How to sort a list of dictionaries by a value of the dictionary in Python?
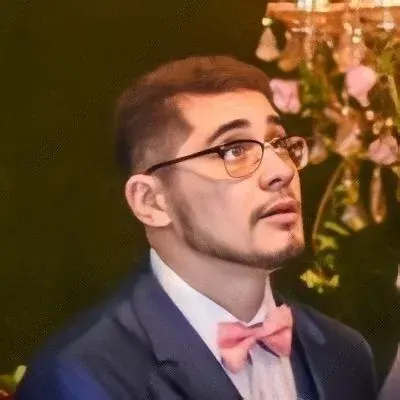
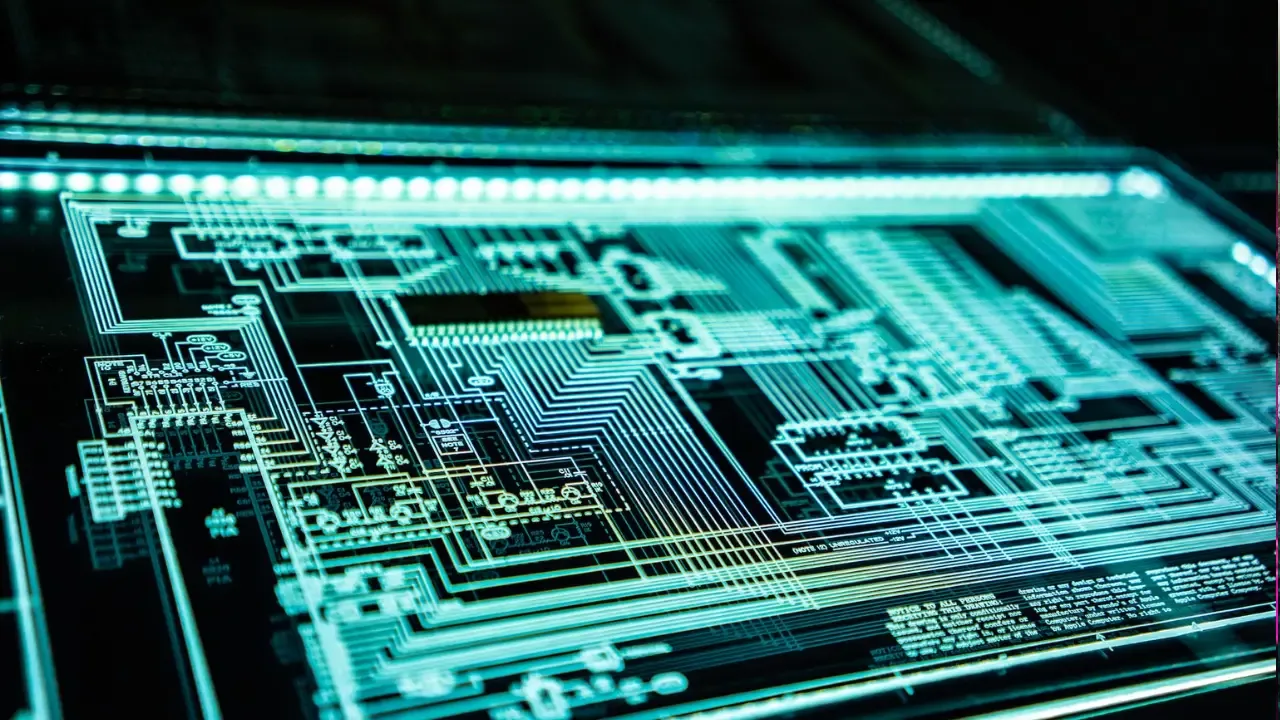
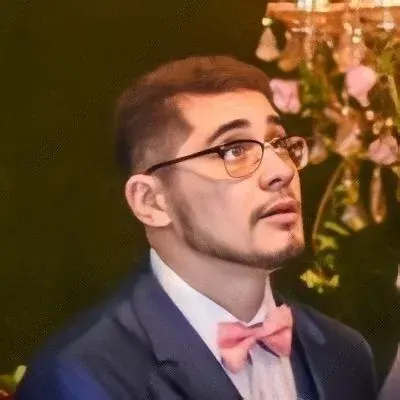
How to Sort a List of Dictionaries by a Value in Python 🐍
Are you struggling to sort a list of dictionaries by a specific value in Python? Sorting can be challenging, especially when you're dealing with complex data structures like dictionaries. But worry not! In this blog post, I'll guide you through the process step by step, providing easy solutions and examples along the way. So let's dive in and sort those dictionaries like a pro! 💪
The Problem 🤔
Consider the following list of dictionaries:
[{'name': 'Homer', 'age': 39}, {'name': 'Bart', 'age': 10}]
You want to sort this list of dictionaries by the 'name'
key, resulting in the following desired output:
[{'name': 'Bart', 'age': 10}, {'name': 'Homer', 'age': 39}]
The Solution 🎉
To achieve this, we'll use Python's built-in sorted()
function along with a lambda
function to specify the key we want to sort by. Here's the code:
sorted_list = sorted(list_of_dicts, key=lambda x: x['name'])
Let's break down what's happening here:
We use the
sorted()
function to sort thelist_of_dicts
based on the'name'
key.The
key
parameter takes a lambda function that extracts the'name'
value from each dictionary (x
) in the list and returns it.The
sorted()
function then uses these extracted values to sort the list.
And just like that, you have a sorted list of dictionaries! 🎊
Example 💡
Let's see the solution in action with a full example:
list_of_dicts = [{'name': 'Homer', 'age': 39}, {'name': 'Bart', 'age': 10}]
sorted_list = sorted(list_of_dicts, key=lambda x: x['name'])
print(sorted_list)
Output:
[{'name': 'Bart', 'age': 10}, {'name': 'Homer', 'age': 39}]
We successfully sorted the list of dictionaries by the 'name'
key!
Conclusion 🌟
Sorting a list of dictionaries in Python doesn't have to be a daunting task. By leveraging the power of the sorted()
function and a lambda function, you can easily sort a list based on any key in the dictionary. Now that you have this knowledge, go ahead and sort your dictionaries like a boss! 💪
I hope you found this guide helpful. If you have any questions or alternative solutions, feel free to leave a comment below. Happy coding! 😊
Action Time! 👇 Share your experience with sorting lists of dictionaries in Python. Have you encountered any challenges or found any cool tricks? Let's start a conversation in the comments section! 🎉