How to show all columns" names on a large pandas dataframe?
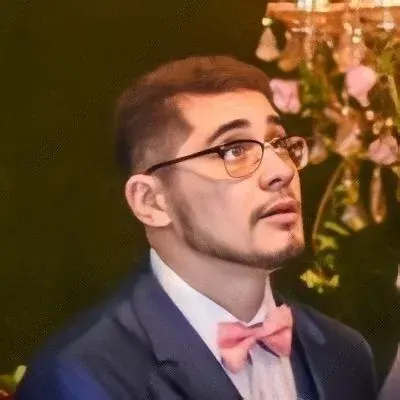
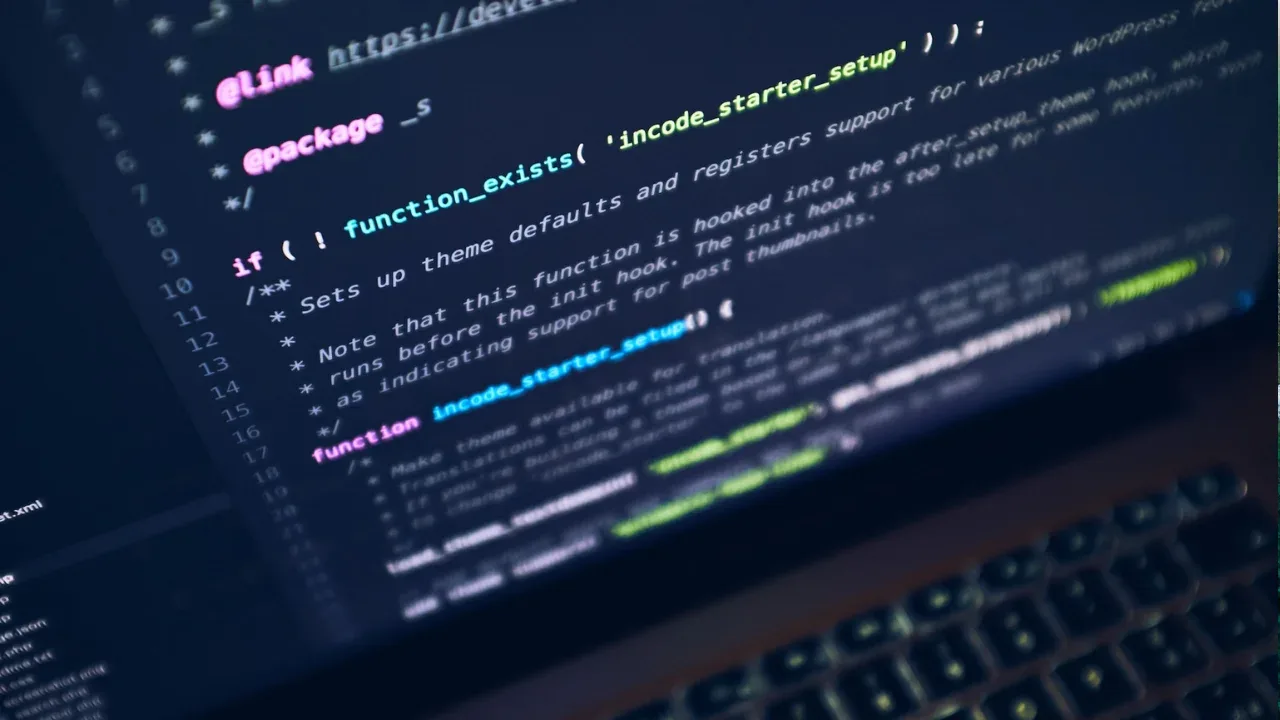
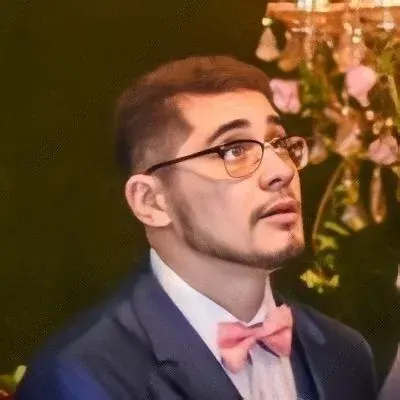
📝 Tech Blog: How to Show All Columns' Names on a Large Pandas Dataframe
Are you dealing with a massive dataframe containing hundreds of columns, and you're having trouble seeing all the column names? Don't worry, we've got you covered! In this guide, we'll walk you through easy solutions to this seemingly tricky problem. Let's dive in! 🚀
The Problem 🤔
You have a dataframe with an overwhelming number of columns, and the default behavior of Pandas is to truncate the list of column names. This can be frustrating when you need to see all the columns for analysis or debugging purposes. But fear not, there are a few simple ways to overcome this limitation. 💪
Solution 1: Use .to_string()
Method 📜
One way to show all column names is by using the .to_string()
method on the dataframe's columns
attribute. Here's how you can do it:
print(df.columns.to_string())
This will display all the column names in a single, comprehensive list. Easy peasy, right? 😎
Solution 2: Set Pandas Display Options ⚙️
If you want to make showing all column names your default behavior in Pandas, you can tweak the display options. By modifying the pd.options.display.max_columns
value, you can decide how many columns get displayed. If you set it to None
, all columns will be shown. Here's an example:
import pandas as pd
pd.options.display.max_columns = None
# Your dataframe code here
With this configuration, Pandas will display all column names, no matter how extensive the dataframe is. 💡
Solution 3: Use the .columns.tolist()
Method 📋
Another straightforward method is to convert the columns
attribute to a list using the .tolist()
method. Here's how you can do it:
column_list = df.columns.tolist()
print(column_list)
This will print out all the column names in a single, easily digestible list. Voila! 🎉
Conclusion and Call-to-Action 👏
Dealing with a large dataframe and needing to display all column names can be a daunting task. However, with these easy solutions at your disposal, you can effortlessly overcome this obstacle and get back to conquering your data analysis challenges. Have fun exploring your dataframe to uncover valuable insights!
Did you find these solutions helpful? Have any other tech questions or topics you'd like us to cover? Let us know in the comments section below! We're always excited to hear from our readers. 📣