How to print pandas DataFrame without index
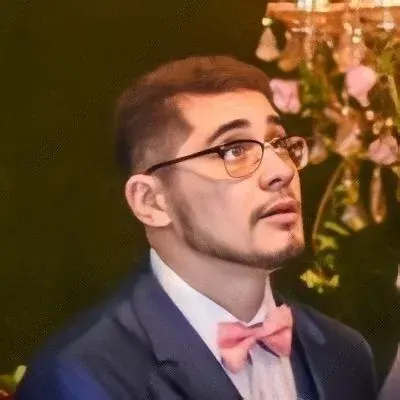
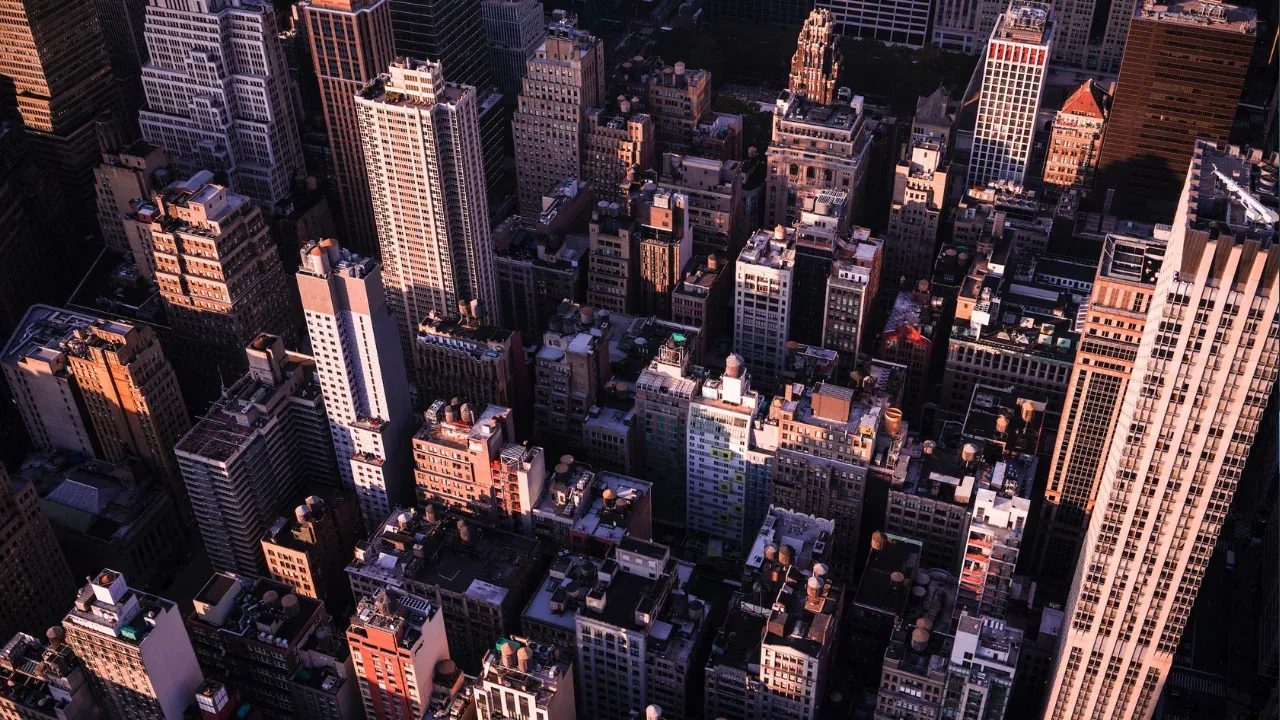
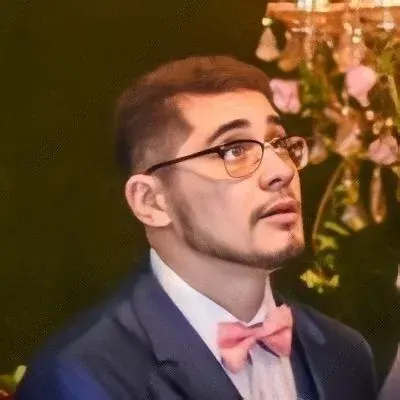
How to Print Pandas DataFrame Without Index: A Simple Guide
Are you struggling with printing a Pandas DataFrame without the index? Look no further! In this blog post, we will address this common issue and provide you with easy solutions. Additionally, if you have a datetime column and only want to print the time, we've got you covered.
The Problem: Printing the Whole DataFrame Without the Index
Let's start by understanding the problem. Imagine you have a DataFrame like this:
User ID Enter Time Activity Number
0 123 2014-07-08 00:09:00 1411
1 123 2014-07-08 00:18:00 893
2 123 2014-07-08 00:49:00 1041
But what you really want is to print it like this:
User ID Enter Time Activity Number
123 00:09:00 1411
123 00:18:00 893
123 00:49:00 1041
Solution 1: Using the to_string()
Method
The simplest way to print the DataFrame without the index is to use the to_string()
method. Here's how you can do it:
print(df.to_string(index=False))
By setting index=False
, you are telling Pandas not to print the index values. This will give you the desired output.
Solution 2: Resetting the Index Before Printing
Another approach is to reset the index of your DataFrame before printing it. Here's how you can achieve it:
df_reset_index = df.reset_index(drop=True)
print(df_reset_index)
The reset_index()
method with drop=True
removes the old index and replaces it with a new one. By doing this, you effectively exclude the index from the printed output.
Solution 3: Converting the DateTime Column to Time Only
If you have a datetime column and want to print only the time, we can solve that too! Here's a simple solution using the strftime()
function:
df["Enter Time"] = df["Enter Time"].dt.strftime("%H:%M:%S")
print(df.to_string(index=False))
In this example, we're using the strftime()
function to format the time as "%H:%M:%S". Modify the format as per your specific requirements.
Wrapping Up
Printing a Pandas DataFrame without the index is a common task that can be easily accomplished using the solutions provided in this guide. Whether you prefer to use the to_string()
method, reset the index, or convert a datetime column to time-only, these approaches should give you the desired output.
Now that you know how to tackle this issue, go ahead and give it a try in your own projects. Happy coding! 😄
Have any more questions or facing other Pandas-related challenges? Let us know in the comments below! We're here to help.