How to open a file using the open with statement
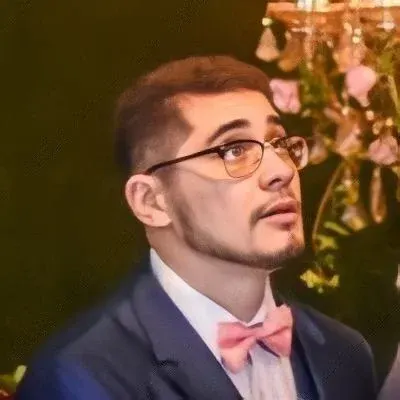
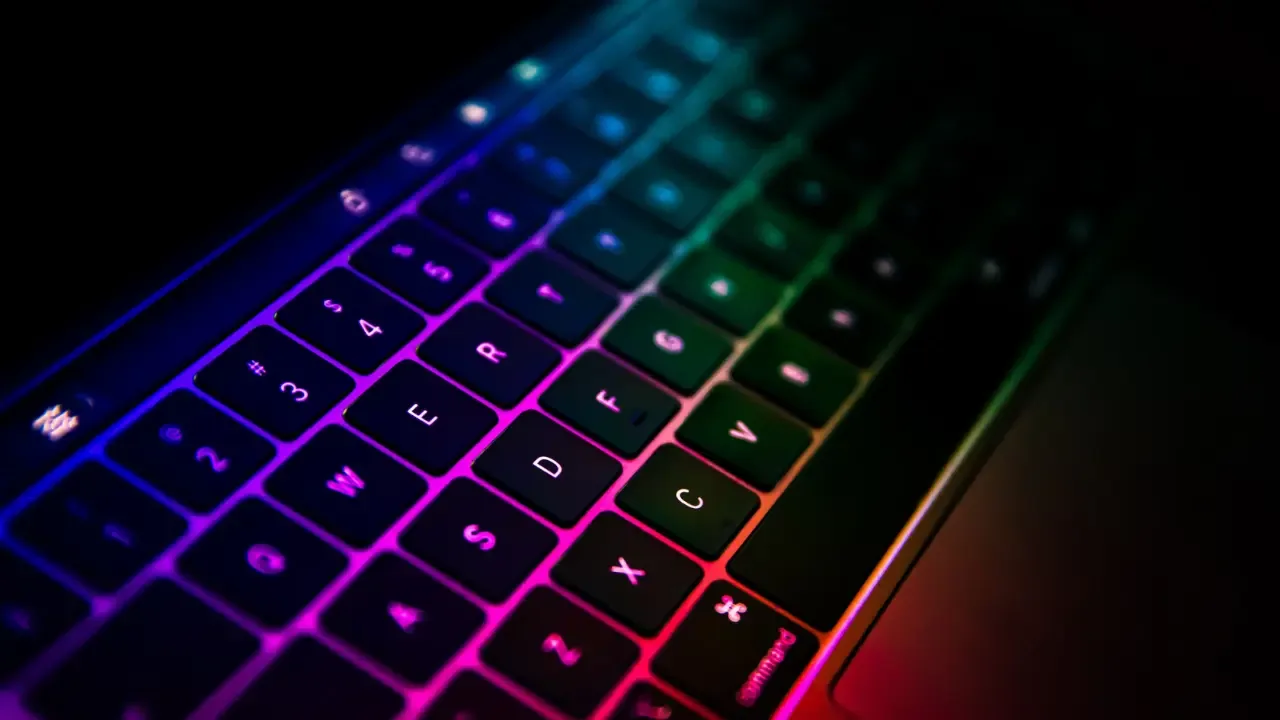
📝 Tech Blog: Easy Guide on Using the Open With Statement to Open Files
Hey there tech enthusiasts! 👋 In today's blog post, we're going to dive into the world of file input and output in Python, specifically focusing on how to open a file using the open with
statement. 📂✨
The Problem: Opening Files with the Open With Statement
Recently, a fellow coder reached out to us with a question. They had a Python code that read a list of names from a file, checked each name against the names in the file, and then appended some text to the occurrences before writing the updated content to another file. The code was functional, but they wondered if there was a better way to achieve the same result. 🤔
Here's a snippet of their code:
def filter(txt, oldfile, newfile):
# Function implementation
# Prompting for user input
text = input('Please enter the name of a great person: ')
letsgo = filter(text, 'Spanish', 'Spanish2')
The challenge they faced was using the with open(...)
statement for both input and output files within the same block. They were concerned that using separate blocks would require storing the names in a temporary location. They wanted to explore a more efficient solution with the open with
statement.
