How to search and replace text in a file?
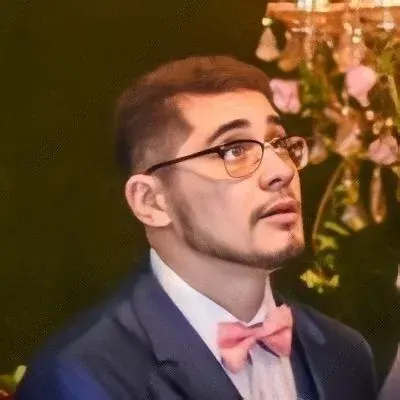
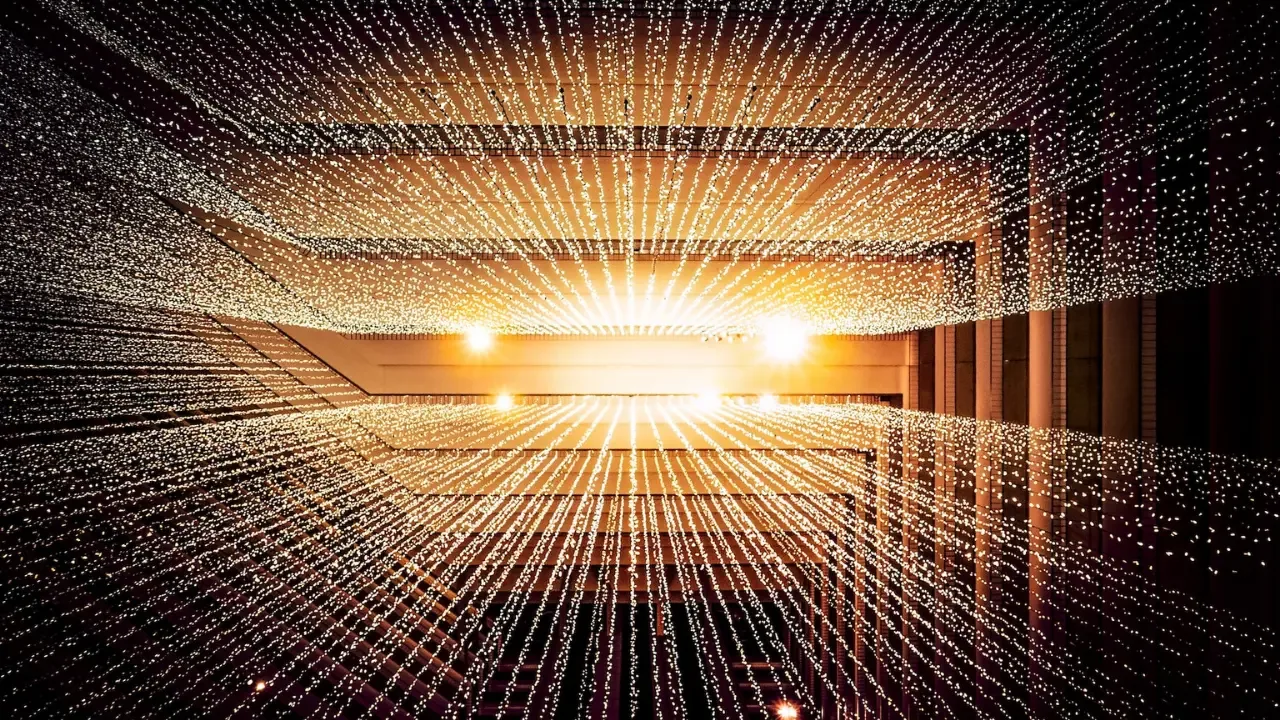
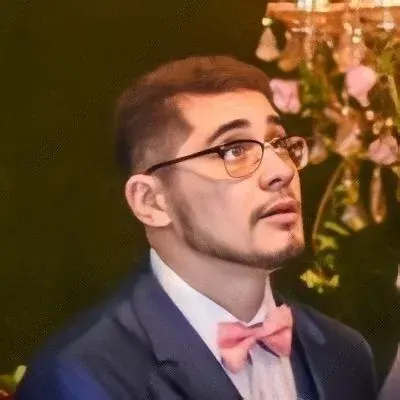
📝 Tech Blog: Easy Search and Replace in Python
Are you tired of manually searching and replacing text in your files? Don't worry, I've got you covered! In this blog post, I will guide you through the process of searching and replacing text in a file using Python 3. 🐍
🔍 Understanding the Problem
Let's start by understanding the problem at hand. You want to search for a specific text in a file and replace it with another. The code snippet you provided seems to do the trick, but there seems to be a small issue when replacing specific text combinations.
👾 Identifying the Issue
In the given example, when replacing 'abcd' with 'ram', some unwanted characters are left at the end of the line. This can be quite frustrating, as it doesn't give us the desired result. But worry not, there's a simple solution for this!
✨ The Easy Solution
To fix this issue, we need to make a slight modification to the code. Instead of using the write()
method directly on the file, we will write the modified text into a temporary file. Then, we will replace the original file with the temporary file, effectively performing the search and replace.
Here's the updated code:
import os
import fileinput
print("Text to search for:")
text_to_search = input("> ")
print("Text to replace it with:")
text_to_replace = input("> ")
print("File to perform Search-Replace on:")
file_to_search = input("> ")
temp_file = open(file_to_search + '.temp', 'w')
for line in fileinput.input(file_to_search):
temp_file.write(line.replace(text_to_search, text_to_replace))
temp_file.close()
os.replace(file_to_search + '.temp', file_to_search)
input('\n\nPress Enter to exit...')
🌟 Example and Explanation
To better understand how this solution works, let's go through an example. Suppose we have the following input file:
hi this is abcd hi this is abcd
This is a dummy text file.
This is how search and replace works abcd
And we want to replace all occurrences of 'abcd' with 'ram'. Here's what the updated code will do:
It opens the input file and creates a temporary file to write the modified text.
For each line in the input file, it replaces all occurrences of 'abcd' with 'ram' and writes the modified line to the temporary file.
Once all lines are processed, it closes the temporary file and replaces the original file with the temporary file.
Finally, it asks for user input to keep the console window open before exiting.
The result will be:
hi this is ram hi this is ram
This is a dummy text file.
This is how search and replace works ram
✅ Problem Solved!
By using this modified approach, you can now easily search and replace text in a file without any junk characters or unexpected issues. 🎉
💡 Take Action
Now that you know the solution, why not try it out yourself? Pick a file, choose some text to search for and replace, and see the magic happen! Don't forget to share your success story or any other issues you encounter in the comments below. Let's learn and grow together!
📣 Join the Discussion
Have you faced any other challenges while working with file manipulation in Python? What other coding problems would you like to see addressed in future blog posts? Share your thoughts and suggestions in the comments section!
Happy coding! 💻