How to POST JSON data with Python Requests?
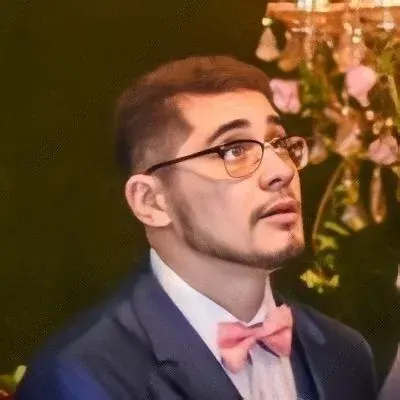
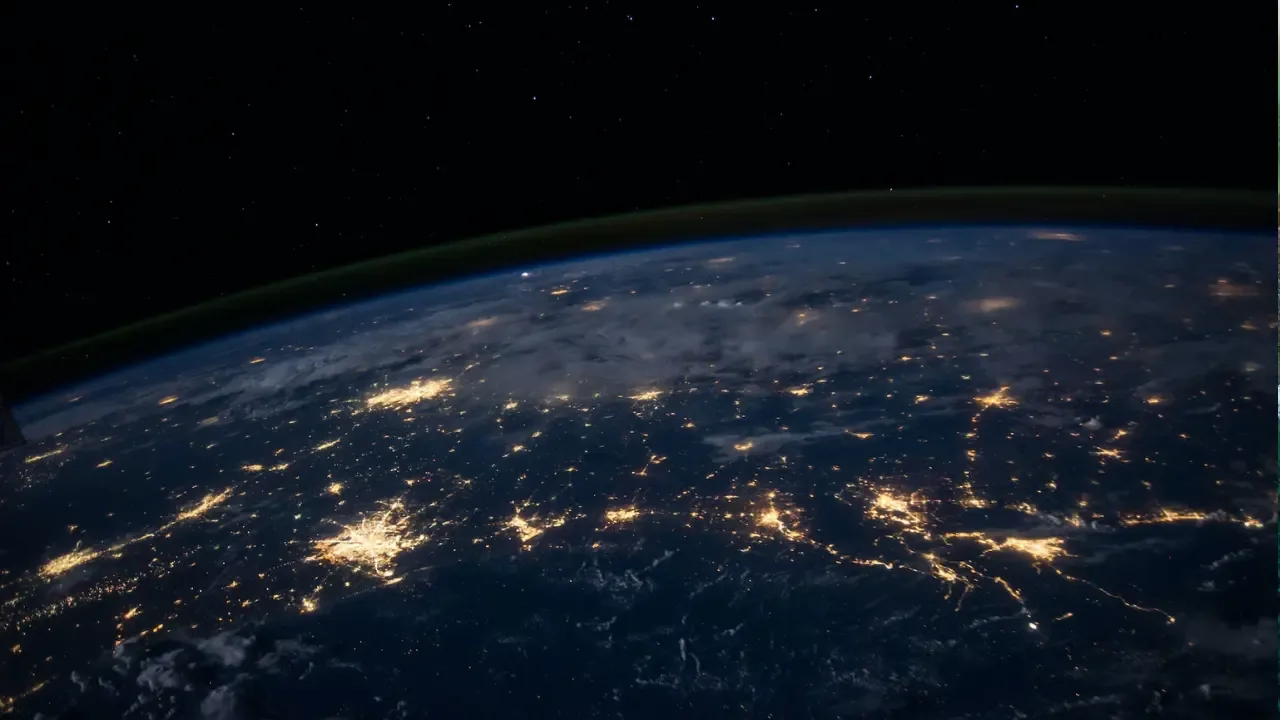
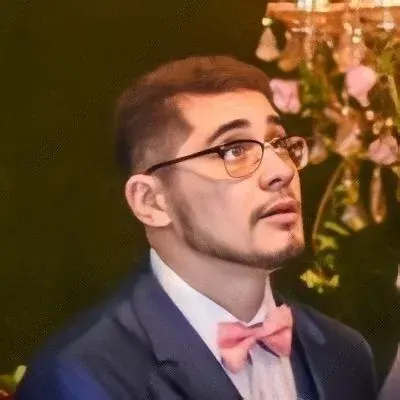
How to POST JSON data with Python Requests?
Hey there! 👋 In this guide, we'll dive into the world of Python Requests and learn how to POST JSON data. We'll address the common issue of encountering a "400 Bad Request" error and provide you with easy solutions to overcome it. So let's get started!
Understanding the problem
Based on the context you provided, it seems like you're trying to send a JSON payload from a client to a server using Python 2.7.1 and simplejson. The client is leveraging the power of Requests, while the server is implemented using CherryPy. However, you're facing a roadblock as you receive a "400 Bad Request" error when attempting to POST the JSON data.
Analyzing the code
To better understand the issue, let's take a closer look at the code you provided.
Client code
data = {'sender': 'Alice', 'receiver': 'Bob', 'message': 'We did it!'}
data_json = simplejson.dumps(data)
payload = {'json_payload': data_json}
r = requests.post("http://localhost:8080", data=payload)
In the client code snippet, you're creating a dictionary named data
that represents the JSON payload you want to send. Next, you're using simplejson
to serialize the data
dictionary into a JSON string named data_json
. After that, you create a payload dictionary by assigning the data_json
as the value for the key json_payload
. Finally, you make a POST request using the requests.post
method, passing the payload as the data
parameter.
Server code
class Root(object):
def __init__(self, content):
self.content = content
print self.content # this works
exposed = True
def GET(self):
cherrypy.response.headers['Content-Type'] = 'application/json'
return simplejson.dumps(self.content)
def POST(self):
self.content = simplejson.loads(cherrypy.request.body.read())
On the server side, you have a CherryPy class named Root
. In the POST
method, you're attempting to load the JSON data from the request body using simplejson.loads
and assigning it to self.content
. However, you're encountering the "400 Bad Request" error at this point.
Resolving the issue
To overcome the "400 Bad Request" error, we need to make a couple of adjustments to the code.
Adjusting the client code
To correctly send JSON data using Requests, we need to pass the JSON string as the request body instead of using the data
parameter. We achieve this by assigning the JSON string to the data
parameter without calling simplejson.dumps
explicitly.
Here's the adjusted client code:
data = {'sender': 'Alice', 'receiver': 'Bob', 'message': 'We did it!'}
r = requests.post("http://localhost:8080", json=data)
By using the json
parameter instead of data
, Requests will automatically serialize the data
dictionary into a JSON string and set the appropriate headers.
Modifying the server code
To ensure that the server correctly interprets the incoming JSON data, we'll make a small change to the server code as well. After reading the request body, we'll set the "Content-Type"
header to "application/json"
to indicate that the request contains JSON data.
Here's the modified server code:
class Root(object):
def __init__(self, content):
self.content = content
print(self.content) # this works
exposed = True
def GET(self):
cherrypy.response.headers['Content-Type'] = 'application/json'
return simplejson.dumps(self.content)
def POST(self):
cherrypy.response.headers['Content-Type'] = 'application/json'
self.content = simplejson.loads(cherrypy.request.body.read())
By setting the "Content-Type"
header explicitly, we ensure that CherryPy correctly interprets the incoming JSON data.
Wrapping up
And that's it! 🎉 By making these adjustments, you should now be able to successfully POST JSON data from your client to the server without encountering the "400 Bad Request" error. Remember to use the adjusted versions of the client and server code provided in this guide.
If you have any other questions or need further assistance, feel free to reach out in the comments below. Happy coding! 💻