The Solution: Combining Input and Output File Operations
Don't worry, our tech-savvy friend! There is indeed a way to use the open with
statement for both input and output files in the same block. Let's take a look at an updated version of their code:
def filter(txt, oldfile, newfile):
'''\
Read a list of names from a file line by line into an output file.
If a line begins with a particular name, insert a string of text
after the name before appending the line to the output file.
'''
with open(newfile, 'w') as outfile, open(oldfile, 'r', encoding='utf-8') as infile:
for line in infile:
if line.startswith(txt):
line = line[0:len(txt)] + ' - Truly a great person!\n'
outfile.write(line)
# No need to explicitly close the files with the "with" statement.
return # You're good to go without this line!
# Prompting for user input
text = input('Please enter the name of a great person: ')
letsgo = filter(text, 'Spanish', 'Spanish2')
By using the with open(...)
statement for both the input and output files, we can elegantly combine the file operations within the same block. This eliminates the need for temporary storage and makes the code more concise and readable. 🌟
Bonus Tips: Working with with open
Statement
Here are a few additional tips to keep in mind when using the with open
statement:
The
with
statement automatically takes care of closing the files, so no need for explicitclose()
calls.The
with
statement guarantees that the file resources will be released properly, even if an exception is raised during file operations.Remember to specify the appropriate file mode when opening the file (
'r'
for reading,'w'
for writing,'a'
for appending, etc.).
Now that you're armed with this knowledge, go forth and conquer your file input and output tasks with confidence! 💪
Share Your Experiences and Stay Connected!
We hope this guide helped you better understand opening files using the open with
statement and gave you a more optimized solution for your Python code. 🚀
If you have any questions, insights, or other cool file handling tips, feel free to share them in the comments below. Let's geek out together! 🤓💬
Happy coding! 🔥👩💻👨💻
🔐 Don't forget to follow us on Twitter for more tech insights and tutorials!]
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
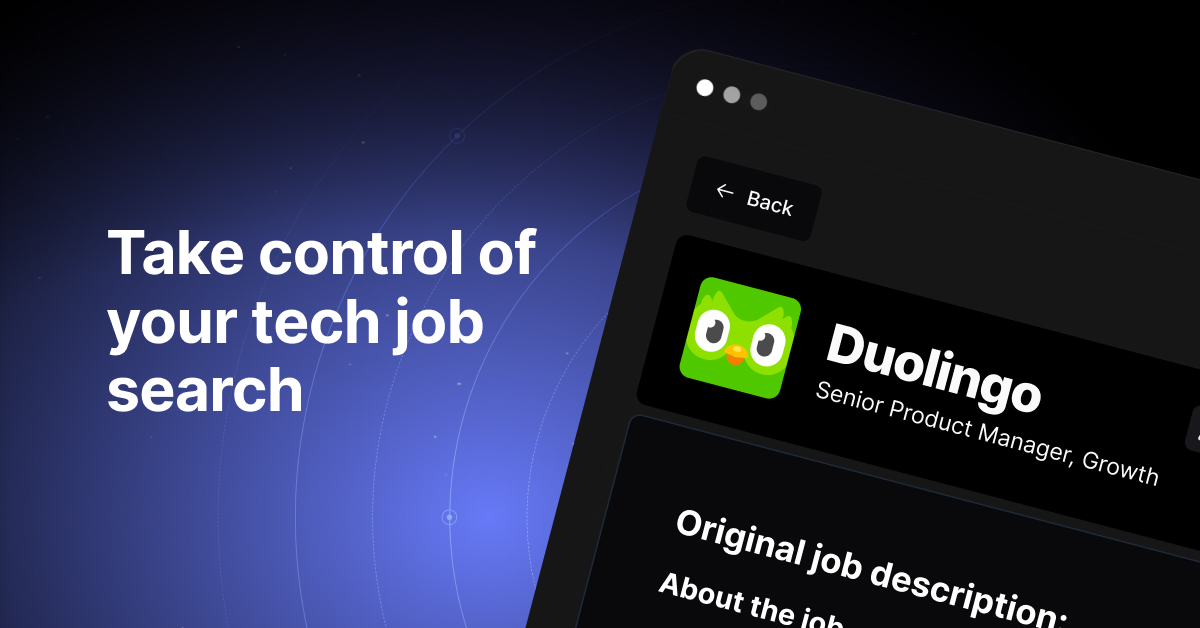