How to remove an element from a list by index
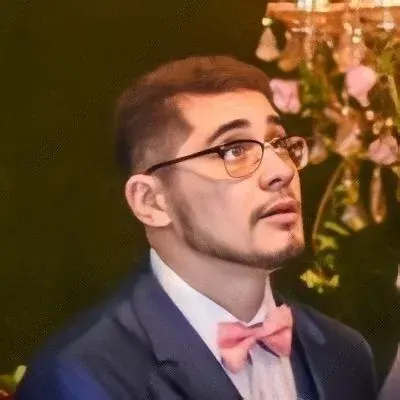
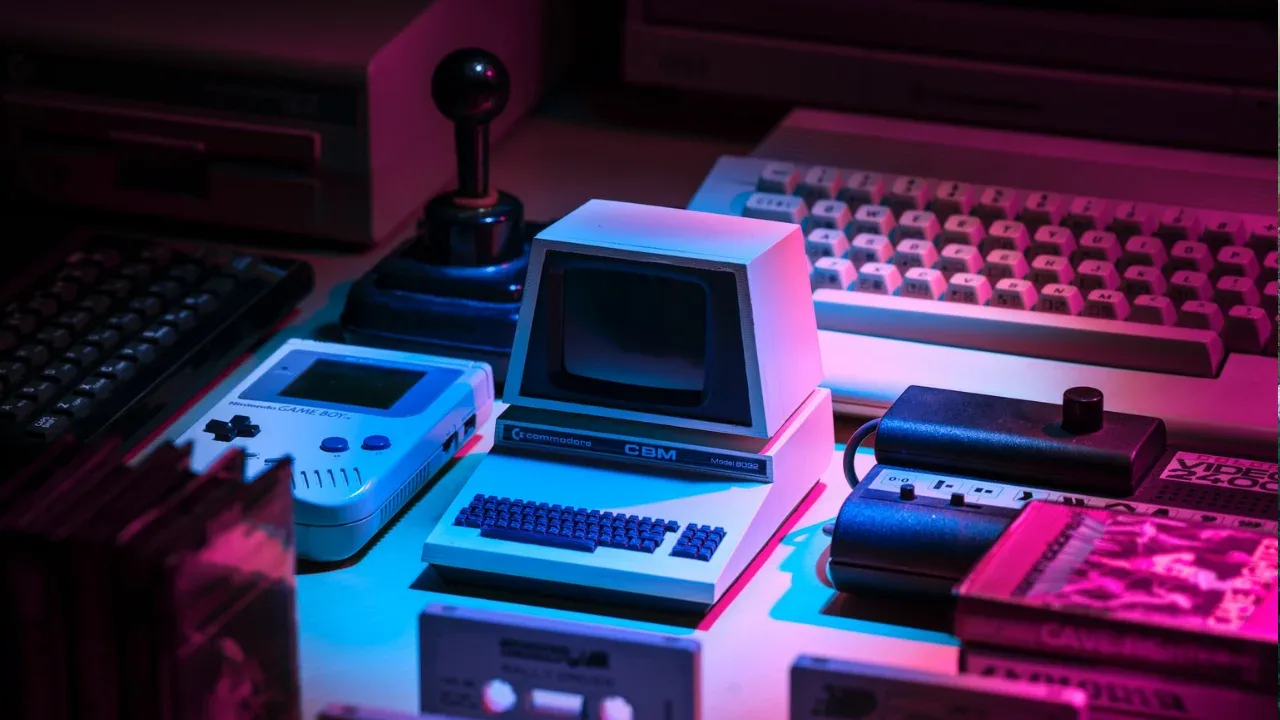
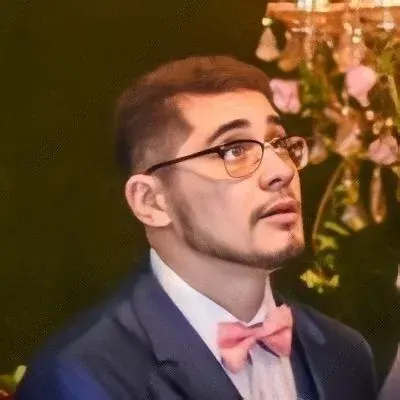
🚀 The Ultimate Guide to Removing Elements from a List by Index 📝
So, you want to remove an element from a list, but not just any element – you want to remove it by index. You've probably stumbled upon the list.remove()
method, but quickly realized it only works to remove elements by value. Don't worry, I got you covered! In this guide, we'll explore some easy solutions to remove elements by index from a list. Let's dive in! 💪
Common Issues 🤔
Before we jump into the solutions, let's quickly talk about some common issues you might encounter when trying to remove an element by index.
1. Index out of range ❌
The first problem you might face is trying to remove an element at an index that doesn't exist in the list. For example, if your list has 5 elements and you try to remove an element at index 10, you'll encounter an "index out of range" error. We'll provide a solution to handle this scenario later.
2. Multiple occurrences of the same element 🔄
Another issue arises when you have multiple occurrences of the same element in your list. If you remove an element by index without considering duplicates, you might unintentionally remove the wrong element. We'll address this concern and show you how to handle it properly.
Easy Solutions 🎉
Now let's get down to business and explore some easy solutions to remove elements from a list by index.
1. Using the del
statement ❌
The simplest way to remove an element from a list by index is by using the del
statement. Here's an example:
my_list = [1, 2, 3, 4, 5]
index_to_remove = 2
del my_list[index_to_remove]
In this example, we use del
followed by the name of the list and the index of the element we want to remove. Keep in mind that using del
will modify the original list directly, so be cautious!
2. Creating a function for safe index removal ✅
To address the first issue mentioned earlier (index out of range), it's a good practice to create a function that handles index removal gracefully. Here's an example:
def remove_by_index(lst, index):
if index >= 0 and index < len(lst):
del lst[index]
return lst
else:
print("Invalid index!")
In this function, we check if the provided index is within the valid range of the list. If it is, we remove the element using del
and return the modified list. Otherwise, we display an error message.
3. Removing all occurrences of an element with multiple duplicates ♻️
If you're dealing with a list containing duplicate elements and you want to remove all occurrences of a particular element by index, you can use a list comprehension. Let's take a look at an example:
my_list = ['a', 'b', 'a', 'c', 'a']
element_to_remove = 'a'
my_list = [item for index, item in enumerate(my_list) if item != element_to_remove or index == my_list.index(element_to_remove)]
In this example, we use a list comprehension to iterate over the list, excluding the elements that match the one we want to remove. Additionally, we include an exception for the first occurrence of the element, ensuring it remains in the list.
Take Action! 📢
Now that you know how to remove elements from a list by index, it's time to put your knowledge into practice! Challenge yourself to implement these solutions in your own code and see the results.
If you found this guide helpful, share it with your friends and fellow developers. Don't hesitate to leave a comment below sharing your thoughts or asking any further questions. Happy coding! 😄👍