How to perform OR condition in django queryset?
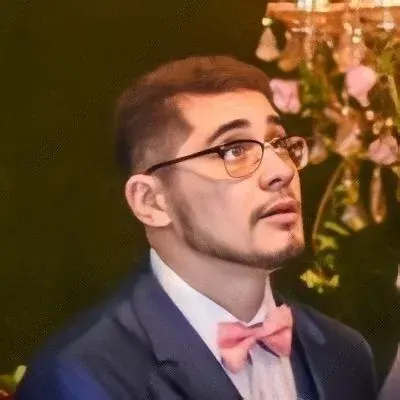
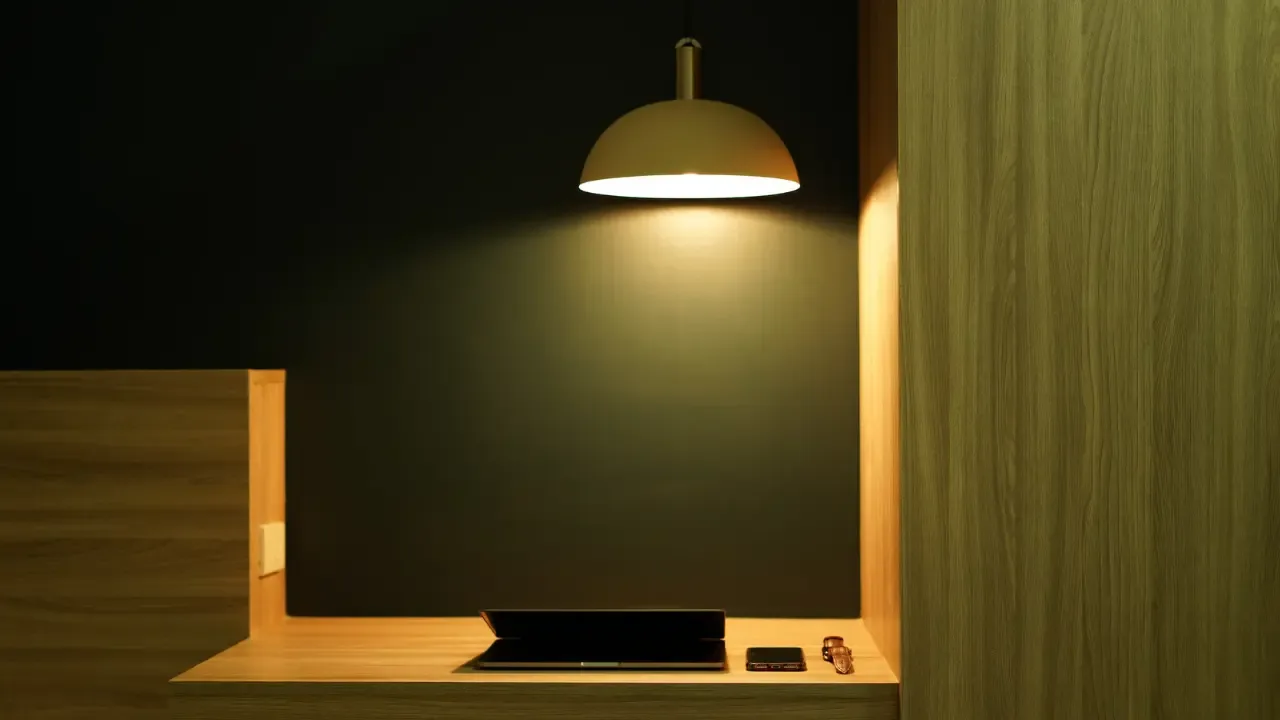
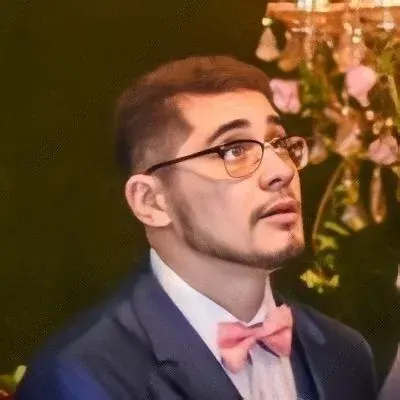
How to Perform OR Condition in Django Queryset? π
Are you stuck trying to perform an OR condition in a Django queryset? Don't worry, we've got you covered! In this blog post, we'll show you how to tackle this common issue and provide you with easy solutions. So, let's dive straight into it!
Understanding the Challenge π
To better comprehend the problem at hand, let's take a look at the context. Our goal is to write a Django query that is equivalent to the following SQL query:
SELECT * FROM user WHERE income >= 5000 OR income IS NULL;
However, when attempting the following Django queryset filter, we encounter a problem:
User.objects.filter(income__gte=5000, income=None)
The issue here is that the above filter conditions are being combined using AND
logic instead of OR
. As a result, we don't get the desired union of individual querysets.
Solution: Q Objects to the Rescue! π¦ΈββοΈ
Fortunately, Django provides us with a powerful tool called Q objects that allows us to perform complex queries by combining conditions using OR
, AND
, and even nested logic. Let's see how we can use them to solve our problem:
from django.db.models import Q
User.objects.filter(Q(income__gte=5000) | Q(income__isnull=True))
In the above code, we import the Q
object from django.db.models
and use it to create two separate conditions. By applying vertical bar (|
) between the conditions, we indicate an OR
operation. The first condition checks if the income is greater than or equal to 5000, while in the second condition, we verify if the income is null.
With this clever usage of Q
objects, we successfully perform an OR
condition in our Django queryset!
Putting It All Together π οΈ
To summarize, here's a complete example of how you can construct the Django queryset filter using Q
objects:
from django.db.models import Q
# Your other code...
users = User.objects.filter(Q(income__gte=5000) | Q(income__isnull=True))
# Further processing or usage of the filtered queryset...
Feel free to adapt the above code to match your specific needs. Now you can effortlessly perform an OR
condition in your Django queryset like a pro! πͺ
Share Your Thoughts! π£οΈ
We hope this guide helped you overcome the challenge of performing an OR condition in a Django queryset. If you have any questions, suggestions, or additional tips, we would love to hear from you! Share your thoughts in the comments section below and let's keep the conversation going!
And don't forget to spread the knowledge by sharing this blog post with your fellow Django developers. Together, we can make coding easier and more enjoyable for everyone! π