How to query as GROUP BY in Django?
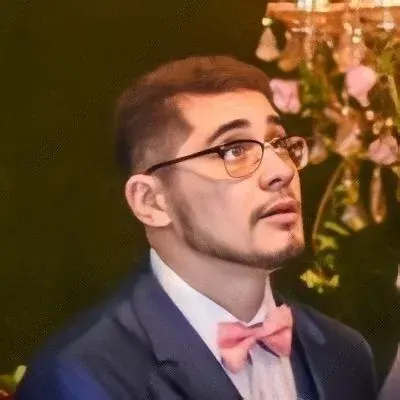
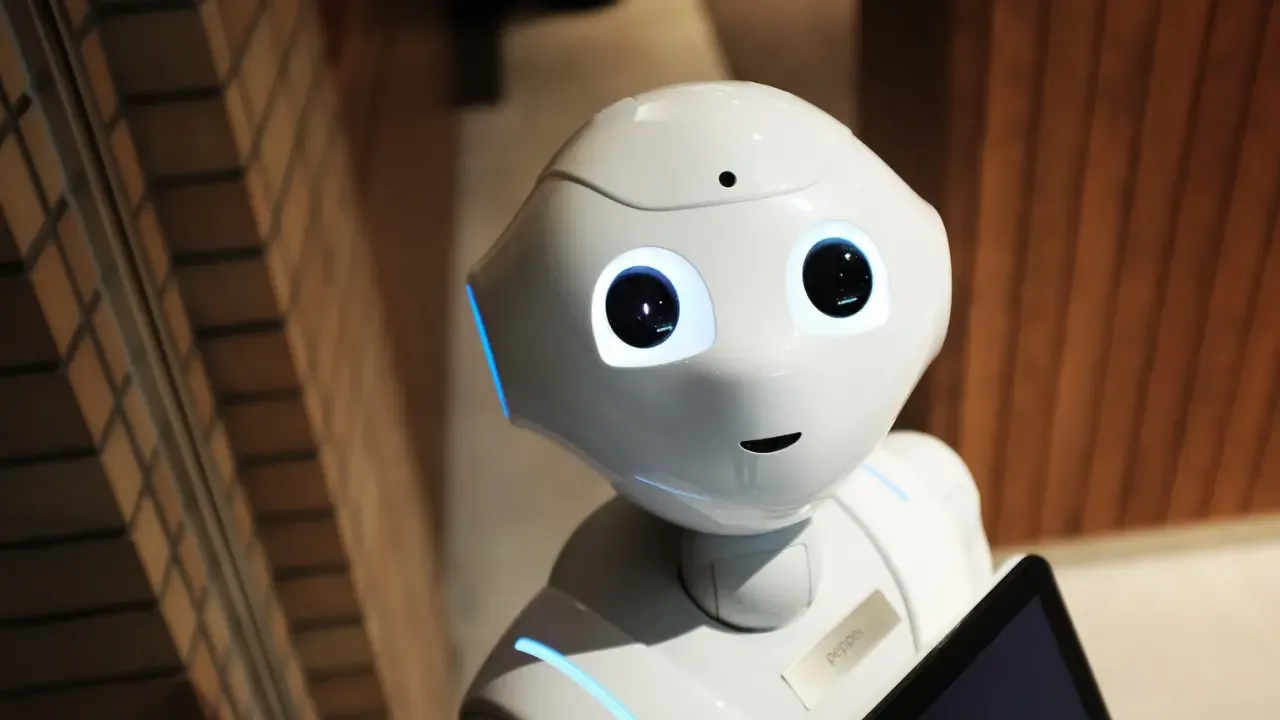
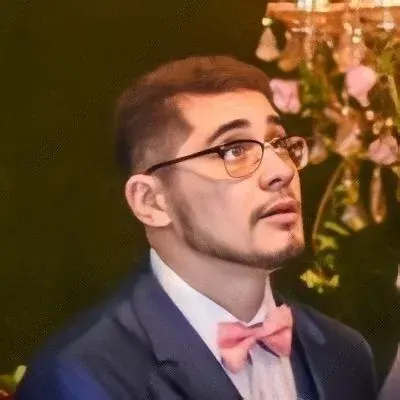
How to query as GROUP BY in Django? 🤔
Are you struggling with grouping your Django query results just like you would with the GROUP BY
clause in SQL? Don't worry, you're not alone! Many Django developers face this challenge. In this blog post, we will explore common issues and provide easy solutions to help you achieve the desired result. 🎯
Understanding the problem 🧐
Let's start by taking a look at the problem in hand. Suppose we have a model called Members
, and we want to query all members and group them by their designation. 🌟
Members.objects.all()
The query returns the following results:
Eric, Salesman, X-Shop
Freddie, Manager, X2-Shop
Teddy, Salesman, X2-Shop
Sean, Manager, X2-Shop
However, this is not what we want. We want to group the results based on the designation
field. Using the group_by
method might seem like the logical solution:
Members.objects.all().group_by('designation')
But unfortunately, this approach won't work as expected. 😞
Exploring the solution 🚀
Now let's dive into the best Django alternative to achieve grouping without patching any core Django files. 🎉
Solution 1: Using values
and annotate
methods ✨
One approach to achieve grouping in Django is by using the combination of the values
and annotate
methods. Here's how you can do it:
from django.db.models import Count
Members.objects.values('designation').annotate(count=Count('designation'))
This query will group the Members
by their designation
and return the count of members within each group. The result will look something like this:
{'designation': 'Salesman', 'count': 2}
{'designation': 'Manager', 'count': 2}
By using the values
method, we specify the field to group by, and the annotate
method performs the grouping and counting operation.
Solution 2: Using distinct
method 🌈
Another way to achieve the desired grouping is by using the distinct
method. This approach is suitable when you only want a distinct list of values for a specific field. Here's how you can do it:
Members.objects.values('designation').distinct()
The above query will return a list of distinct designations:
{'designation': 'Salesman'}
{'designation': 'Manager'}
Your turn to try it out! 💡
Now that you have learned two different approaches to achieve grouping in Django, it's time to put that knowledge into action. Go ahead and try out these solutions in your Django project. Let us know in the comments which approach worked best for you, or if you have any other questions or suggestions. We love hearing from our readers! 💌
So, stop struggling with grouping in Django and start implementing these easy solutions in your codebase. Happy coding! 💻💪
Conclusion 🎉
In this blog post, we explored how to perform grouping in Django, which acts like the GROUP BY
clause in SQL. We provided easy-to-implement solutions using the values
, annotate
, and distinct
methods. Now you can confidently group your Django query results without the need for any patching or complex tricks. 🙌
If you found this blog post helpful, don't forget to share it with your fellow Django developers. Knowledge shared is knowledge multiplied! 😉💡
Stay tuned for more Django tips and tricks on our blog. See you next time! 👋