How to read a file line-by-line into a list?
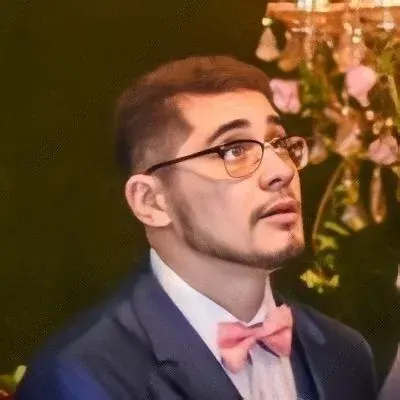
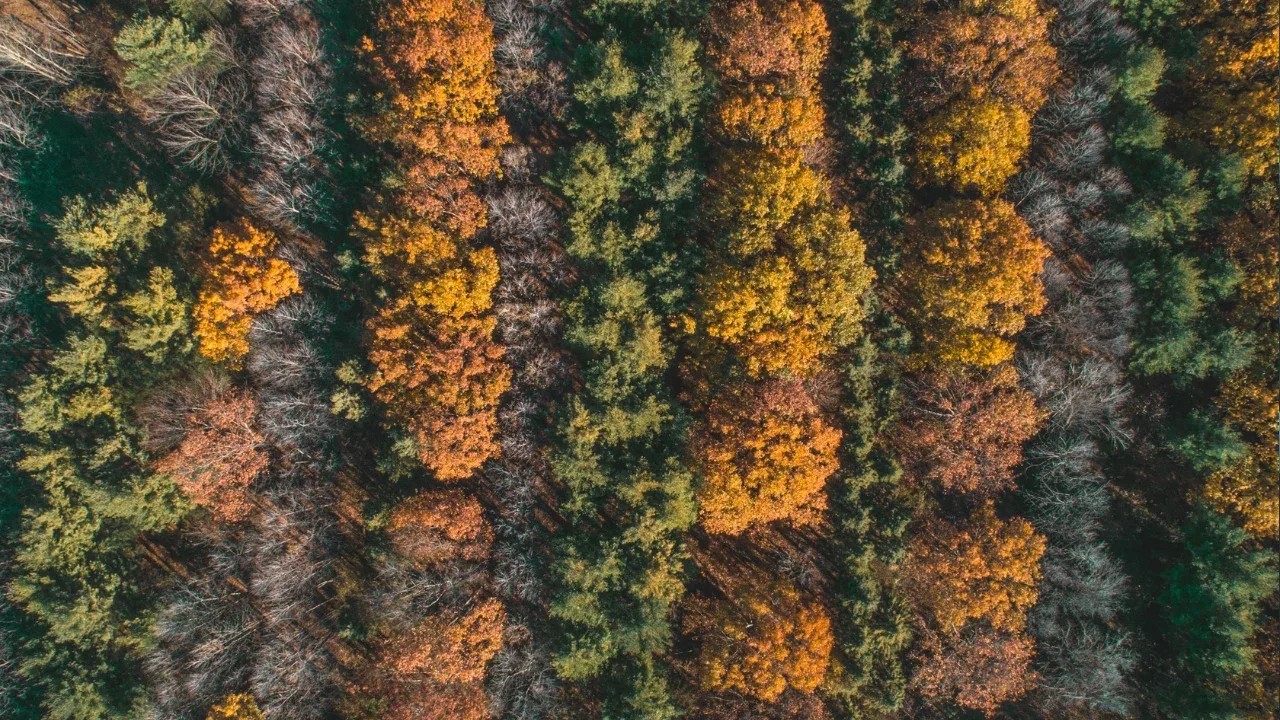
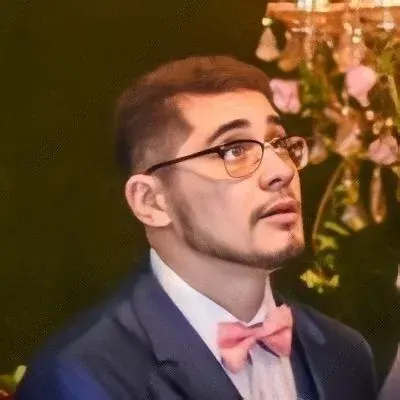
đđđ How to Read a File Line-by-Line into a List in Python đđģī¸
Are you struggling to read a file in Python and store each line as an element in a list? Look no further, as we're about to dive into an easy and efficient solution to this common problem! đ
đ¤ Understanding the Problem
The main challenge here is to open a file and extract each line as a separate element of a list. By doing so, you can then manipulate or analyze the contents of the file more easily.
âī¸ The Solution
Thankfully, Python provides an elegant way to accomplish this task using a context manager and list comprehension. Here's the step-by-step guide:
Open the file: Start by opening the file using the built-in
open()
function. This function accepts the file path as its parameter and returns a file object.
with open('filename.txt', 'r') as file:
# Continue to next step
â ī¸ Replace 'filename.txt'
with the actual file name and extension you want to read.
Read the lines: Inside the
with
block, you can now loop through the file object and read each line using afor
loop. This will allow you to iterate over each line of the file.
with open('filename.txt', 'r') as file:
lines = [line.strip() for line in file]
âšī¸ī¸ The `strip()` method removes any leading or trailing white spaces and newlines from each line.
Store in a list: With the help of list comprehension, you can append each line to a list called
lines
while simultaneously stripping out any unwanted characters.
with open('filename.txt', 'r') as file:
lines = [line.strip() for line in file]
And that's it! đī¸ You now have a list, lines
, which contains each line of the file as a separate element. You can now use this list for further processing or analysis.
đ Common Issues and Troubleshooting
Here are a couple of common issues you might encounter while reading a file line-by-line into a list, along with their solutions:
âī¸ Issue 1: Improper file path
The error message FileNotFoundError
might pop up if Python cannot locate the specified file. Double-check the file path and ensure that it is correctly spelled and provided in the proper format.
âī¸ Issue 2: Incorrect file handling mode
The function open()
accepts a second parameter to specify the file handling mode. If you accidentally set it to 'w'
for writing instead of 'r'
for reading, you won't be able to read the lines.
Make sure you set the correct mode to ensure the file is opened for reading by passing 'r'
as the second argument.
đĸ Take Action and Enhance Your Python Skills!
Now that you know how to read a file line-by-line into a list using Python, it's time to put this knowledge into practice! đī¸
Try implementing this technique in your own Python projects and experiment with different file types and formats.
Share this blog post with your friends or fellow developers who might find it helpful.
Have any other Python-related questions or struggling with a particular problem? Let us know in the comments below! We love helping fellow coders find solutions. đ
Happy coding! â¨ī¸đģī¸