How to set class attribute with await in __init__
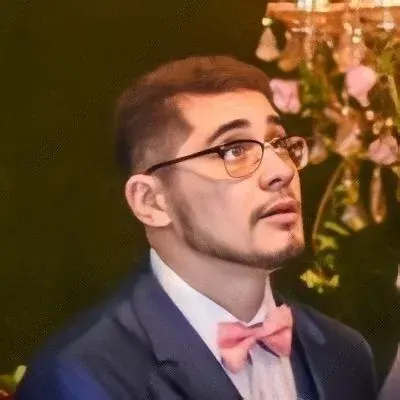
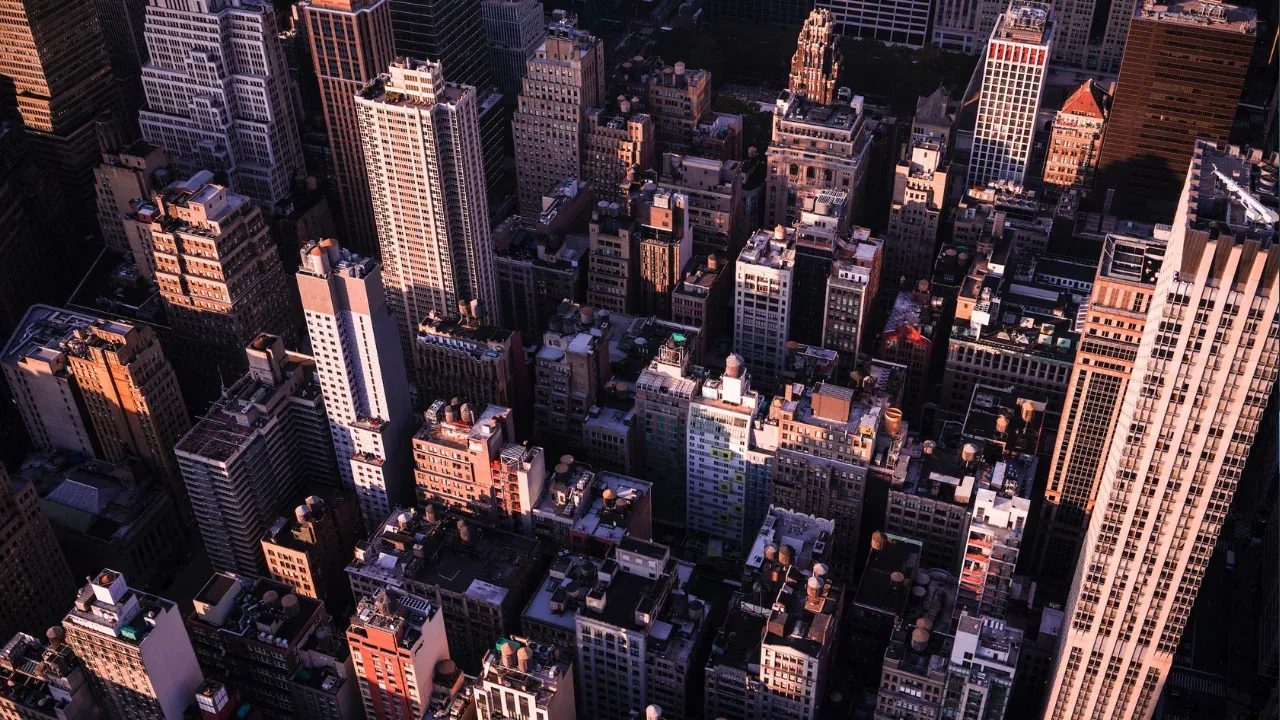
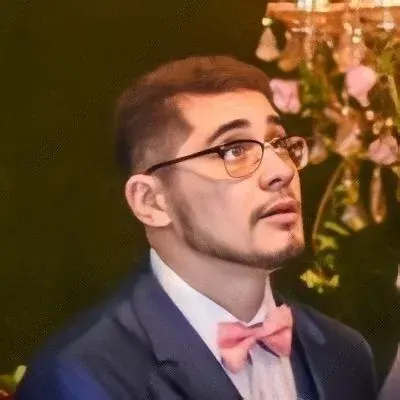
šļø Title: "Setting Class Attributes with Await in init Made Easy"
š Introduction:
Hey there, tech enthusiasts! If you've ever wondered how to set class attributes using the await
keyword in the __init__
method or class body, you've come to the right place. In this blog post, we'll address this common issue and provide you with easy solutions to help you tackle this problem like a pro. So let's dive right in!
š” The Problem:
Many developers face a stumbling block when they try to define a class with await
in the constructor or class body. They find themselves encountering the dreaded TypeError: __init__() should return None, not 'coroutine'
or syntax errors, leaving them scratching their heads.
š Understanding the Issue: To shed some light on the problem, consider the example below:
import asyncio
class Foo(object):
async def __init__(self, settings):
self.settings = settings
self.pool = await create_pool(dsn)
foo = Foo(settings) # Raises TypeError
In this case, the await
keyword in the __init__
method causes a TypeError
because the __init__
method is expected to return None
, not a coroutine.
Another situation is when you attempt to use await
in a class body:
class Foo(object):
self.pool = await create_pool(dsn) # Raises syntax Error
def __init__(self, settings):
self.settings = settings
foo = Foo(settings)
This code snippet triggers a syntax error because you cannot use await
outside an async function or method.
š” Easy Solution: To overcome these challenges, you can follow this simple approach:
class Foo(object):
def __init__(self, settings):
self.settings = settings
async def init(self):
self.pool = await create_pool(dsn)
foo = Foo(settings)
await foo.init()
Here, we define a separate async method called init()
that initializes the class attribute self.pool
using await create_pool(dsn)
. By calling await foo.init()
, we ensure the create_pool()
coroutine gets executed within an async context. This allows us to use await
without violating any syntax or causing type errors.
š£ Engage with Us:
We hope this guide helps you overcome the obstacle of setting class attributes with await
in the __init__
method or class body. If you have any other questions or alternative solutions, feel free to share them in the comments below. Let's learn and grow together!
Happy coding! šš©āš»šØāš»
PS: Don't forget to share this post with your friends who might find it useful!