How to reversibly store and load a Pandas dataframe to/from disk
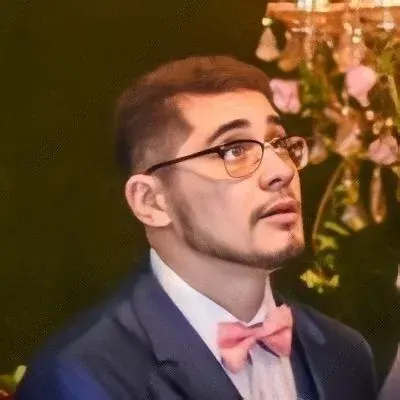
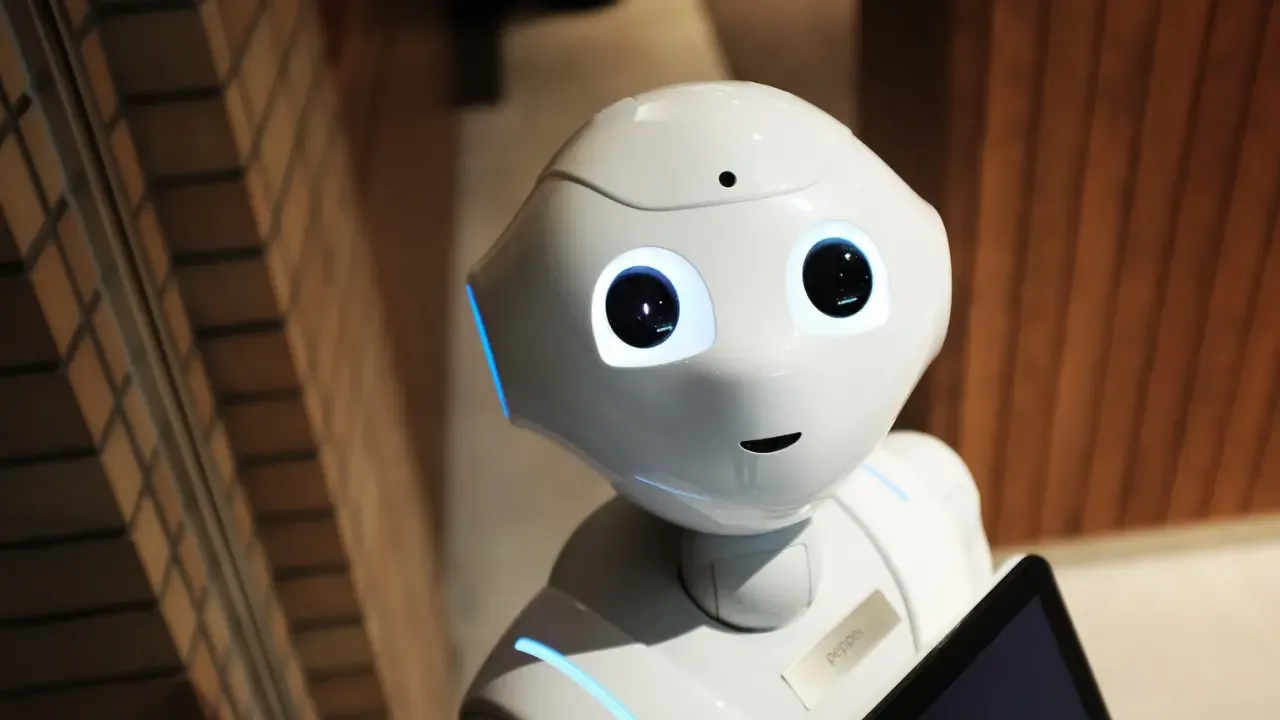
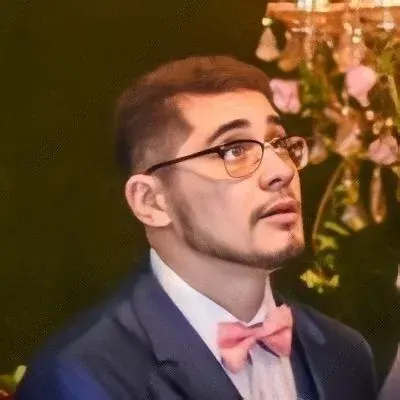
📊 How to Save and Load a Pandas Dataframe Reversibly to/from Disk 📊
Are you tired of waiting for your script to run every time you need to import a large CSV file as a Pandas dataframe? 😫 Well, fret no more! In this blog post, we'll explore a solution to keep that valuable dataframe available between runs, saving you precious time ⏳ and effort. Let's dive right in!
📥 Loading the DataFrame from Disk
To avoid the time-consuming process of importing your CSV over and over again, we can save the dataframe to disk after the initial import. Then, we can load it quickly on subsequent runs. Here's how you can achieve this using Pandas:
import pandas as pd
# Load the CSV file into a dataframe
df = pd.read_csv('path/to/your/csv')
# Save the dataframe as a pickle file
df.to_pickle('path/to/your/file.pkl')
By using to_pickle
, we serialize the dataframe and save it as a binary file on disk. This file can be loaded back into a dataframe with ease, as we'll see in the next section!
📤 Reloading the DataFrame from Disk
Once your dataframe is saved in a pickle file, you can load it swiftly into memory whenever you need it. Here's how:
import pandas as pd
# Load the dataframe from the pickle file
df = pd.read_pickle('path/to/your/file.pkl')
Super easy, right? By using read_pickle
, we deserialize the pickle file and obtain the dataframe in its original form. This method avoids the time-consuming CSV import process, allowing you to work with your data right away!
⚖️ The Benefits and Caveats
The ability to reversibly store and load a Pandas dataframe brings numerous advantages. Let's take a closer look at some of them:
✅ Time and Effort Savings: By avoiding expensive CSV imports, you save valuable time and effort, improving the efficiency of your workflow.
✅ Consistency: Since you're always working with the same dataframe, you ensure consistency in your data analysis or machine learning tasks.
✅ Version Control: The pickle file serves as a snapshot of your dataframe at a specific point in time. You can track changes, compare versions, and easily roll back if needed.
However, it's essential to be aware of a caveat when using this approach:
❗ Potential Compatibility Issues: Pickle files created with one version of Pandas might not be compatible with a different version. Make sure to use the same Pandas version when loading a pickle file to avoid any potential compatibility problems.
To mitigate these issues, keep track of the Pandas version you used to create the pickle files and ensure you have the same version installed when loading them.
📣 Your Turn!
You're now equipped with the knowledge to save and load a Pandas dataframe seamlessly. Apply this technique to your projects, and enjoy the benefits of faster data loading and consistency in your analyses. Share your success stories, show off your code, and let us know how this method has boosted your productivity!
Do you have any other data-related questions? We're here to help! Leave a comment below and let's start a vibrant discussion. Happy coding! 💻💡
Psst... Don't forget to follow us on Twitter and subscribe to our newsletter for more exciting tech tips and tricks!