How to see the raw SQL queries Django is running?
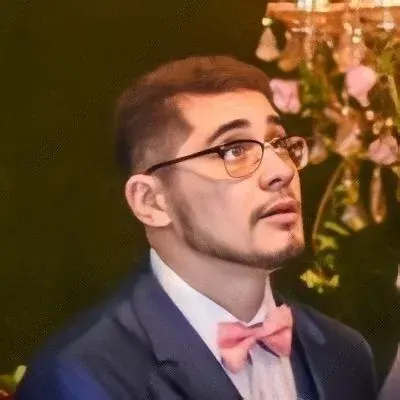
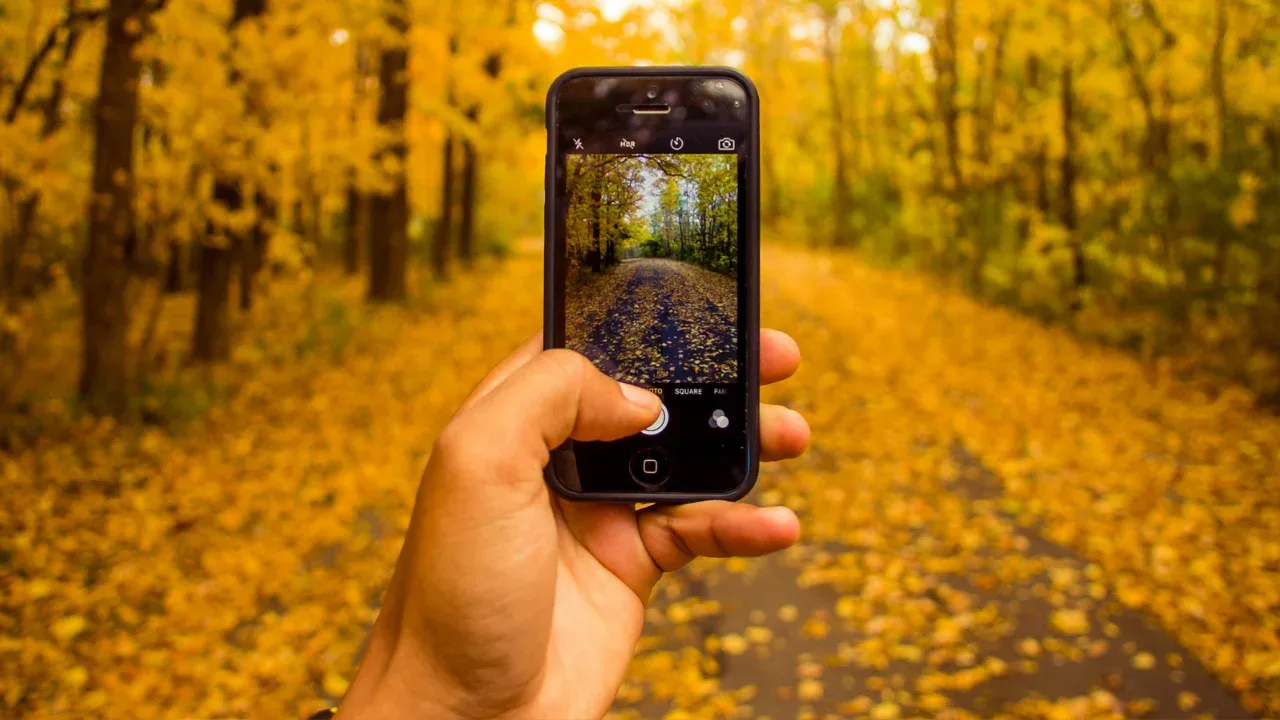
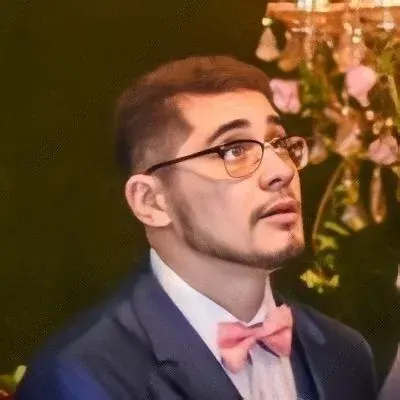
🕵️♀️ Revealing Django's Hidden Secrets: Unleashing the Raw SQL Queries 💻
Are you tired of wondering what really goes on behind the scenes when Django performs a database query? 🕵️♀️ Do you wish to dive into the depths of SQL to gain a better understanding of your application's performance? 🔍 Well, you're in luck! In this guide, we'll unveil the secret to exposing Django's raw SQL queries, empowering you with insights that will take your debugging game to a whole new level! 🚀
The Quest for Raw SQL Queries
<p>Is there a way to show the SQL that Django is running while performing a query?</p>
🔍 Understanding the Challenge
Django, being an abstracted framework, shields developers from the complexities of raw SQL queries by providing its own query API. While this offers convenience and boosts productivity, it can sometimes leave us clueless about the actual SQL statements executed.
🎯 The Solution: Logging to the Rescue!
To uncover Django's hidden SQL queries, we can tap into Django's logging capabilities. Django provides a powerful logging framework that can be configured to record various levels of debug information, including SQL queries.
1️⃣ Step 1: Configuring Logging
First, we need to configure logging in Django's settings. Open your settings.py file and add or modify the logging configuration:
LOGGING = {
'version': 1,
'disable_existing_loggers': False,
'loggers': {
'django.db.backends': {
'level': 'DEBUG',
'handlers': ['console'], # Customize your handlers as needed
},
},
}
2️⃣ Step 2: Enable Debug Mode
In order for the above logging configuration to take effect, ensure that DEBUG
mode is set to True
in your settings.py file:
DEBUG = True
3️⃣ Step 3: Witness the Magic!
With the logging configuration in place, Django will now print the raw SQL queries in the console as they are executed. Execute your Django code, and behold the SQL revelations! 🔮
Let's Put It to the Test
To ensure our newfound powers are working correctly, let's consider an example. Suppose we have a Django model called Employee
, and we want to retrieve all employees whose salary is above a certain threshold:
>>> employees = Employee.objects.filter(salary__gt=50000)
When we execute this code snippet, Django will generate the corresponding SQL query according to the defined filters, and now, thanks to our logging configuration, it will also print the raw SQL query in the console. Resulting in something like this:
[DEBUG] (0.012) SELECT "app_employee"."id", "app_employee"."name", "app_employee"."salary" FROM "app_employee" WHERE "app_employee"."salary" > 50000
Easy, right? We can now understand the SQL queries happening behind the scenes!
💡 Pro Tips
Remember to revert the
DEBUG
mode toFalse
in your production environment to avoid any sensitive information being exposed.Use this technique sparingly for troubleshooting or optimizing specific queries, as excessive logging can impact performance.
📣 Take It to the Next Level
Congratulations, you've conquered the art of unraveling Django's raw SQL queries! ✨ But don't stop here! Experiment with different queries, explore complex joins, and uncover the true potential of your database interactions. Share your newfound wisdom with fellow developers, and remember, the power of knowledge lies in sharing! 👥💬
So go ahead, unleash the hidden SQL queries within Django, and let the debugging adventure begin! Happy Pythoning! 🐍💻
PS: Are you a Django pro who knows some tips and tricks about optimizing database queries? Share your insights in the comments below! Let's exchange knowledge and level up our coding game! 🚀🔥
📢 Did you find this guide helpful? Share it with your friends! 🌟 And don't forget to subscribe to our newsletter 📧 to receive more exciting Django tips and tricks straight to your inbox!