How to pull a random record using Django"s ORM?
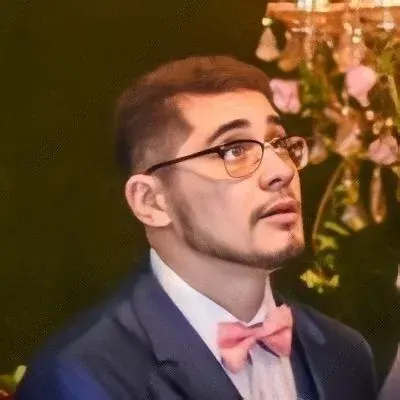
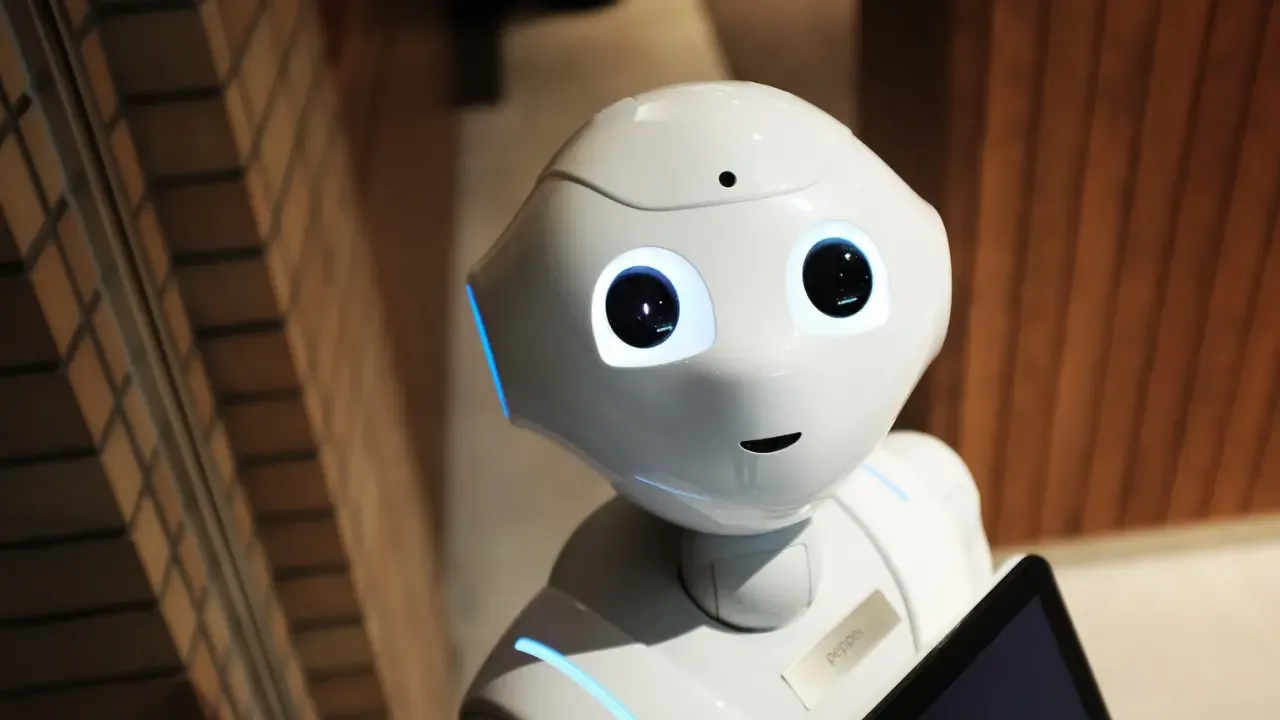
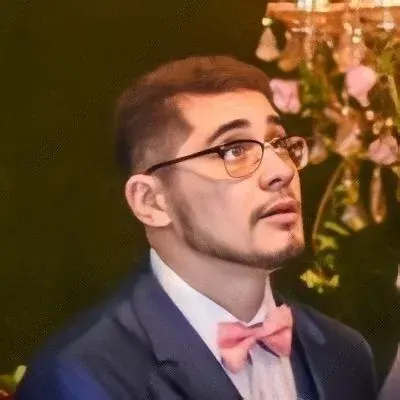
šØ How to Pull a Random Record using Django's ORM šØ
š Hey there fellow Django enthusiasts! Are you struggling with pulling a random record from your Django model? We've got you covered! In this guide, we'll explore different easy solutions to help you achieve this effortlessly. Let's dive in! šŖ
š§ The Problem: Pulling a random record š²
You have a fantastic website showcasing beautiful paintings, and you want to add a touch of randomness by displaying a random painting on your main webpage. While pulling the newest, least visited, and most popular paintings is a breeze with Django's ORM, pulling a random record gives you some trouble. But fret not, we have got the solution! š
š Option 1: Using Django's ORM inside the Model šļø
You don't want to clutter your view with random record logic - it should be handled within your model, right? Absolutely! Here's a solution that keeps everything neat and tidy:
1ļøā£ In your models.py file, create a custom manager by extending the Manager
class:
class RandomPaintingManager(models.Manager):
def get_random(self):
count = self.count()
random_index = randrange(count)
return self.all()[random_index]
2ļøā£ Then, in your Painting
model, add the custom manager:
class Painting(models.Model):
# Your fields here
objects = RandomPaintingManager()
3ļøā£ Voila! Now you can easily retrieve a random painting within your model:
random_paint = Painting.objects.get_random()
š„ This approach encapsulates the random record logic within the model, keeping your view clean and your codebase organized. š
š Option 2: Leveraging Django's query functions šļø
If you prefer a more concise approach, Django provides query functions that can solve your random record dilemma in a single line!
1ļøā£ Simply update your view by using the order_by('?')
function:
random_paint = Painting.objects.order_by('?').first()
š Hooray! With just one line of code, you can fetch a random painting! š¼ļø
š„ This approach allows you to leverage Django's powerful query functions, making your code more expressive and readable. š
š¬ Share Your Random Painting Experience! š¬
Now that you know how to pull a random record using Django's ORM, we want to hear from you! Have you successfully implemented the solutions we provided? Do you have any other interesting ways of achieving the same result? Don't forget to share your thoughts and experiences in the comments below! Let's learn and grow together! š
š Pulling Random Records - Mission Accomplished! š
Congratulations, you've mastered the art šØ of pulling random records using Django's ORM! Whether you prefer encapsulating the logic within the model or leveraging Django's query functions, you now have the tools to add a touch of randomness to your website effortlessly. Give it a try, and let us know the masterpieces you unearthed! š¼ļøš