How to make a class JSON serializable
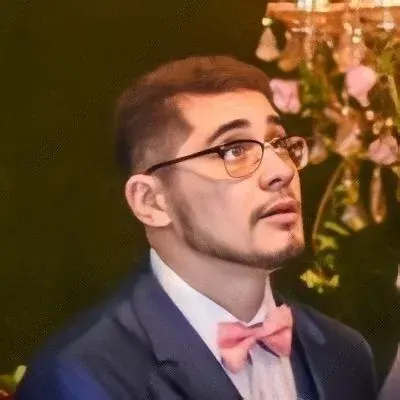
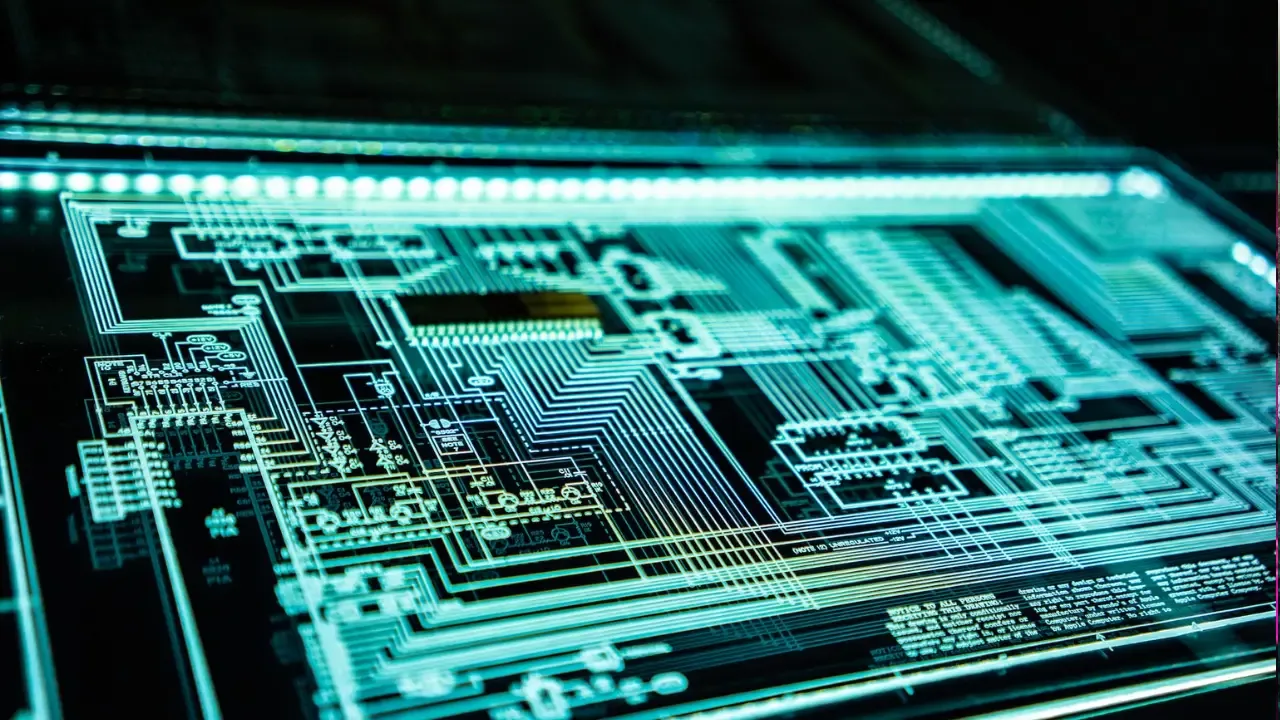
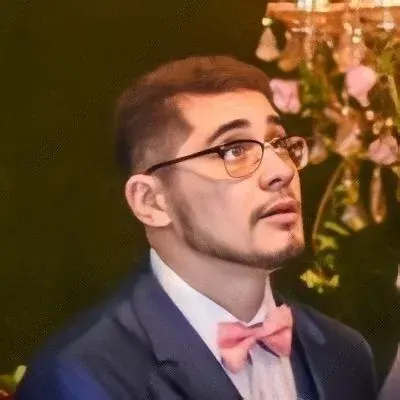
Making a Class JSON Serializable 🌟
So, you've encountered the dreaded TypeError: Object of type 'FileItem' is not JSON serializable
when trying to serialize a Python class to JSON. Don't worry, we've all been there! 🤷♀️
Serializing a class to JSON means converting its object into a JSON string, which is useful for transferring data between different systems or storing it in a file. However, not all Python classes are JSON serializable by default, as they may contain non-serializable attributes or complex data types.
But fear not, my fellow Pythonistas! In this guide, I will show you some easy solutions to make your class JSON serializable.
The Problem 🧐
Let's start with an example class called FileItem
:
class FileItem:
def __init__(self, fname):
self.fname = fname
Now, imagine you have an instance of this class and you want to serialize it to JSON using the json.dumps()
function:
import json
x = FileItem('/foo/bar')
json.dumps(x)
At this point, you will be met with the frustrating TypeError: Object of type 'FileItem' is not JSON serializable
. 😞
The reason for this error is that the json
library doesn't know how to handle custom classes by default. Luckily, there are a few ways to overcome this obstacle and make our class JSON serializable.
Solution 1: Implementing the __dict__
Method 📝
One simple way to make a class JSON serializable is to define the __dict__
method. This method returns a dictionary representation of the class instance, which can be easily serialized to JSON.
Here's how to implement this method in our FileItem
class:
class FileItem:
def __init__(self, fname):
self.fname = fname
def __dict__(self):
return self.__dict__
Now, if we try to serialize an instance of FileItem
to JSON, it should work like a charm:
x = FileItem('/foo/bar')
json.dumps(x)
No more TypeError
! 🎉
Solution 2: Implementing the json.JSONEncoder
Class 🎚
Another approach is to create a custom JSON encoder by inheriting from the json.JSONEncoder
class. This allows us to define how our class should be serialized to JSON.
Here's an example:
import json
class FileItemEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, FileItem):
return obj.__dict__
return super().default(obj)
x = FileItem('/foo/bar')
json.dumps(x, cls=FileItemEncoder)
By overriding the default()
method of json.JSONEncoder
, we check if the object is an instance of FileItem
. If it is, we return its __dict__
, which is then serializable to JSON.
Solution 3: Using the dataclasses
Module 💡
Starting from Python 3.7, we have the dataclasses
module, which provides a decorator to automatically generate special methods for classes. One of these methods is the asdict()
method, which returns a dictionary representation of the class.
To make our FileItem
class serializable, we can leverage the dataclasses
module:
from dataclasses import dataclass, asdict
@dataclass
class FileItem:
fname: str
x = FileItem('/foo/bar')
json.dumps(asdict(x))
The dataclass
decorator adds the necessary methods behind the scenes, including the asdict()
method, which we can use to convert our instance to a dictionary and then serialize it to JSON.
Conclusion and Call-to-Action 🎉
Congratulations! You have learned how to make a Python class JSON serializable. We covered three different solutions:
Implementing the
__dict__
method: Ideal when you want a simple and quick solution.Implementing the
json.JSONEncoder
class: Useful when you want more control over the serialization process.Using the
dataclasses
module: Great for automatically generating serialization methods.
Now it's time for you to put this knowledge into practice! Try serializing your own custom classes and see the magic happen. ✨
If you found this guide helpful, share it with your fellow Python enthusiasts and let them also become JSON serialization masters! 🚀
Have any questions or additional tips to share? Let me know in the comments below. Together, we can conquer any serialization challenge! 💪
Happy coding! 😄🐍