How to read and write INI file with Python3?
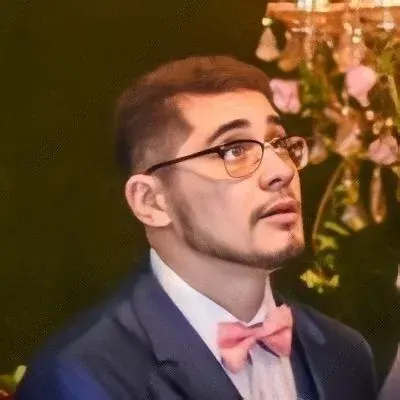
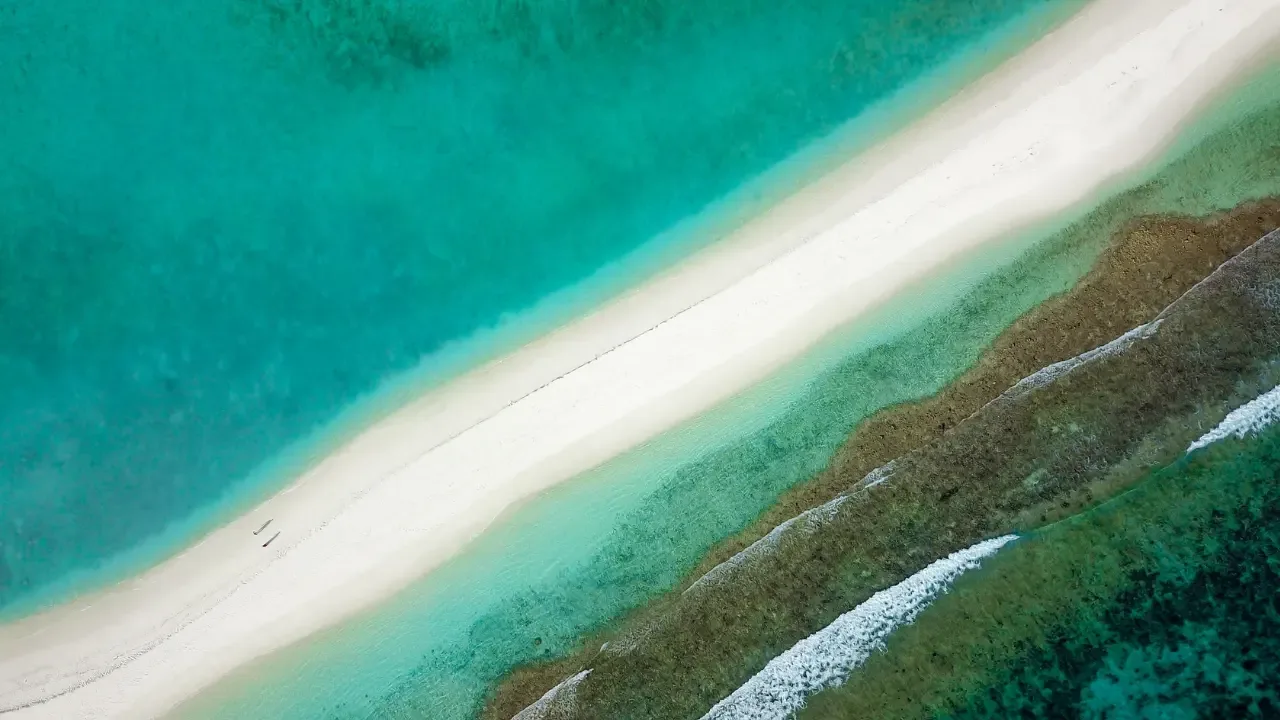
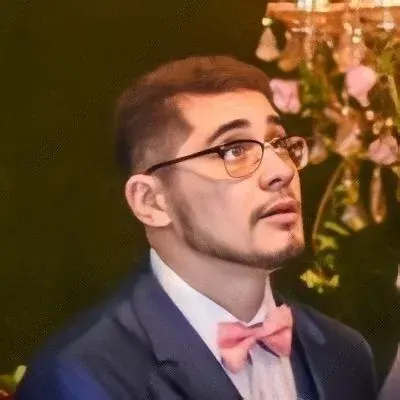
How to Read and Write INI File with Python3 🐍
Do you need to read, write, or create an INI file using Python3? Look no further! In this guide, we'll walk you through the process step by step, provide easy solutions to common issues, and give you a compelling call-to-action to encourage reader engagement. Let's get started!
Understanding INI Files 📝
INI files are a simple and widely-used configuration file format. They consist of sections, keys, and values. Each section is enclosed within square brackets ([]
), and each key-value pair is represented with the format key = value
. INI files are commonly used to store settings and configurations for software applications.
The Problem 😕
So, you need to manipulate an INI file using Python3. Whether you want to read the file, update existing values, or add new sections and keys, there are a few things you need to know.
The Solution 💡
To read and write INI files in Python3, we can use the configparser
module. This module provides a convenient way to interact with INI files by providing methods to read, write, and update values.
Here's an example of how you can read an INI file and access the values:
import configparser
# Create a ConfigParser object
config = configparser.ConfigParser()
# Read the INI file
config.read('FILE.INI')
# Access the value of "default_path"
default_path = config.get('section_name', 'default_path')
# Print the value
print(default_path)
In the above example, make sure to replace 'section_name'
with the actual section name in your INI file.
Now, let's see how we can update an existing value or add a new section/key:
import configparser
# Create a ConfigParser object
config = configparser.ConfigParser()
# Read the INI file
config.read('FILE.INI')
# Update an existing value
config.set('section_name', 'default_path', '/var/shared/')
# Add a new section and key
config.add_section('new_section')
config.set('new_section', 'new_key', 'new_value')
# Write the changes back to the INI file
with open('FILE.INI', 'w') as file:
config.write(file)
By using the set()
method, you can update existing values, and by using the add_section()
and set()
methods, you can add new sections and keys.
Handling Common Issues 🚧
Issue 1: File Not Found
If you encounter a FileNotFoundError
when trying to read an INI file, make sure the file actually exists in the specified path. Double-check the file name and path to ensure they are correct.
Issue 2: Key or Section Not Found
When accessing a key or section that doesn't exist, the configparser
module will raise a NoOptionError
or NoSectionError
, respectively. To handle these errors, you can use exception handling techniques such as try-except
blocks.
import configparser
config = configparser.ConfigParser()
try:
value = config.get('section_name', 'non_existing_key')
except configparser.NoOptionError:
print('The key was not found.')
Your Turn! 🚀
Now that you know how to read and write INI files using Python3, it's time to put your knowledge into practice! Try manipulating an INI file by following the examples mentioned above. Share your experience with us in the comments section below.
Don't forget to subscribe to our newsletter to receive more Python tips and tricks delivered straight to your inbox! Happy coding! 😊👩💻👨💻
📌 TL;DR
INI files are a simple and widely-used configuration file format.
Use the
configparser
module in Python3 to read, write, and update INI files.Use the
get()
method to retrieve values from an INI file.Use the
set()
method to update existing values or add new ones.Handle common issues such as file not found or key/section not found using exception handling.
Put your knowledge into practice and share your experience in the comments section.