How to print without a newline or space
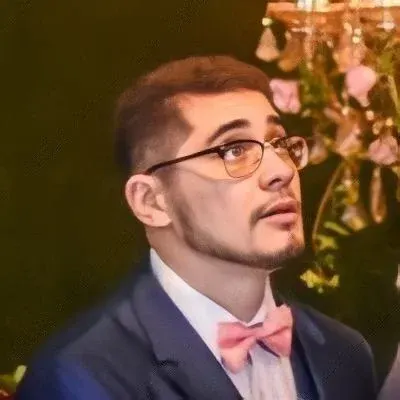
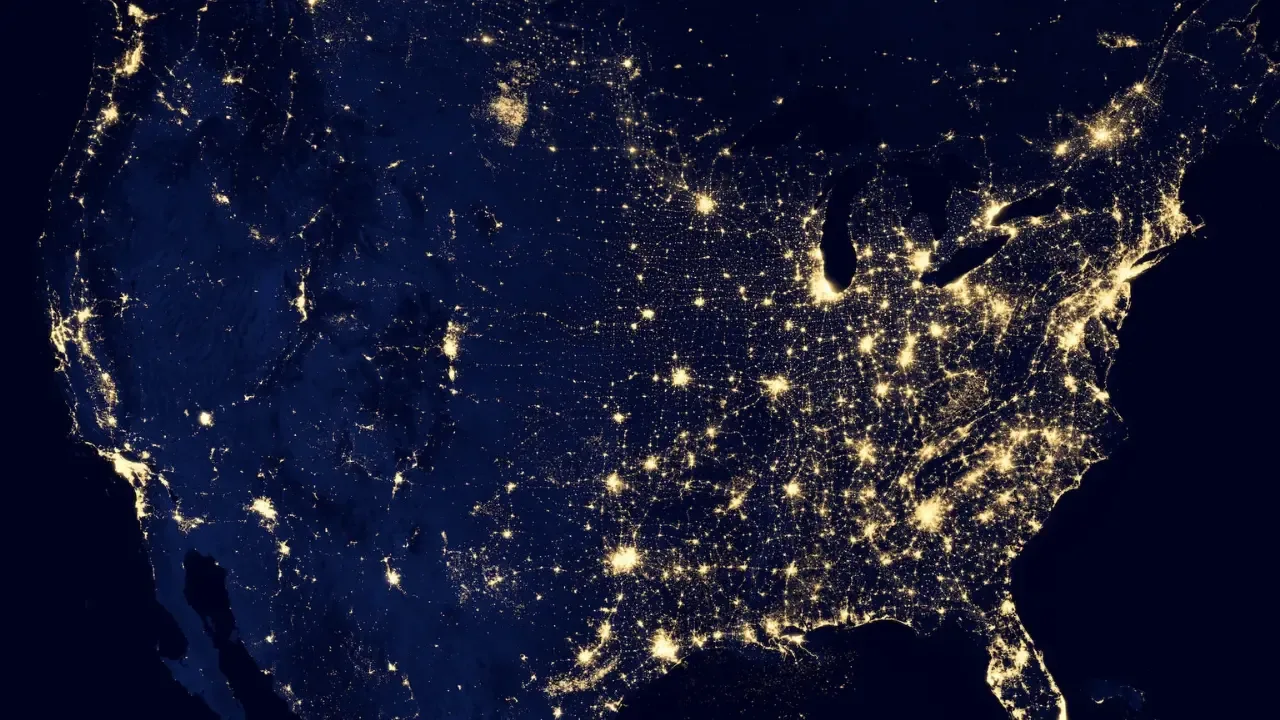
How to Print Without a Newline or Space: A Complete Guide
If you've ever used the print
function in Python, you may have noticed that by default, it adds either a newline character (\n
) or a space between each value you pass to it. But what if you want to print multiple values without any spacing or line breaks? In this blog post, we'll explore the common issues surrounding this question and provide easy solutions to help you achieve the desired output. 💻📝🤔
The Problem: Unwanted Spacing Between Values
Consider the following examples using the print
function in Python:
>>> for i in range(4):
... print('.')
.
.
.
.
>>> print('.', '.', '.', '.')
. . . .
