How to overcome "datetime.datetime not JSON serializable"?
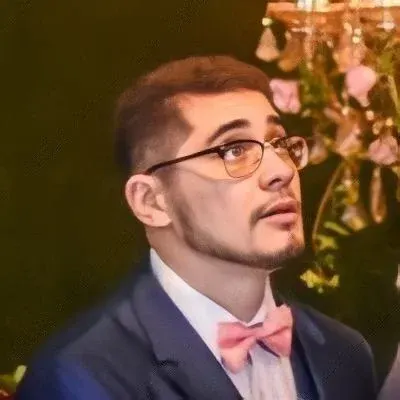
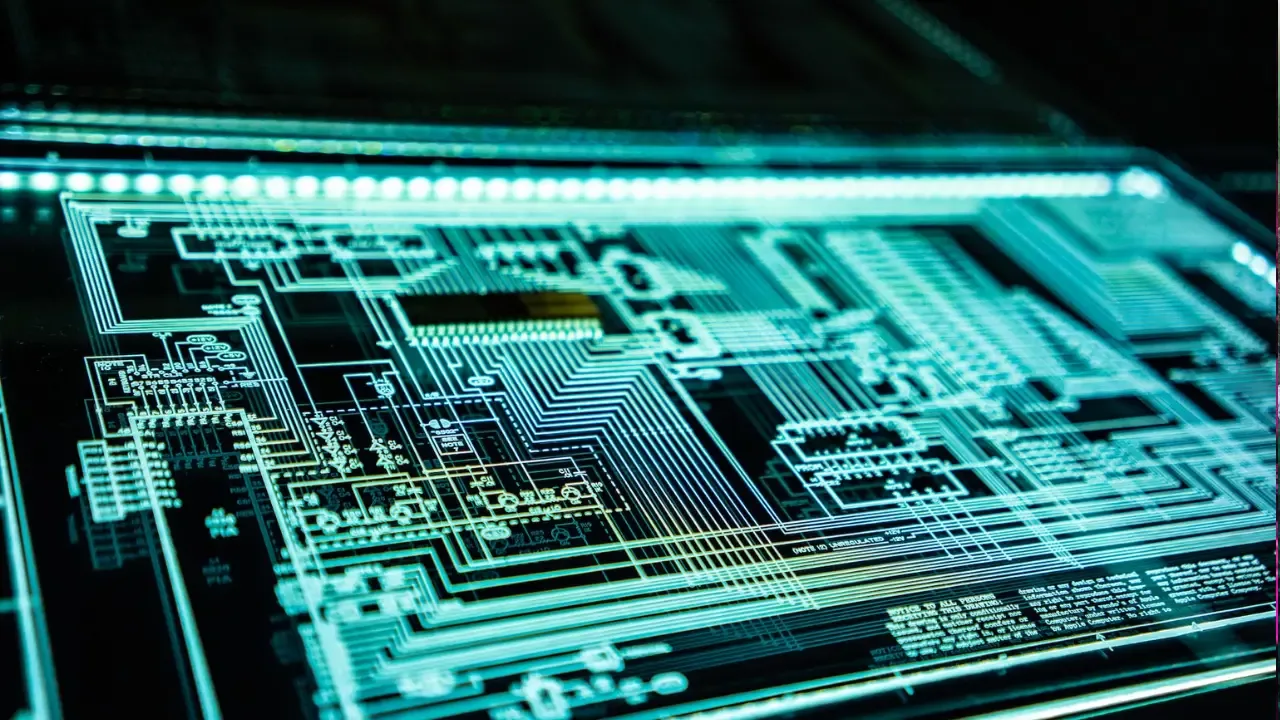
How to overcome "datetime.datetime not JSON serializable"? 🤔
So you've encountered the dreaded TypeError: datetime.datetime() is not JSON serializable
error message when trying to jsonify a dictionary that contains datetime values. Don't worry, you're not alone! This is a common issue that many developers face, especially when working with databases like MongoDB.
In this blog post, we'll explore why this error occurs, discuss potential solutions, and provide you with easy-to-implement fixes. Let's dive in! 💪
Understanding the problem 🕵️♀️
The error message is telling us that the datetime
object within the dictionary is not directly serializable to JSON. JSON only supports a limited set of data types, and datetime
is not one of them. When you try to jsonify the dictionary, it encounters the datetime
object and doesn't know how to handle it.
Solution 1: Convert datetime to string format 📅
One simple solution is to convert the datetime object to a string before serializing it. In Python, you can achieve this by using the strftime
method, which formats the datetime object into a string representation based on a given format.
