How to serialize SqlAlchemy result to JSON?
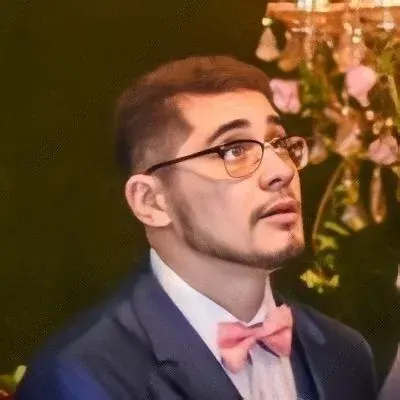
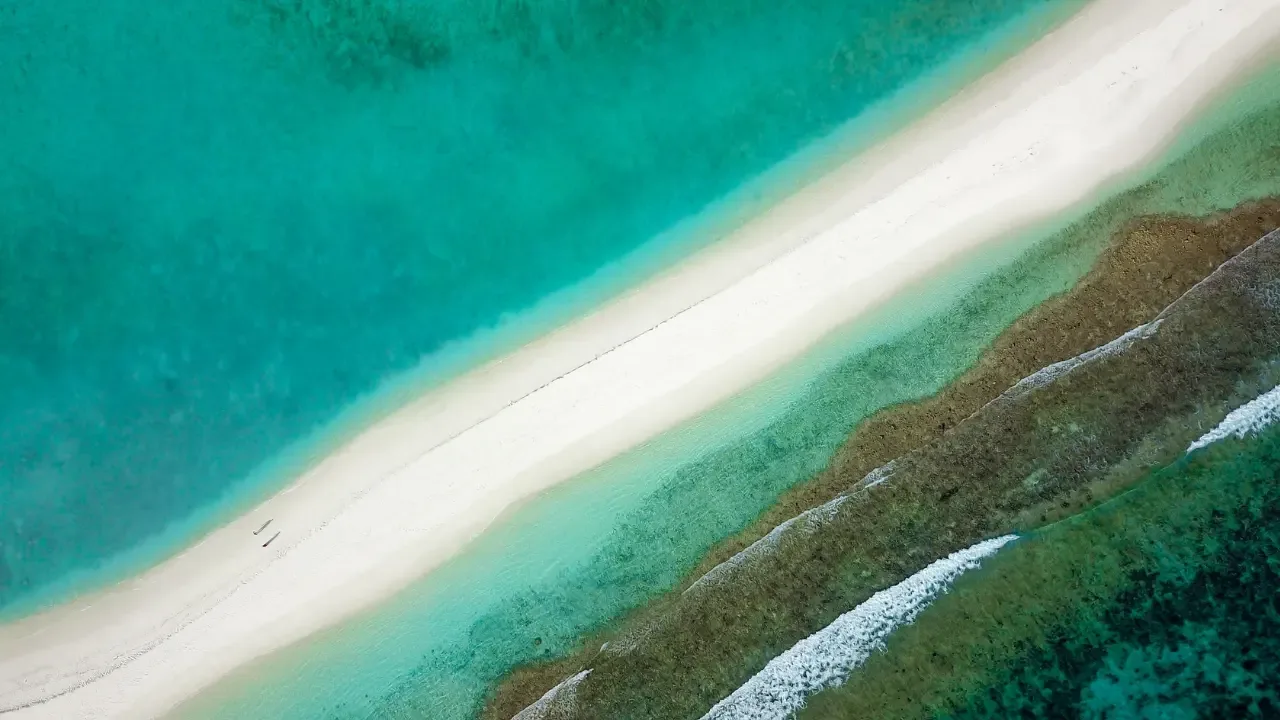
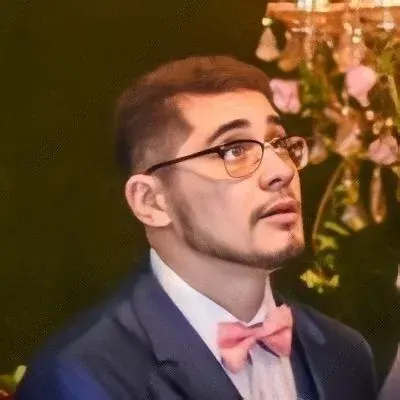
How to Serialize SqlAlchemy Result to JSON? ππΎπ
If you've ever worked with SqlAlchemy, you might have found yourself in a situation where you need to serialize the query result into JSON format. Unfortunately, SqlAlchemy doesn't provide a built-in JSON serializer for its ORM objects. But fret not! In this blog post, we will explore some easy solutions to tackle this problem. Let's dive right in! πͺπΌ
The Challenge π€
When attempting to serialize a SqlAlchemy query result using well-known methods like jsonpickle.encode
or json.dumps
, you may encounter the dreaded TypeError: <Product('3', 'some name', 'some desc')> is not JSON serializable
error. This occurs because these methods struggle to serialize the actual SqlAlchemy ORM objects directly into JSON. π±
Solution 1: Custom Serializer π§ͺ
One way to overcome this challenge is by implementing a custom serializer. Let's take a look at an example:
def serialize(obj):
if isinstance(obj.__class__, DeclarativeMeta):
# Handle SqlAlchemy ORM objects
return obj.__dict__
# Handle other non-serializable types if needed
return None
The serialize
function above detects if the object is an ORM object and returns its __dict__
, which represents the object's attributes in a dictionary format. You can then use this function while serializing the query result using json.dumps
:
import json
# Assuming 'items' is the result of a SqlAlchemy query
serialized_items = json.dumps(items, default=serialize)
Using this approach, you can now successfully serialize the query result to JSON!
Solution 2: Custom Mixin π³
Another approach is to utilize a custom mixin class that extends the functionality of SqlAlchemy's base model. Here's an example:
class JsonSerializableMixin(object):
def to_json(self):
return {column.name: getattr(self, column.name) for column in self.__table__.columns}
The to_json
method above creates a dictionary mapping the column names to their corresponding values in the ORM object. You can then call this method on your ORM object to get the JSON representation:
# Assuming 'item' is a single ORM object
json_item = item.to_json()
This way, you can convert individual objects to JSON easily.
Bring on the JSON! π
With these solutions in your arsenal, you can effortlessly serialize your SqlAlchemy query results into JSON format. Whether you opt for a custom serializer or a mixin class, the choice ultimately depends on your project requirements.
So go ahead, serialize those objects like a pro and unleash the power of JSON in your data-driven applications! π
If you have any other tips, tricks, or alternative methods for serializing SqlAlchemy results to JSON, please share them in the comments below. Let's keep the conversation going! π¬β¨