How to replace NaNs by preceding or next values in pandas DataFrame?
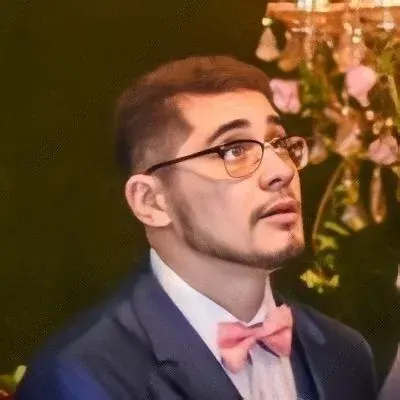
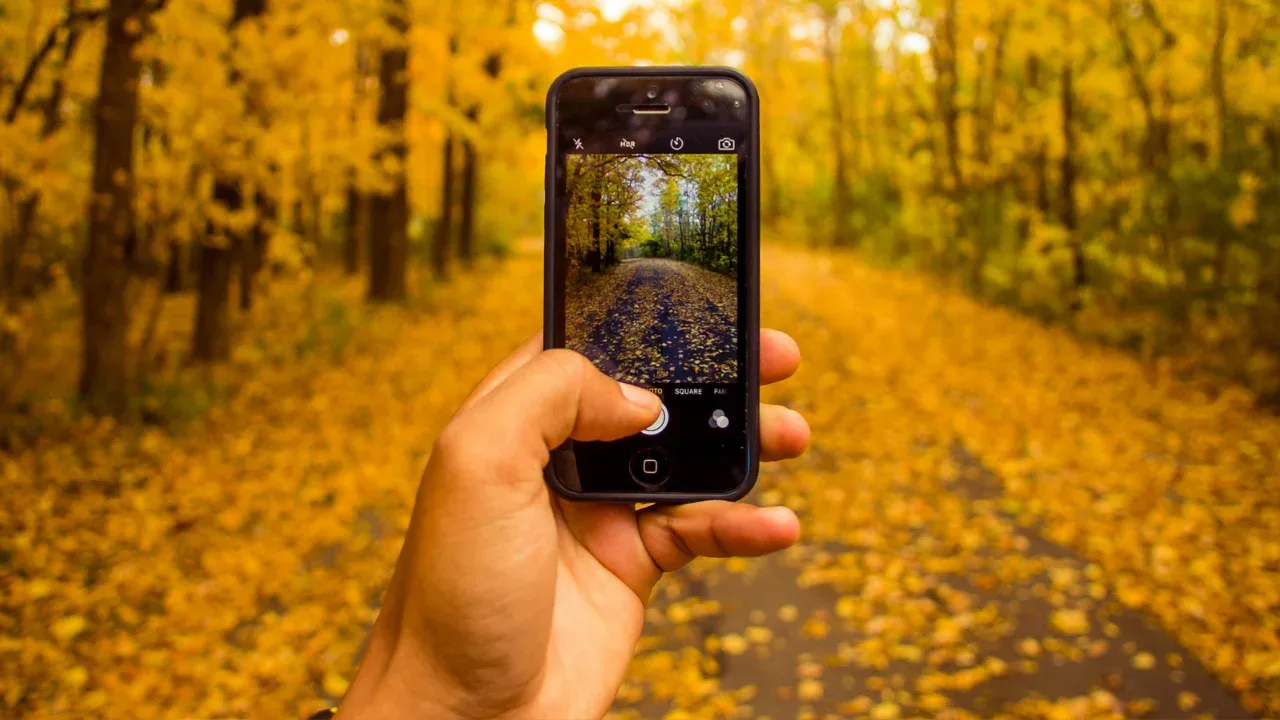
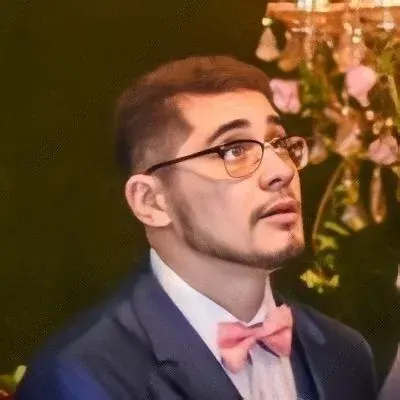
How to Replace NaNs by Preceding or Next Values in Pandas DataFrame? 💭📚
Dealing with missing values, represented as NaN (Not a Number), is a common challenge when working with data. In pandas, a popular Python library for data manipulation and analysis, we have various options to handle these missing values. One common scenario is replacing NaNs with either the preceding or next non-NaN value in the same column.
In this blog post, we will explore an easy and efficient approach to replace NaNs in a pandas DataFrame using built-in methods. We will consider the context provided, where the first row is guaranteed to never contain NaNs.
Understanding the Problem and Expected Output 📝💡
Let's start by examining the provided data. We have a DataFrame with some NaNs, as shown below:
import pandas as pd
df = pd.DataFrame([[1, 2, 3], [4, None, None], [None, None, 9]])
The DataFrame looks like this:
0 1 2
0 1 2 3
1 4 NaN NaN
2 NaN NaN 9
The goal is to replace each NaN with the first non-NaN value that appears above it in the same column. Following this logic, the expected output for the given example would be:
0 1 2
0 1 2 3
1 4 2 3
2 4 2 9
Replace NaNs with Forward Fill (ffill) Method 🔄🆙
To achieve our desired outcome, pandas provides the ffill
method, which stands for forward fill. This method fills missing values with the preceding non-NaN value along the specified axis (by default, it works column-wise). Let's see how we can utilize it to replace NaNs by preceding values:
df_filled = df.ffill()
The resulting DataFrame, df_filled
, would look like this:
0 1 2
0 1 2 3
1 4 2 3
2 4 2 9
With just one line of code, we have replaced all the NaNs with the preceding non-NaN values in each column.
Replace NaNs with Backward Fill (bfill) Method 🔄🔙
Similarly, if we want to replace NaNs with the next non-NaN value in each column, we can use the bfill
method, which stands for backward fill. This method fills missing values with the next non-NaN value along the specified axis. Here's an example:
df_filled = df.bfill()
The resulting DataFrame, df_filled
, would be:
0 1 2
0 1 2 3
1 4 9 9
2 9 9 9
As we can see, the backfilled approach replaces each NaN with the next non-NaN value in the same column.
Combining Forward Fill and Backward Fill for Optimal NaN Replacement 🔄🔃
In some cases, utilizing both forward fill and backward fill can provide a more comprehensive NaN replacement. By employing the ffill
method first and then applying the bfill
method, we can account for both preceding and next non-NaN values. Here's how we can achieve this:
df_filled = df.ffill().bfill()
The resulting DataFrame, df_filled
, would be:
0 1 2
0 1 2 3
1 4 2 3
2 4 2 9
By combining the two methods, we have successfully replaced all the NaNs in the DataFrame with the closest non-NaN values.
Conclusion and Call-to-Action 🎉📢
Dealing with missing values is a crucial aspect of data cleaning and preprocessing. In this blog post, we have explored an easy and efficient way to replace NaNs by either the preceding or next non-NaN values in a pandas DataFrame. By utilizing the ffill
and bfill
methods, we were able to achieve the desired results without writing complex loops.
Remember, understanding different techniques for handling missing values in pandas can greatly enhance your data analysis and modeling workflows.
So go ahead and give it a try! Replace NaNs in your own DataFrames and see how it impacts your analysis. Share your experience and any cool tips in the comments below. Happy coding! 😄💻