How to postpone/defer the evaluation of f-strings?
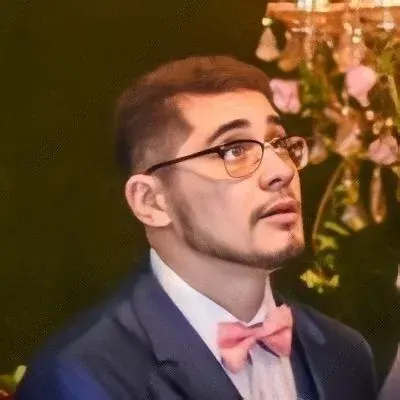
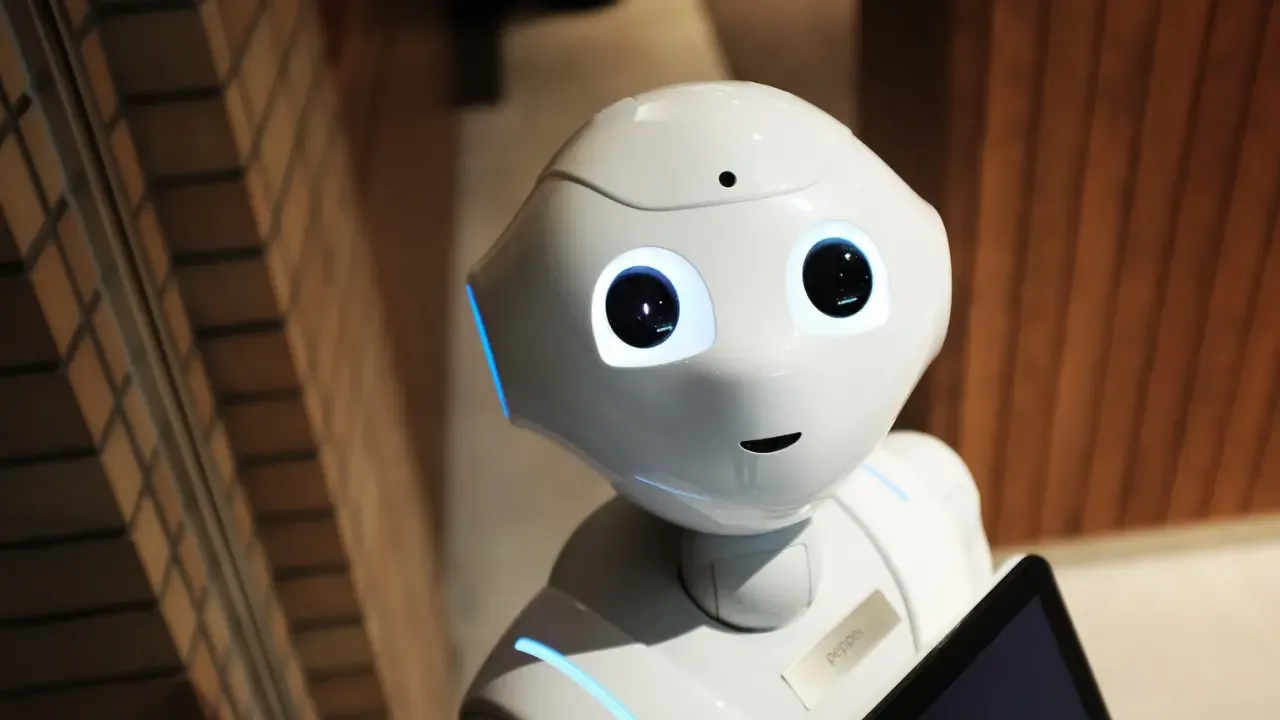
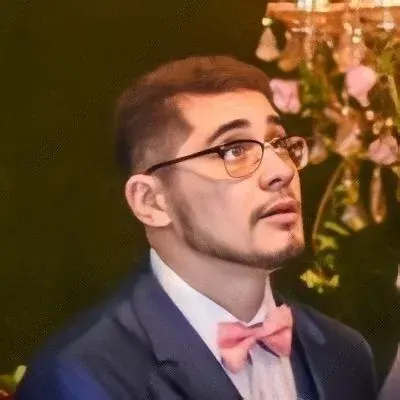
๐ Postponing/Deferring the Evaluation of f-strings
Are you tired of lengthy template code when generating files? ๐งพ Look no further! Python 3.6 introduced f-strings ๐, offering a concise way to format strings. But what if you want to define your template elsewhere and avoid using the .format(**locals())
call? ๐ค In this blog post, we'll explore a solution to this problem and help you optimize your code. Let's dive in! ๐
The Old Way vs. The F-String Way
In the old days, you might have written code like this:
template_a = "The current name is {name}"
names = ["foo", "bar"]
for name in names:
print(template_a.format(**locals()))
This works, but it's not as clean and concise as we'd like. ๐คทโโ๏ธ Fortunately, f-strings allow us to simplify our code:
names = ["foo", "bar"]
for name in names:
print(f"The current name is {name}")
๐ But what if you want to bring in a string from elsewhere and have it interpreted as an f-string? Can we avoid using .format(**locals())
? Let's find a solution! ๐ก
Enter the "Magic" Function
To achieve our desired output without reading the file twice, we need a way to convert a static string into an f-string. ๐ฎ Unfortunately, Python doesn't provide a built-in "magic" function for this purpose. However, we can create our own function to achieve similar results.
Here's how we can accomplish this:
def magic_fstring_function(template):
# Evaluate the template string as an f-string
return eval(f'f"""{template}"""')
template_a = magic_fstring_function("The current name is {name}")
# OR [Ideal2] template_a = magic_fstring_function(open('template.txt').read())
names = ["foo", "bar"]
for name in names:
print(template_a)
By using the magic_fstring_function
, we can interpret the static string as an f-string. This allows us to use it in our code without the need for .format(**locals())
. ๐
Conclusion
With our custom "magic" function, you can now bring in a string and have it interpreted as an f-string, avoiding the use of .format(**locals())
. ๐ฉโจ This helps reduce code complexity and increases the readability of your code. Remember, f-strings are an excellent tool for concise and clean string formatting in Python! ๐
Try implementing this solution in your code and share your experiences with us! We'd love to hear how it works for you. ๐
Start using f-strings today and level upโฌ๏ธ your Python programming! Happy coding! ๐ป๐
Have any questions? Need more help? Feel free to reach out in the comments below.
Share this post with your fellow Pythonistas and spread the f-string magic! โจ๐