
method. Instead of using {u'entities.user_mentions.screen_name':1}
, we use 'entities.user_mentions.screen_name'
directly as a string.
By making this small modification, you'll be able to sort your MongoDB data seamlessly using PyMongo.
📣 Share Your Success!
We hope this guide helped you sort your MongoDB data successfully using PyMongo. If it did, we'd love to hear about your experience! Share your success story or any other issues you faced by leaving a comment below. Let's help each other and make our developer community stronger! 💪😃
So go ahead, give it a try, and let us know how it works for you. Happy coding! 🚀