How to make an immutable object in Python?
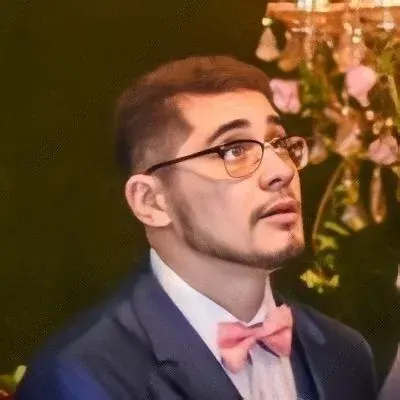
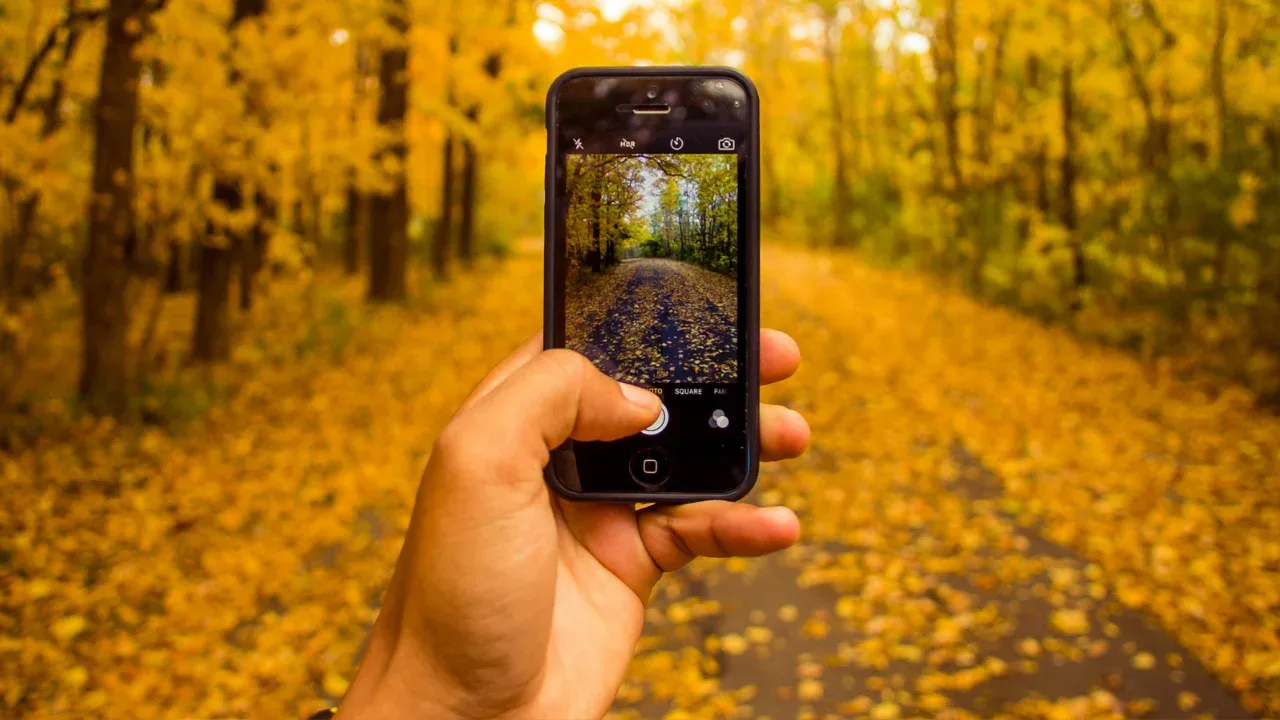
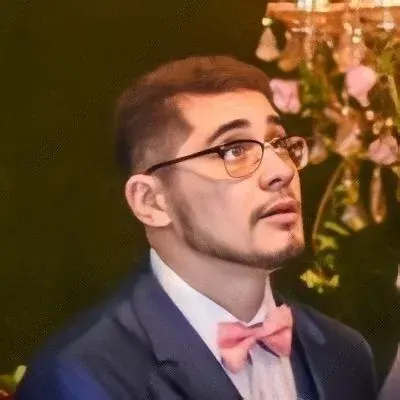
How to Make an Immutable Object in Python: A Complete Guide 🐍
"Immutability is not just a feature, it's a lifestyle" 💪
Are you tired of accidentally modifying your objects in Python? Do you want to ensure that your data remains untouched and unchanged? If so, you've come to the right place! In this blog post, we will explore the concept of immutability in Python and provide you with easy solutions to make your objects immutable. Let's get started! 🚀
Understanding Immutability 🧐
Before we dive into the solutions, let's clarify what immutability means. An immutable object is an object that cannot be modified after it is created. When an object is immutable, its state remains constant throughout its lifetime. This characteristic can be useful in many scenarios, such as ensuring data integrity or for thread safety.
The Challenges of Making an Object Immutable in Python 🤔
Python provides a simple way to define immutable objects through its built-in types like tuples and frozensets. However, creating custom immutable objects requires some additional effort. You might have encountered challenges like:
Inability to set attributes: The
__setattr__
method prevents setting attributes in both the constructor and later stages.Accessing attributes through indexing: Subclassing a tuple or using other workarounds often results in accessing attributes using indexes, which can be cumbersome.
Now that we understand the challenges, let's explore some solutions! 💡
Solution 1: Subclassing a Tuple 📚
Subclassing a tuple is a clever trick that allows us to create immutable objects in Python. By leveraging the __new__
method, we can control the object's creation, and by defining properties for each attribute, we can ensure read-only access. Here's an example code snippet:
class Immutable(tuple):
def __new__(cls, a, b):
return tuple.__new__(cls, (a, b))
@property
def a(self):
return self[0]
@property
def b(self):
return self[1]
def __str__(self):
return "<Immutable {0}, {1}>".format(self.a, self.b)
def __setattr__(self, *ignored):
raise NotImplementedError
def __delattr__(self, *ignored):
raise NotImplementedError
In this example, we define a class Immutable
that subclasses tuple
. We override the __new__
method to ensure that the object is created correctly with the desired attributes. Properties are defined for each attribute (a
and b
), providing read-only access to the values. The __setattr__
and __delattr__
methods are implemented to raise an exception if any attempt is made to modify or delete attributes.
Solution 2: Using C Extensions 📦
If your needs go beyond what is possible in pure Python, you can create immutable objects using C extensions. This approach requires more advanced knowledge of Python's C API and might not be suitable for every use case. However, if performance is crucial and a more fine-grained control is needed, this solution can be a great option.
The Future is Now 🔮
Starting from Python 3.7, there is even a more straightforward solution available - the @dataclass
decorator. This decorator, introduced in the dataclasses
module, automatically generates boilerplate code for creating immutable objects with minimal effort. To learn more about this approach, check out the official documentation for the @dataclass
decorator.
Conclusion and Your Next Move 🏁
Congratulations! You now have a solid understanding of how to create immutable objects in Python. 😎 Whether you choose to subclass a tuple or explore C extensions, immutability can help you enforce data integrity and maintain control over your objects.
Now it's your turn! Give it a try and experiment with creating your immutable objects. Share your experiences and any other creative techniques you discover!
Have you ever worked with immutable objects in Python? What challenges have you faced, and how did you overcome them? Share your thoughts and ideas in the comments below. Let's have a discussion! 👇
Happy Pythoning! 🐍✨