Here's an example of how you can convert the datetime object in your dictionary to a string:
import json
from datetime import datetime
sample = {}
sample['title'] = "String"
sample['somedate'] = datetime.now().strftime('%Y-%m-%d %H:%M:%S')
json_data = json.dumps(sample)
By using strftime
, we convert the somedate
value to a string in the YYYY-MM-DD HH:MM:SS
format. Now, when you call json.dumps
, the dictionary can be successfully serialized into JSON because it contains only serializable data types.
Solution 2: Create a custom JSON encoder 🧰
If you find yourself handling datetime objects frequently, it might be more convenient to create a custom JSON encoder that knows how to handle datetime objects. This way, you won't have to convert datetime objects to strings every time you want to serialize a dictionary.
Here's an example of how you can create a custom JSON encoder:
import json
from datetime import datetime
class DateTimeEncoder(json.JSONEncoder):
def default(self, o):
if isinstance(o, datetime):
return o.isoformat()
return super().default(o)
sample = {}
sample['title'] = "String"
sample['somedate'] = datetime.now()
json_data = json.dumps(sample, cls=DateTimeEncoder)
In this code snippet, we create a class called DateTimeEncoder
that subclasses json.JSONEncoder
. We override the default
method, which is called for objects that are not natively serializable. Within the default
method, we check if the object is an instance of datetime
. If it is, we convert it to its ISO format using isoformat()
, which returns a string representation of the datetime object. If it's not a datetime object, we delegate the serialization responsibility to the parent class using super().default(o)
.
By passing cls=DateTimeEncoder
as an argument to json.dumps
, we tell the JSON serializer to use our custom encoder when serializing the dictionary.
Conclusion and call-to-action 💡
By understanding why the "datetime.datetime not JSON serializable" error occurs and implementing one of the solutions mentioned above, you can easily overcome this issue and successfully serialize dictionaries that contain datetime objects.
Remember, converting datetime objects to strings or using a custom JSON encoder are just two of the many possible solutions. Choose the one that best suits your needs and integrate it into your codebase.
Have you ever faced this error before? How did you solve it? Share your experiences and solutions in the comments below! Let's help each other overcome this common developer frustration. 🙌
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
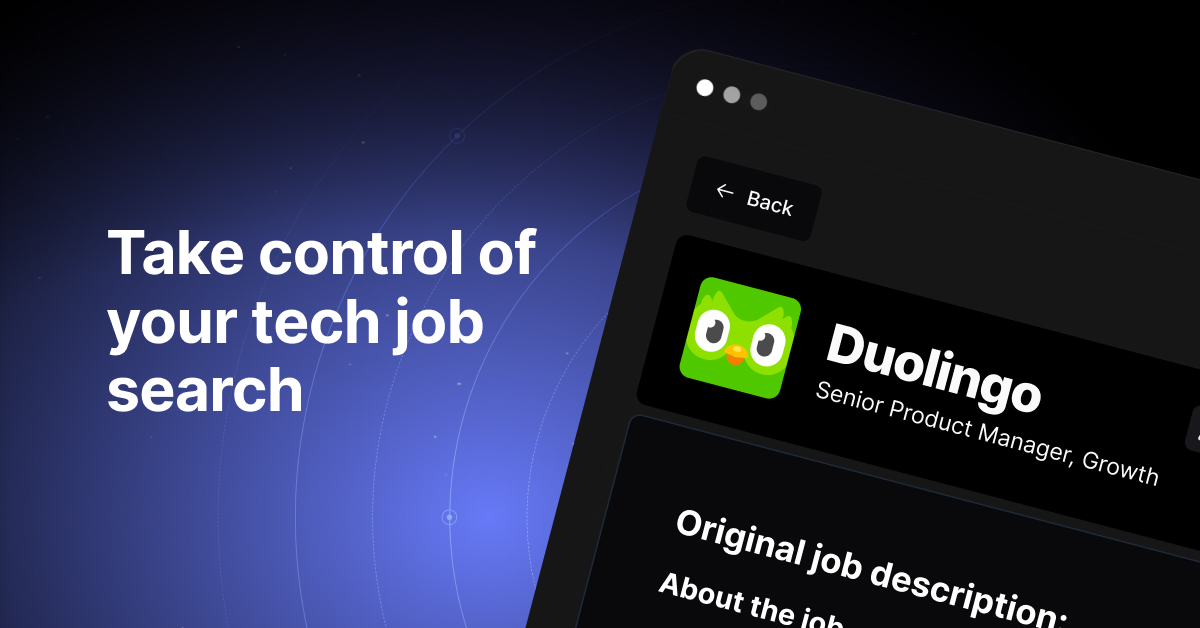