In both cases, a newline or space is automatically added between each period ('.'). However, what if you want the output to be ....
instead? In other words, how can you "append" strings to the standard output stream without any spacing or line breaks? 📜👀
The Solution: Using the end
Parameter
Fortunately, Python provides a simple solution to this problem. The print
function allows you to specify the end
parameter, which determines what character or string to append at the end of each call to print
.
To achieve the desired output, you can set the end
parameter to an empty string ('') like this:
>>> for i in range(4):
... print('.', end='')
....
>>> print('.', '.', '.', '.', end='')
....
By providing an empty string as the value for end
, you effectively remove the default newline or space character that print
adds at the end of each call. This allows you to print multiple values without any additional spacing or line breaks. 🙌✨💯
Taking it Further: Adding Custom Separators
Beyond just removing the default spacing, you can also customize the separators between your values when printing. Python allows you to use the sep
parameter to specify a string that separates the different values you pass to print
.
Here's an example that demonstrates how to print multiple values without spaces, but with a period ('.') as the separator:
>>> values = ['one', 'two', 'three', 'four']
>>> print(*values, sep='.')
one.two.three.four
By using the sep
parameter, you can control the separator between values and achieve the desired output format. This gives you more flexibility in how you present your printed output. 🎉🔠🖨️
Conclusion: Printing Without Spacing Made Easy
We've explored the common problem of printing without unwanted spacing or line breaks in Python. By using the end
parameter with an empty string, you can achieve the desired output format. Additionally, you can take it further by customizing the separators between values using the sep
parameter.
Next time you find yourself needing to print without spacing, remember these simple solutions. Happy coding! 😊🌟🐍
Have any other tips or tricks for printing without spacing? Share them in the comments below! 📝💬👇
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
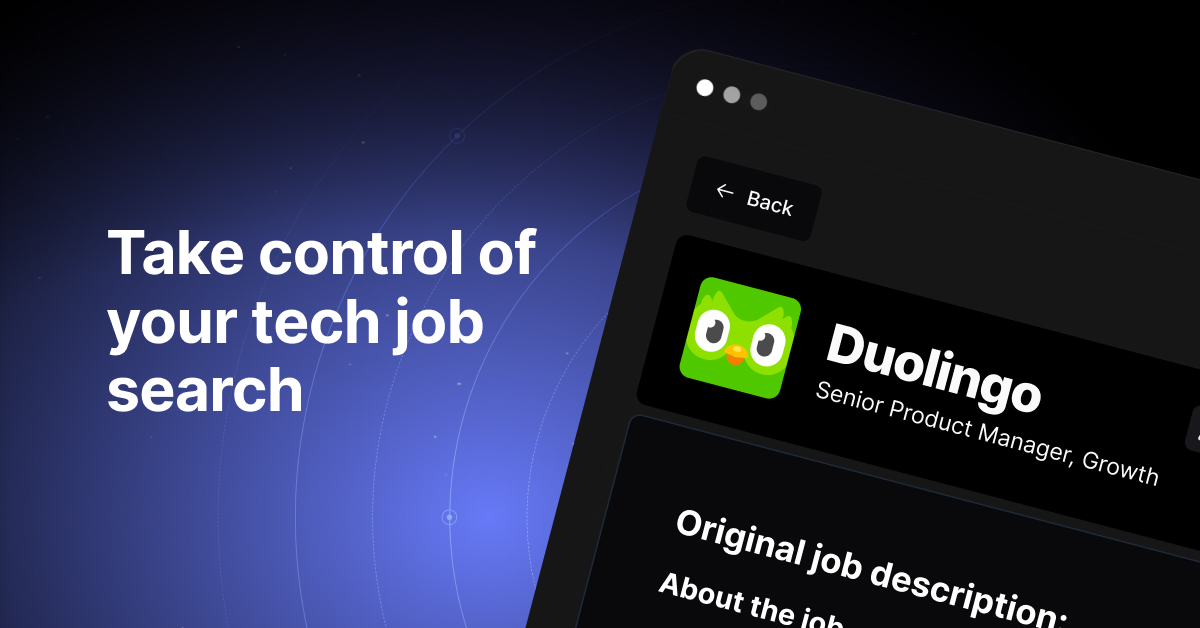