How to parse XML and get instances of a particular node attribute?
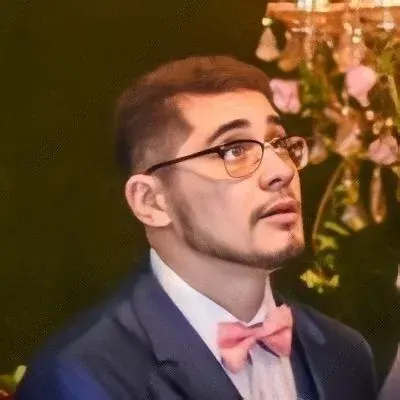
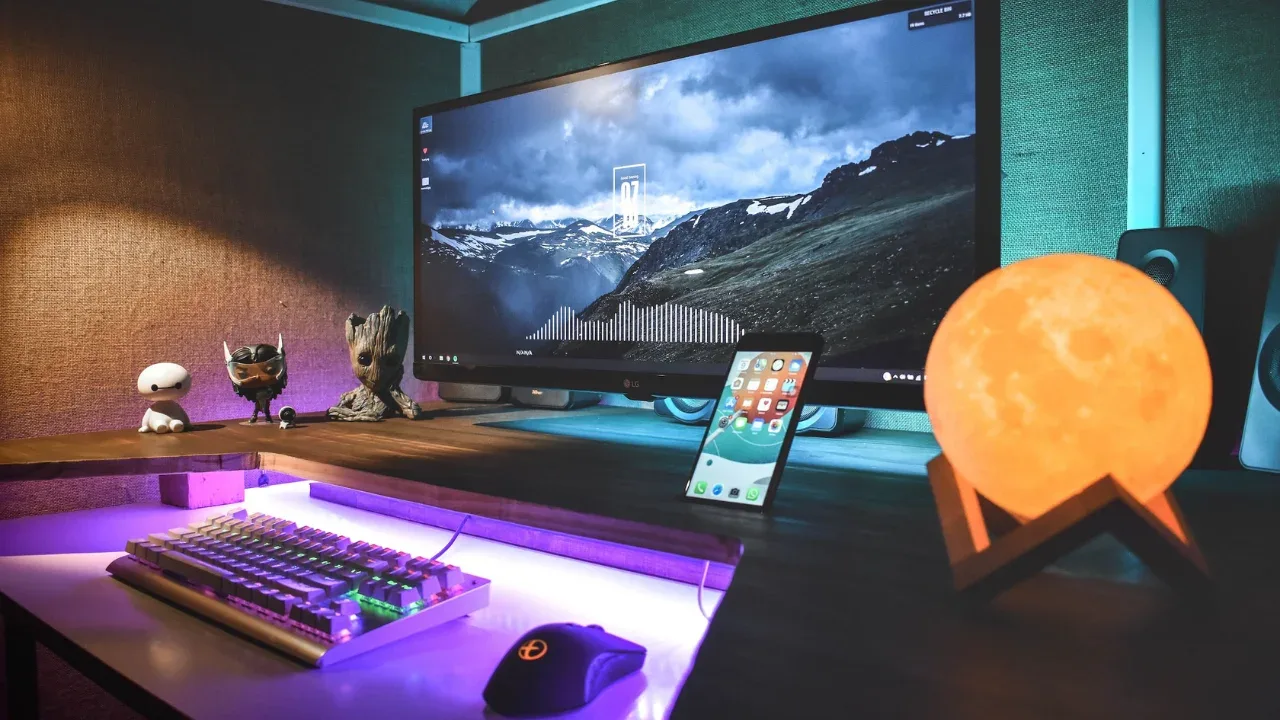
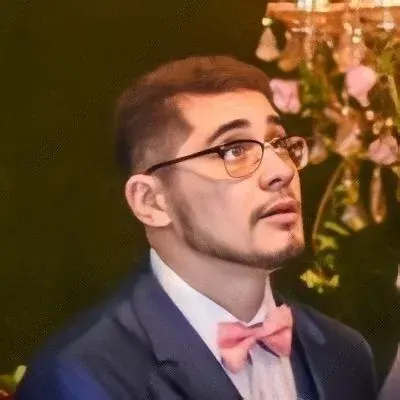
š„šParsing XML and Getting Instances of a Node Attributešš„
š Hey there, tech enthusiasts! Are you puzzled by how to parse XML and extract instances of a specific node attribute? No worries, we've got you covered! In this guide, we'll show you a step-by-step approach to tackle this common XML parsing problem. Let's dive in! š»š
Identifying the Issue š§
š¤ So, you're dealing with an XML document that has multiple rows, and you want to retrieve the values of a particular node attribute. In our example, we have a <foo>
element containing a <bar>
element that holds multiple <type>
nodes, each with a foobar
attribute. We're interested in accessing the values of those foobar
attributes, which in this case are "1" and "2". š
Getting Started š
To solve this problem, we'll use an XML parser library that suits our programming language of choice. Many languages come with built-in or third-party XML parsing libraries, making our task easier. We'll demonstrate the solution using Python's xml.etree.ElementTree
module. However, the concept can be applied to other languages and libraries as well. šš¤
The Solution š”
š» Let's go step-by-step on how to parse the XML and extract the desired node attributes using Python's xml.etree.ElementTree
:
Import the required module:
import xml.etree.ElementTree as ET
Parse the XML document:
tree = ET.parse('your_xml_file.xml')
root = tree.getroot()
Traverse through the XML structure and locate the desired node attributes:
for type_node in root.findall('.//type'):
foobar_value = type_node.attrib['foobar']
print(foobar_value)
Voila! You can now access the values of the
foobar
attribute for each<type>
node.
Putting It All Together š
Let's consider the complete code snippet, combining all the above steps:
import xml.etree.ElementTree as ET
# Parsing the XML document
tree = ET.parse('your_xml_file.xml')
root = tree.getroot()
# Retrieving the desired node attributes
for type_node in root.findall('.//type'):
foobar_value = type_node.attrib['foobar']
print(foobar_value)
And that's it! The above code will iterate through each <type>
node in your XML document and print the values of the foobar
attribute.
Take It to the Next Level šš
Now that you've learned how to extract the instances of a particular node attribute from XML, think about how you can use this knowledge to solve real-world problems! You could further process the extracted values, store them in a database, or perform calculations based on the obtained data. The possibilities are endless! š”š
š We hope this guide has helped demystify the process of parsing XML and getting instances of a specific node attribute. Now it's your turn to give it a try! Use the provided solution as a blueprint and adapt it to your preferred programming language and XML library. Have fun exploring XML's power and unleash your creativity! šāØ
Let us know in the comments if you found this guide helpful or if you have any questions. We're here to assist you! š¤š
š Don't forget to share this post with your fellow tech enthusiasts to spread the knowledge! Until next time, happy XML parsing! ššš¾